Flutter learning resources
An instructor-led version of our very popular 'Write your first Flutter app' codelab.
Create a simple random-name generator app. This app is responsive and runs on mobile, desktop, and web.
Discover Dart 3's new records and patterns features. Learn how you can use them in a Flutter app to help you write more readable and maintainable Dart code.
Start with an app that performs simple, straightforward scrolling and enhance it to create fancy and custom scrolling effects by using slivers.
Learn how to use some of the features in Material 3 to make your app beautiful and responsive.
Learn how to build a Flutter app that uses the power of flutter_animate
, fragment shaders, and particle fields.
Learn how to build a Flutter app that adapts to the platform that it's running on, be that Android, iOS, the web, Windows, macOS, or Linux.
Learn how to build animated effects in Flutter. You'll learn how to build implicit and explicit animations, and customize navigation transition animations the animations package and predictive back on Android.
Learn how to use the Material animations package to add pre-built transitions to a Material app called Reply.
Step-by-step instructions on how to debug common layout problems using the Flutter Inspector and Layout Explorer.
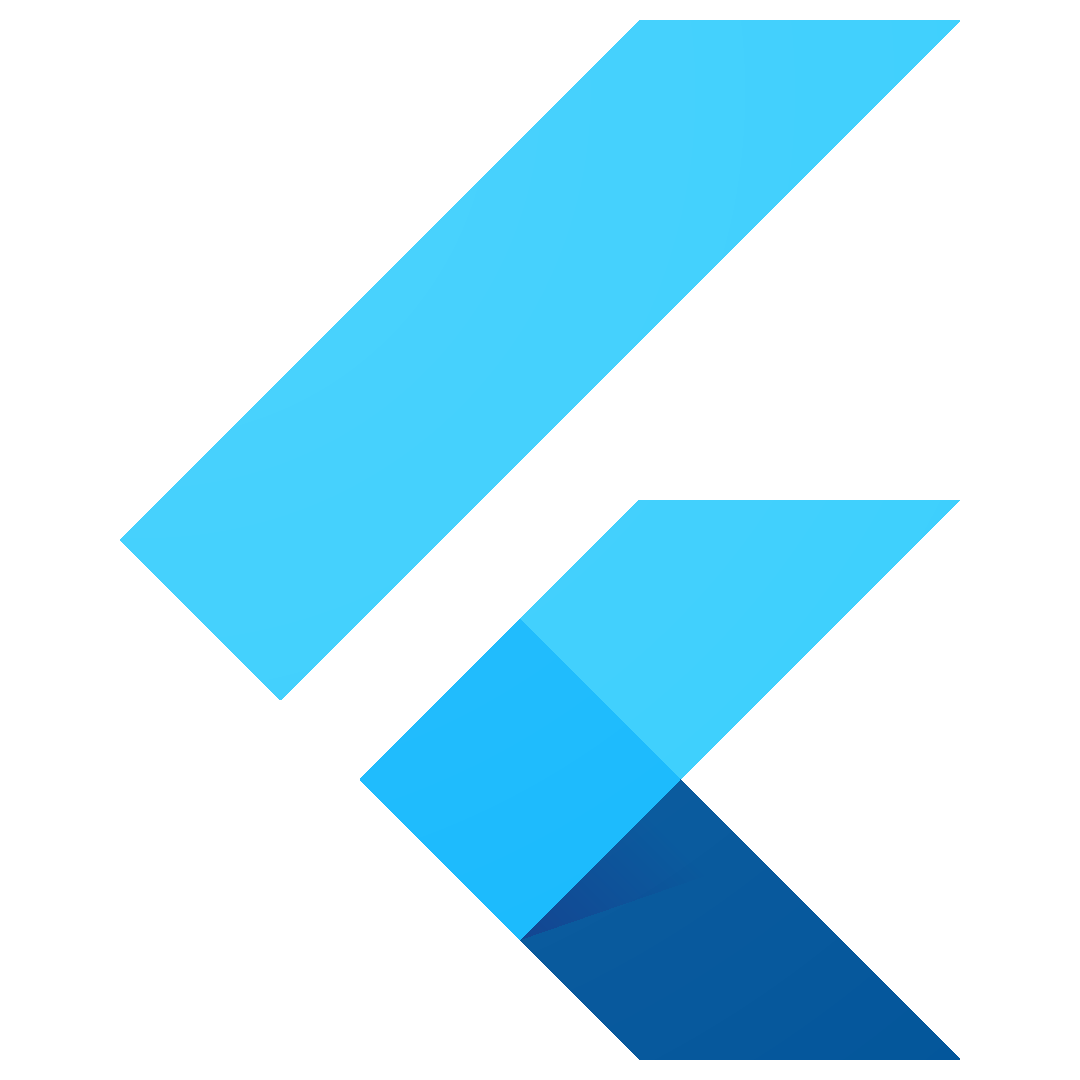
Use DartPad (no downloads required!) to learn how to use implicit animations to add motion and create visual effects for the widgets in your UI.
Learn the basics of using Material Components by building a simple app with core components.
Learn how to use Material for structure and layout in Flutter. Continue building the e-commerce app, introduced in MDC-101, by adding navigation, structure, and data.
Discover how Material Components for Flutter make it easy to differentiate your product, and express your brand through design.
Improve your design and learn to use our advanced component backdrop menu.
Learn how to add an AdMob banner, an interstitial ad, and a rewarded ad to an app called Awesome Drawing Quiz, a game that lets players guess the name of the drawing.
Learn how to implement inline banner and native ads to a travel booking app that lists possible flight destinations.
Extend a simple gaming app that uses the Dash mascot as currency to offer three types of in-app purchases: consumable, non-consumable, and subscription.
Learn how to add Firebase authentication to a Flutter app with only a few lines of code.
Build an event RSVP and guestbook chat app on both Android and iOS using Flutter, authenticating users with Firebase Authentication, and sync data using Cloud Firestore.
Learn how to develop a multi-platform app with Flutter and Firebase Cloud Messaging, integrating FCM to send and receive messages on Android, iOS, and web.
The SoLoud package, a free and portable engine, delivers the low-latency and high-performance sound that's essential for many games.
This codelab guides you through crafting game mechanics in a Flutter and Flame game using a 2D physics simulation called Forge2D.
This codelab focuses on building word puzzle games, and dives into using Flutter's background processing to generate expansive crossword-style grids of interlocking words.
Build a Breakout clone using the Flame 2D game engine and embed it in a Flutter wrapper.
Display a Google map in an app, retrieve data from a web service, and display the data as markers on the map.
With the WebView Flutter plugin you can add a WebView widget to your Android or iOS Flutter app.
Learn how to use Dart's FFI (foreign function interface) library, ffigen, allowing you to leverage existing native libraries that provide a C interface.
Start with a simple app that manages state with the Provider package. Unit test the provider package. Write widget tests for two of the widgets. Use Flutter Driver to create an integration test.
Learn how to add a Home Screen widget to your Flutter app on iOS. This applies to your home screen, lock screen, or the today view.
Build a Flutter desktop app (Windows, Linux, or macOS) that accesses GitHub APIs, and create and use plugins to interact with native APIs and desktop applications.
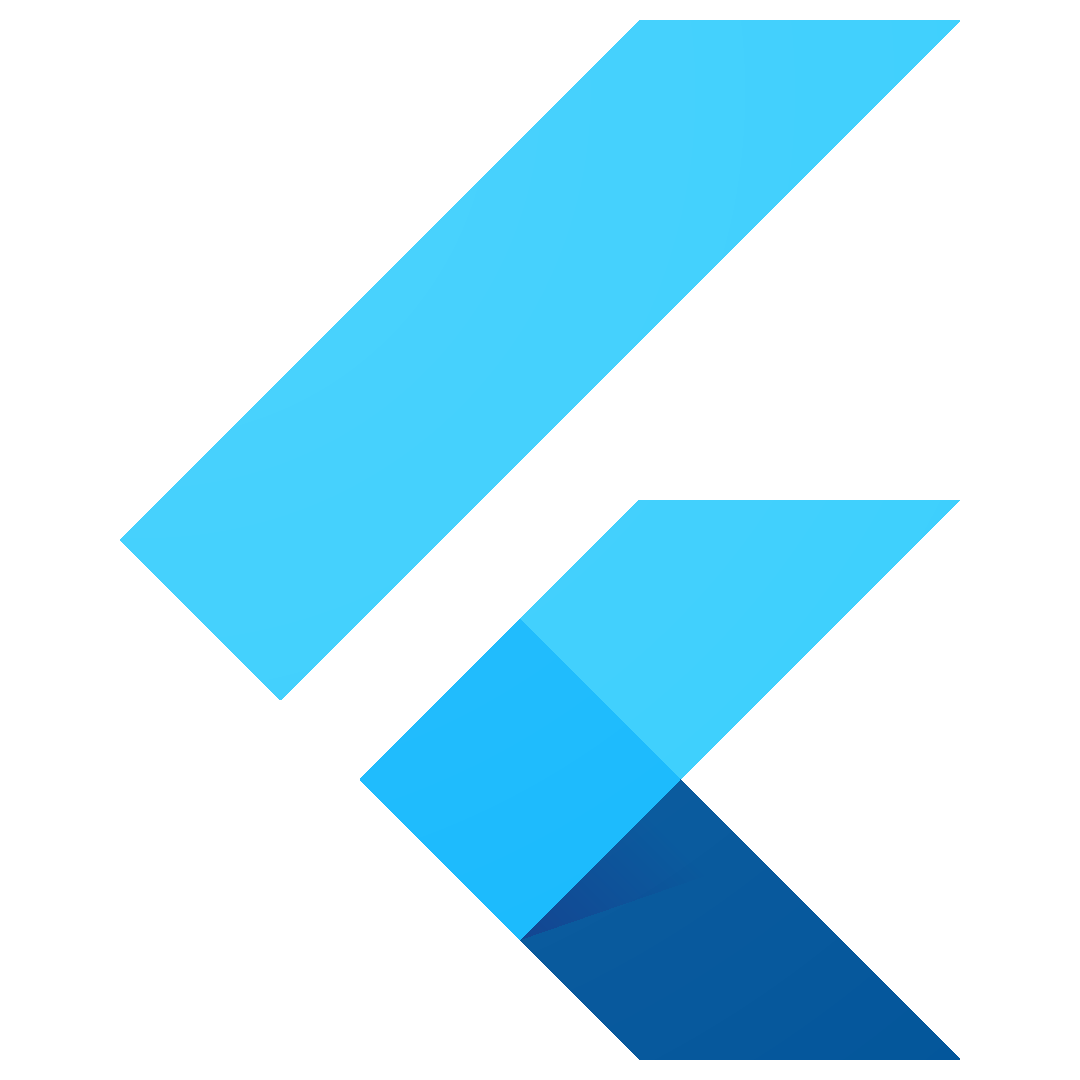
Transition between routes by animating the new route into view from the bottom of the screen.
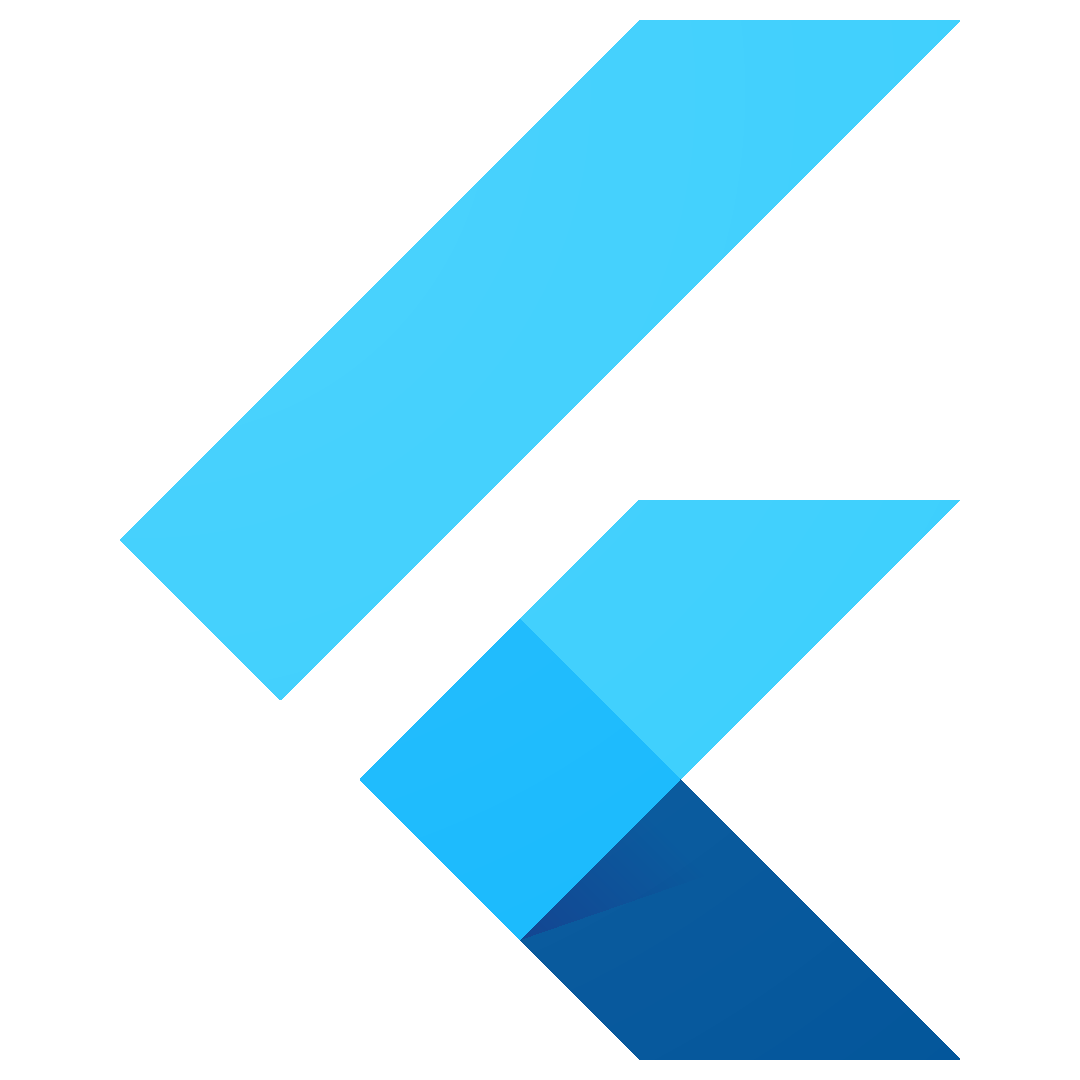
Learn how to move a widget from a dragged point back to the center using a spring simulation.
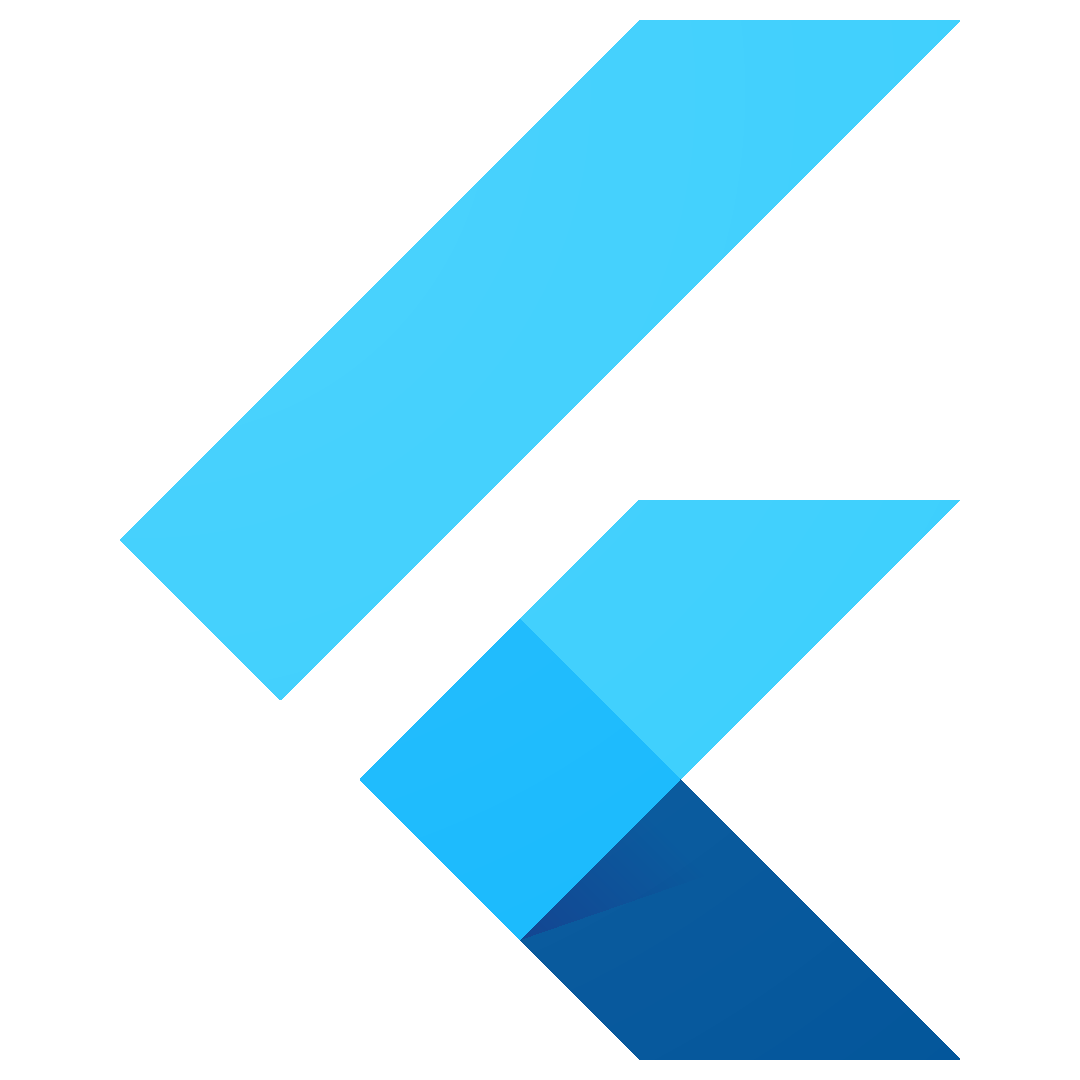
Use AnimatedContainer to animate the size, background color, and border radius of a Container.
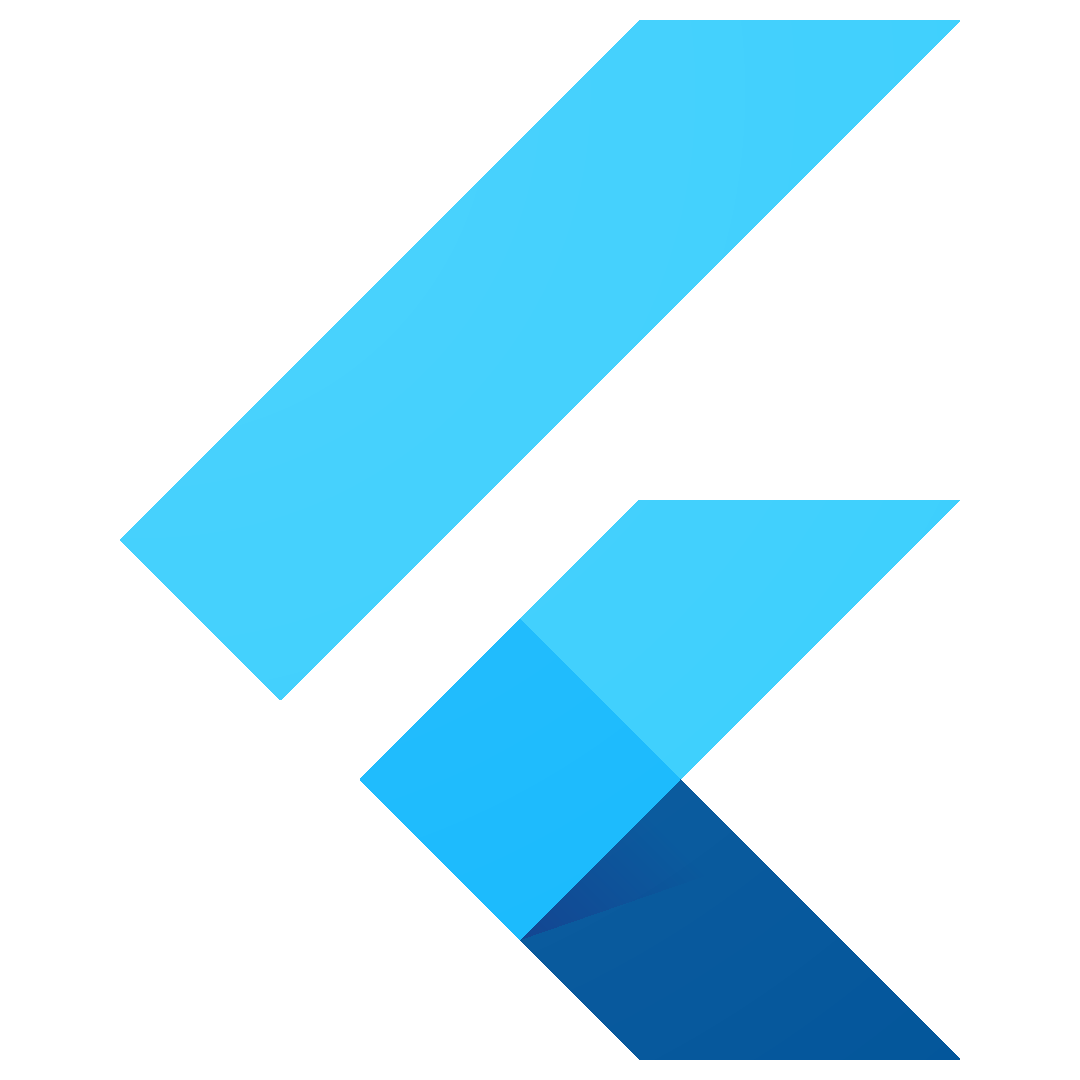
The AnimatedOpacity widget makes it easy to perform opacity animations.
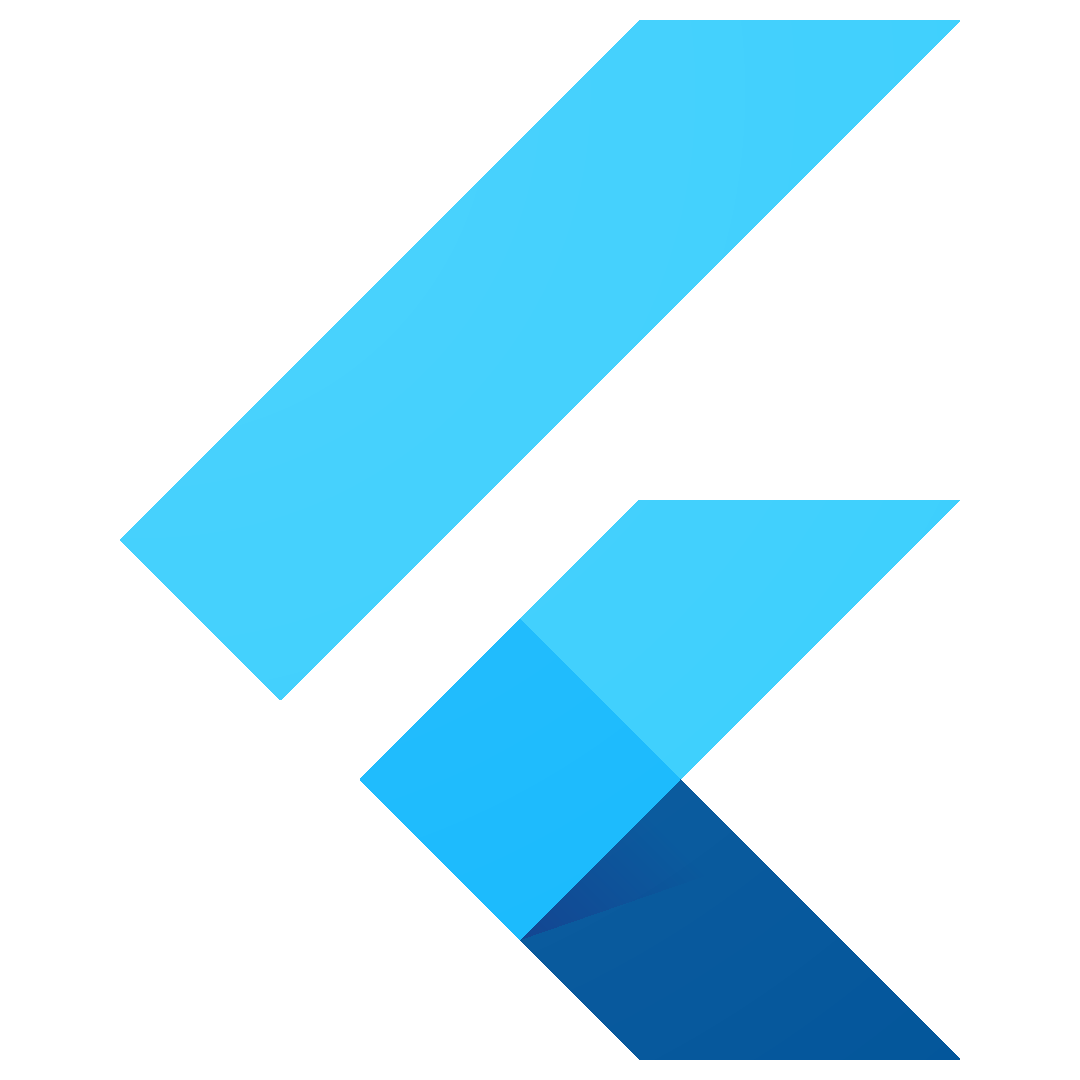
Use the Drawer widget in combination with a Scaffold to create a layout with a Material Design drawer.
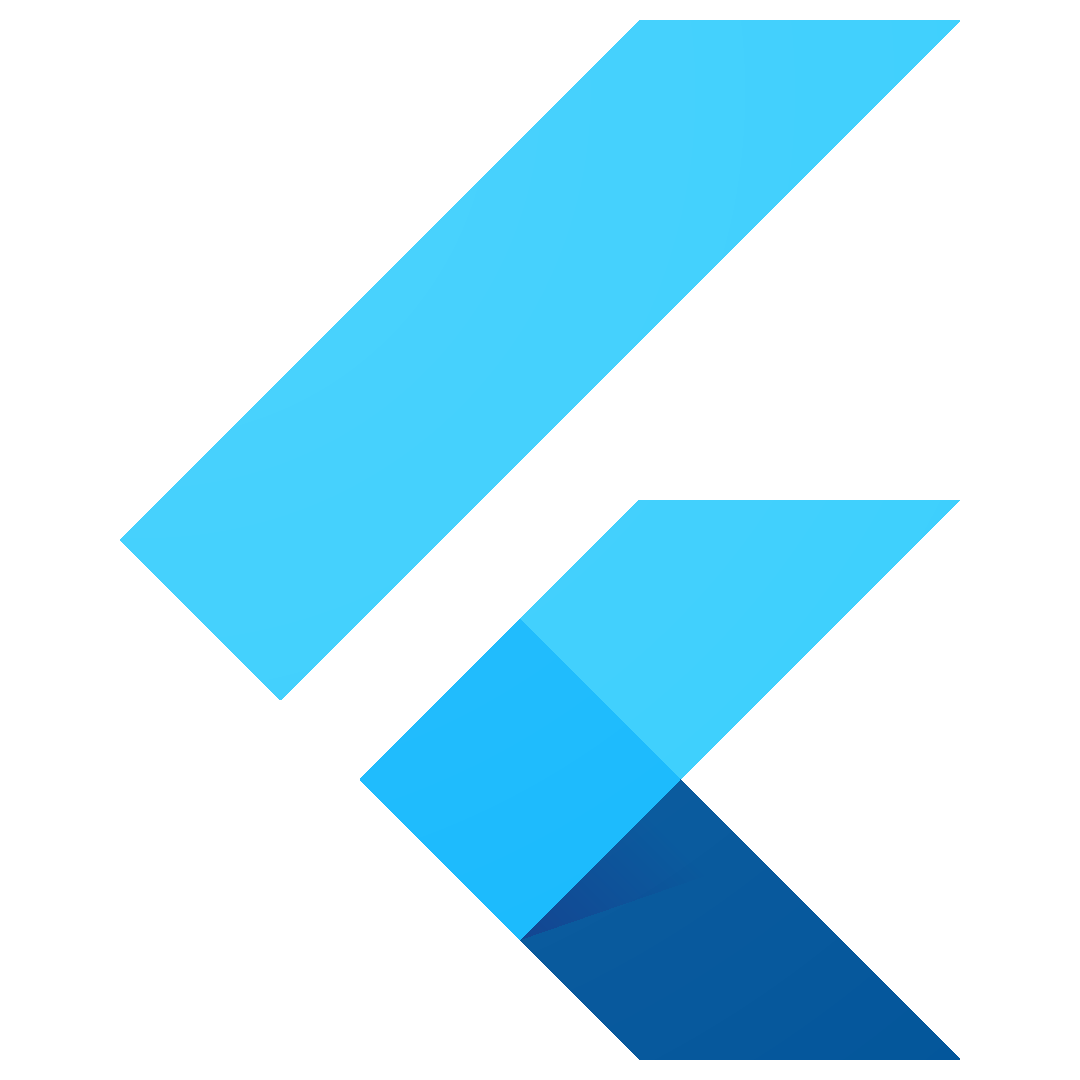
Use the Snackbar widget to display messages to your users.
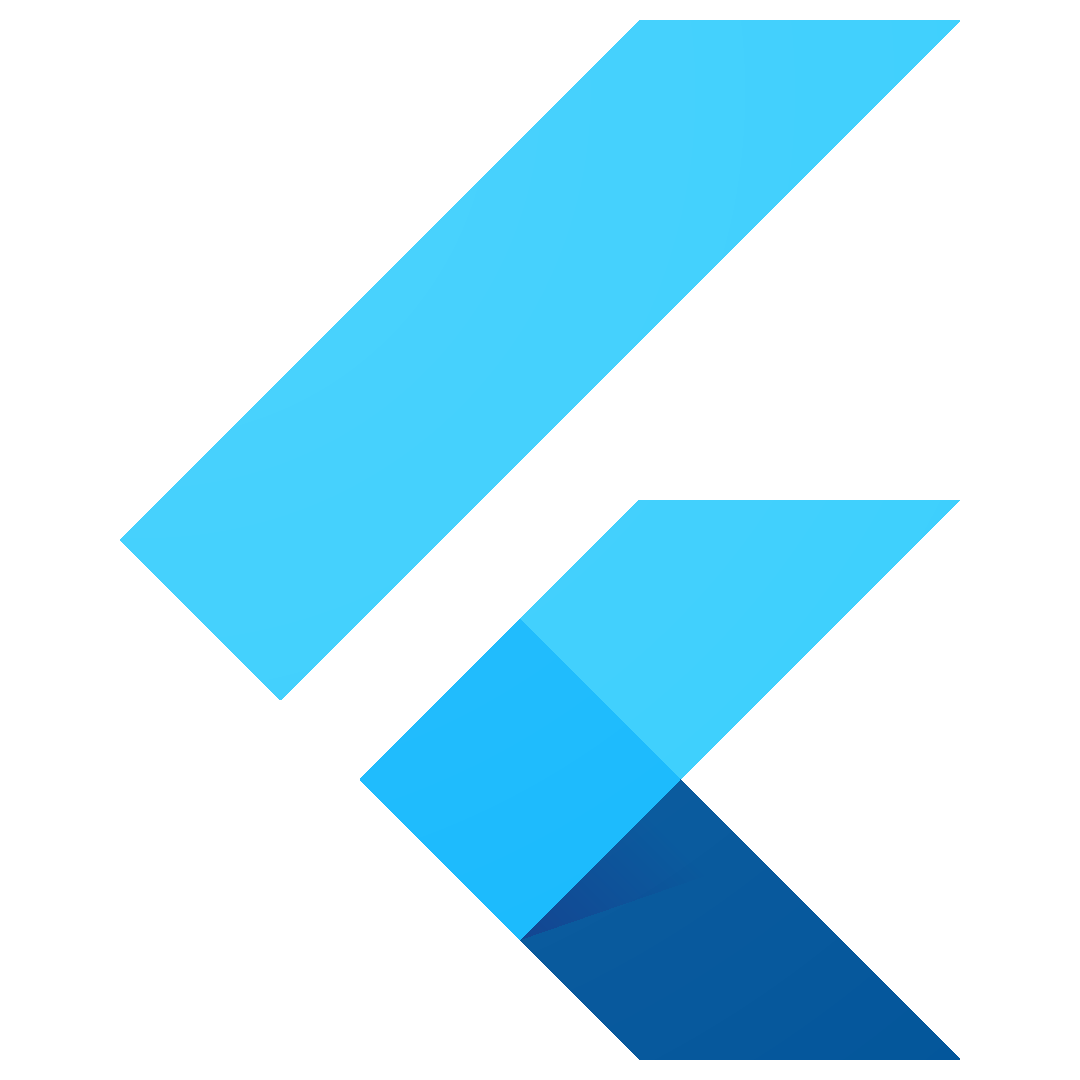
Use a font across multiple apps.
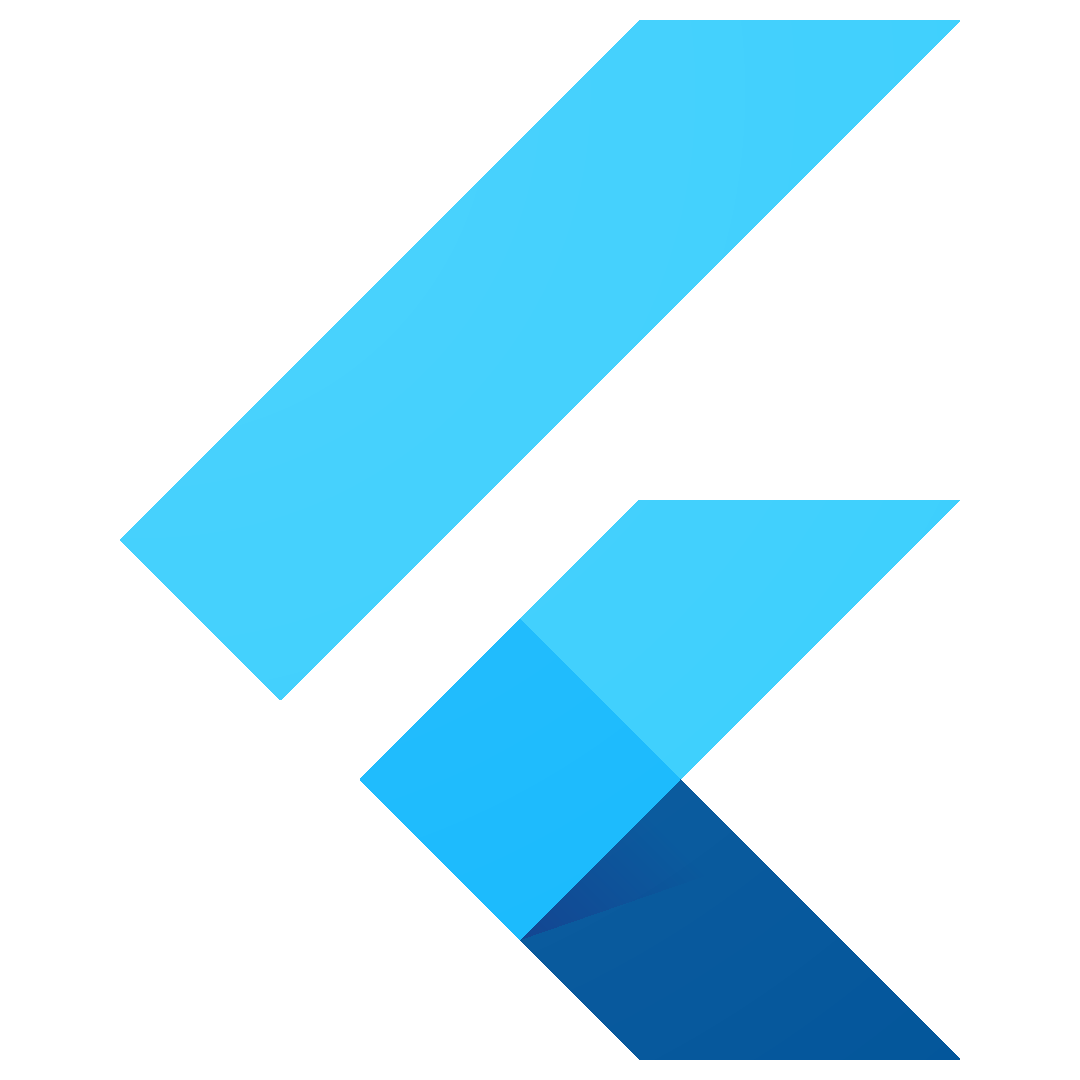
Build a list that displays two columns in portrait mode and three columns in landscape mode.
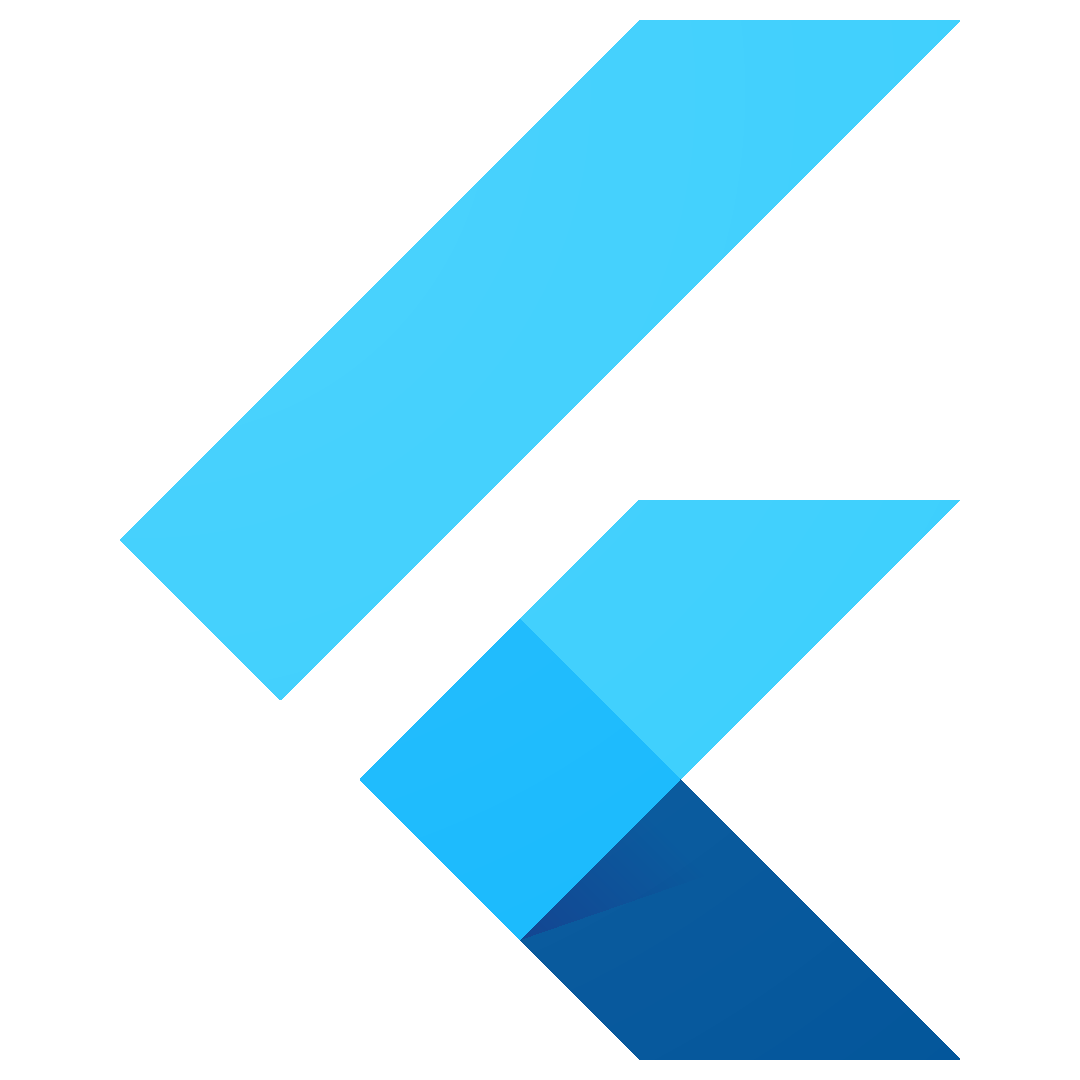
Apply fonts to your entire app or individual widgets.
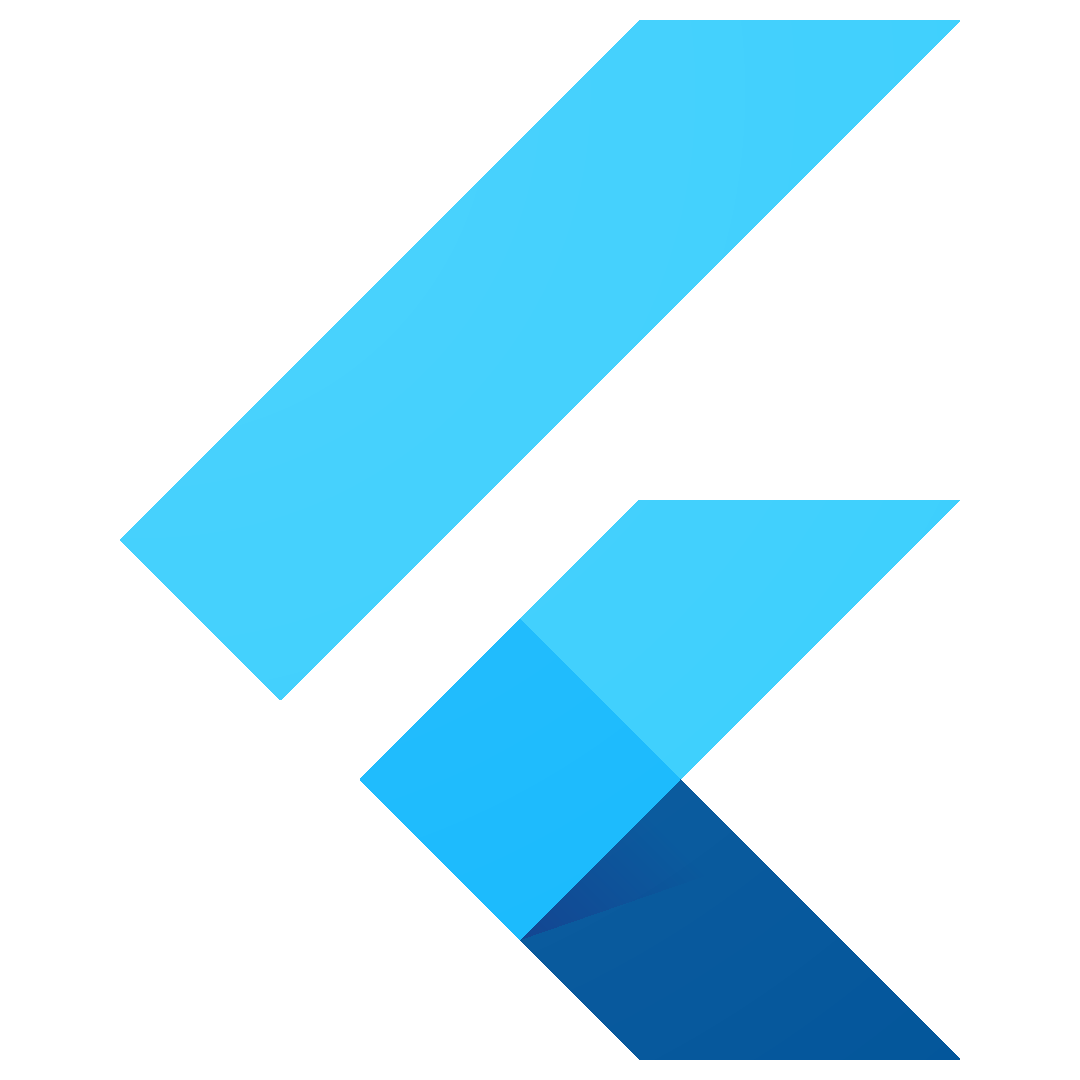
To share styles across your app, use Themes.
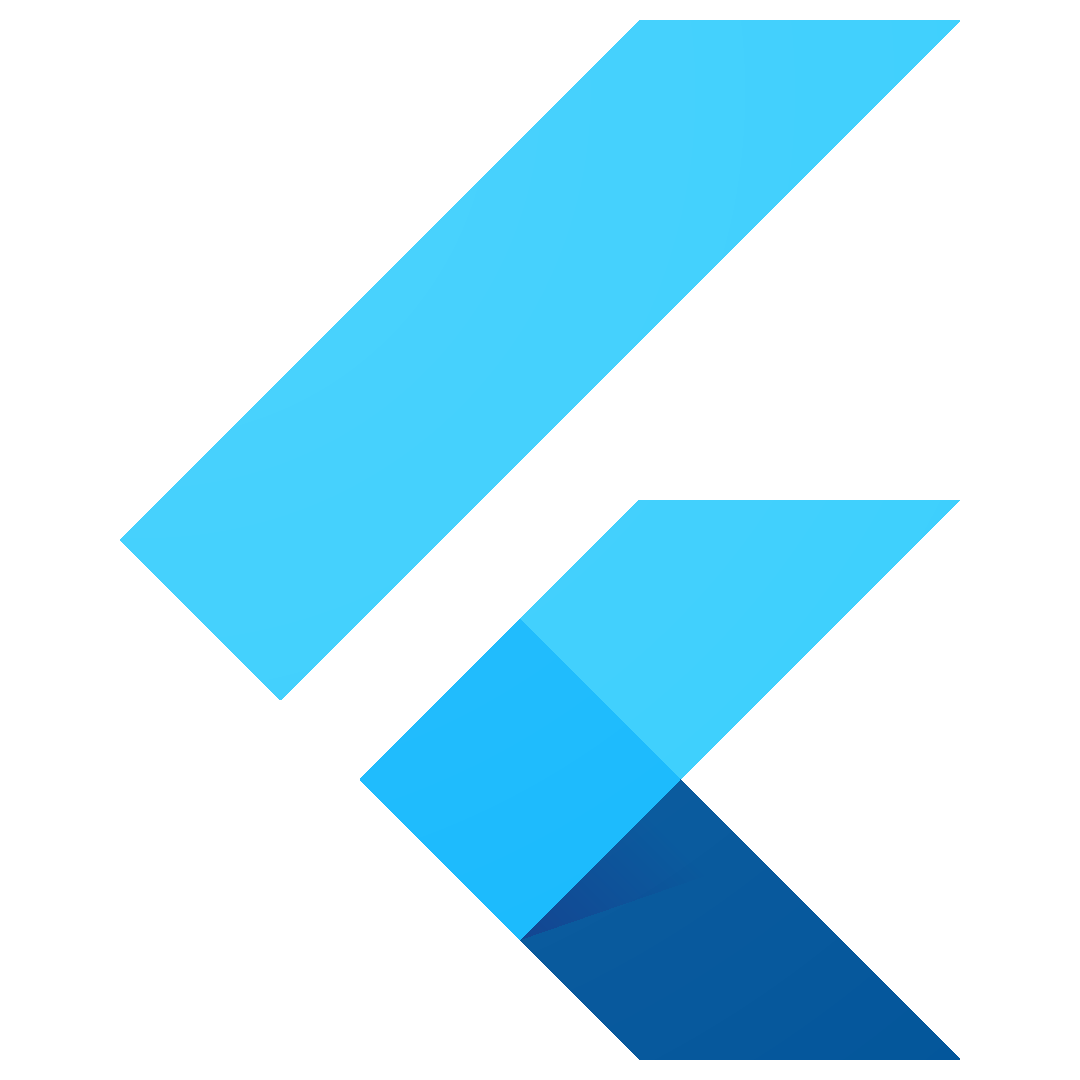
Working with tabs is a common pattern in mobile apps that follow the Material Design or Cupertino guidelines.
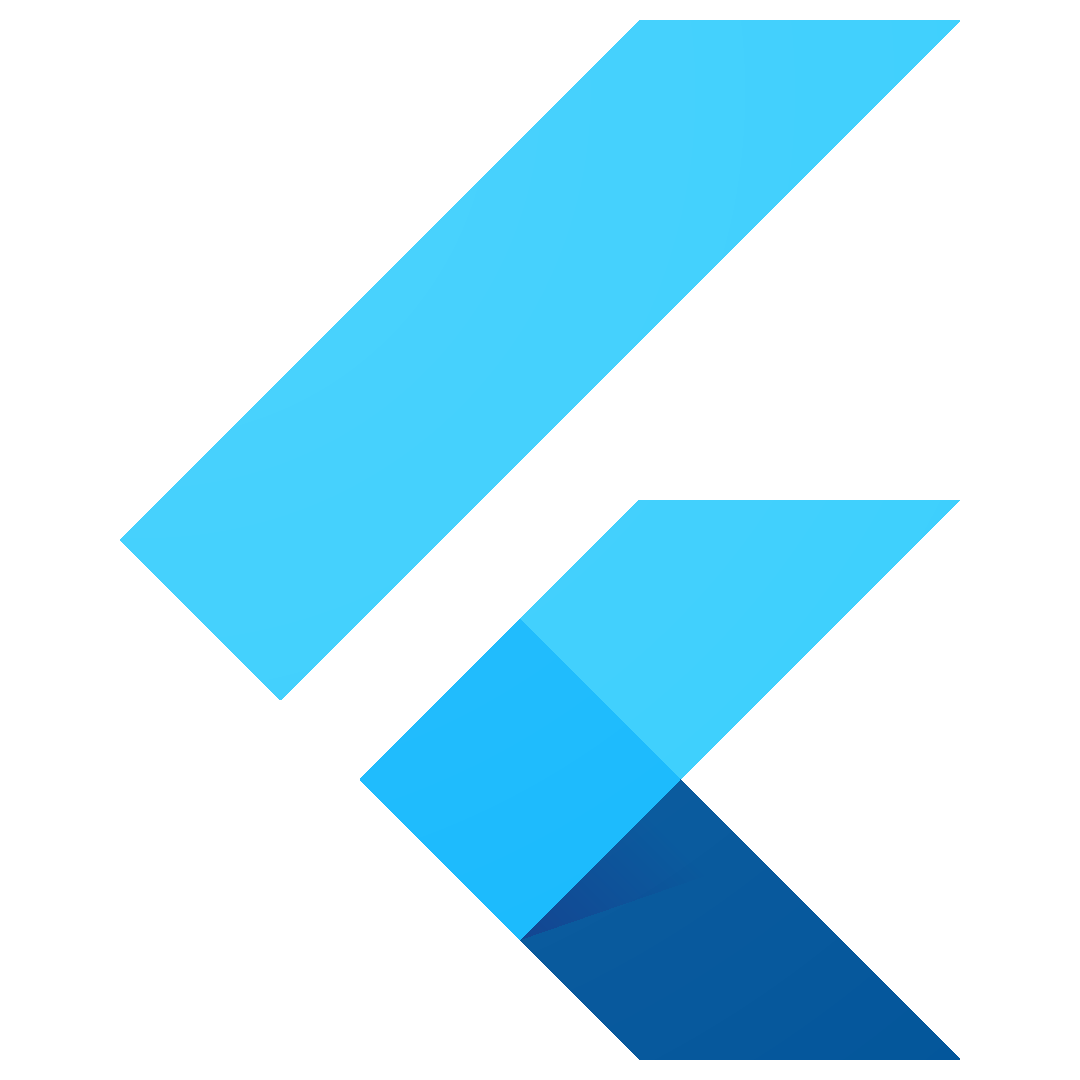
Build a download button that transitions through multiple visual states, based on the status of an app download.
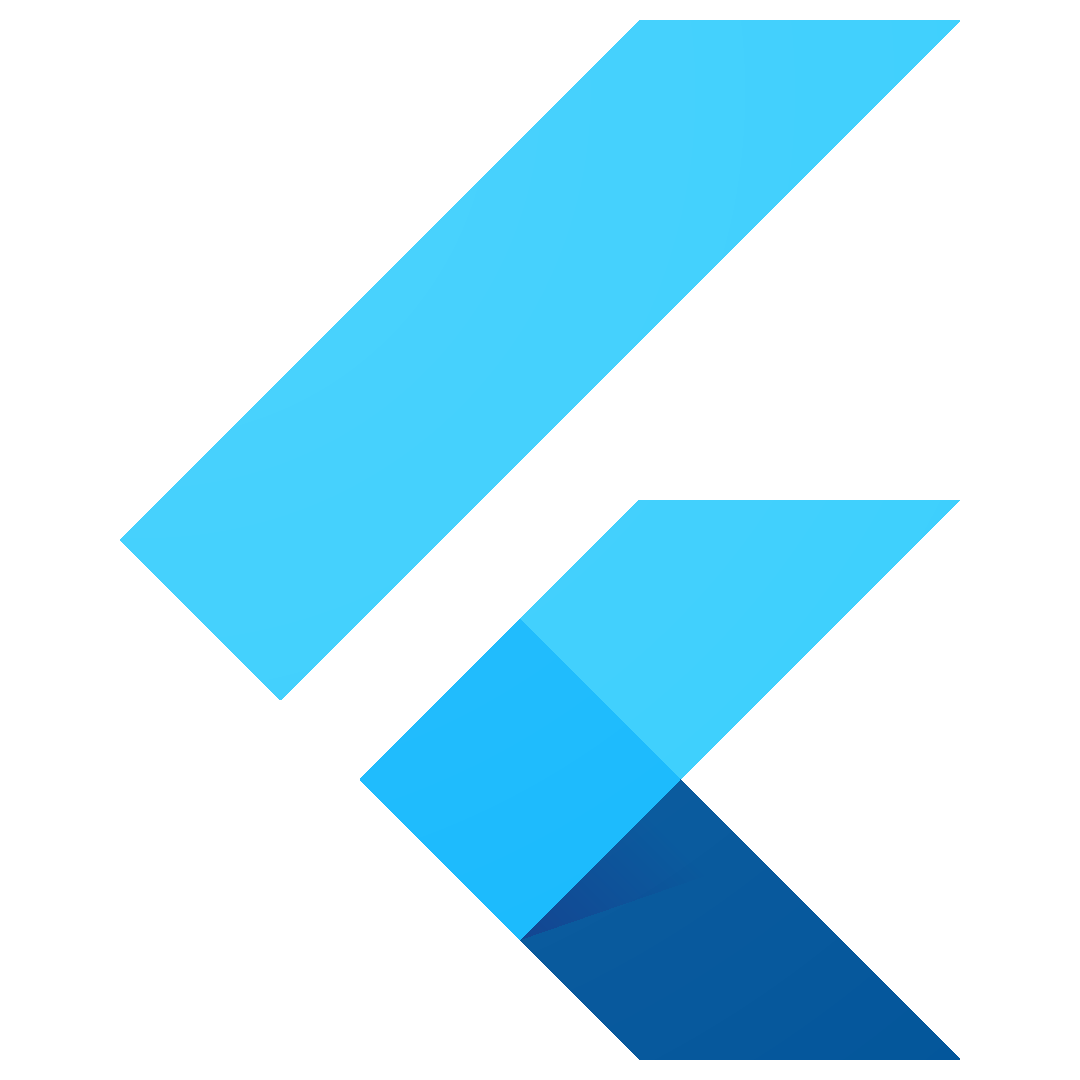
Create top level routes, and routes nested below specific widgets.
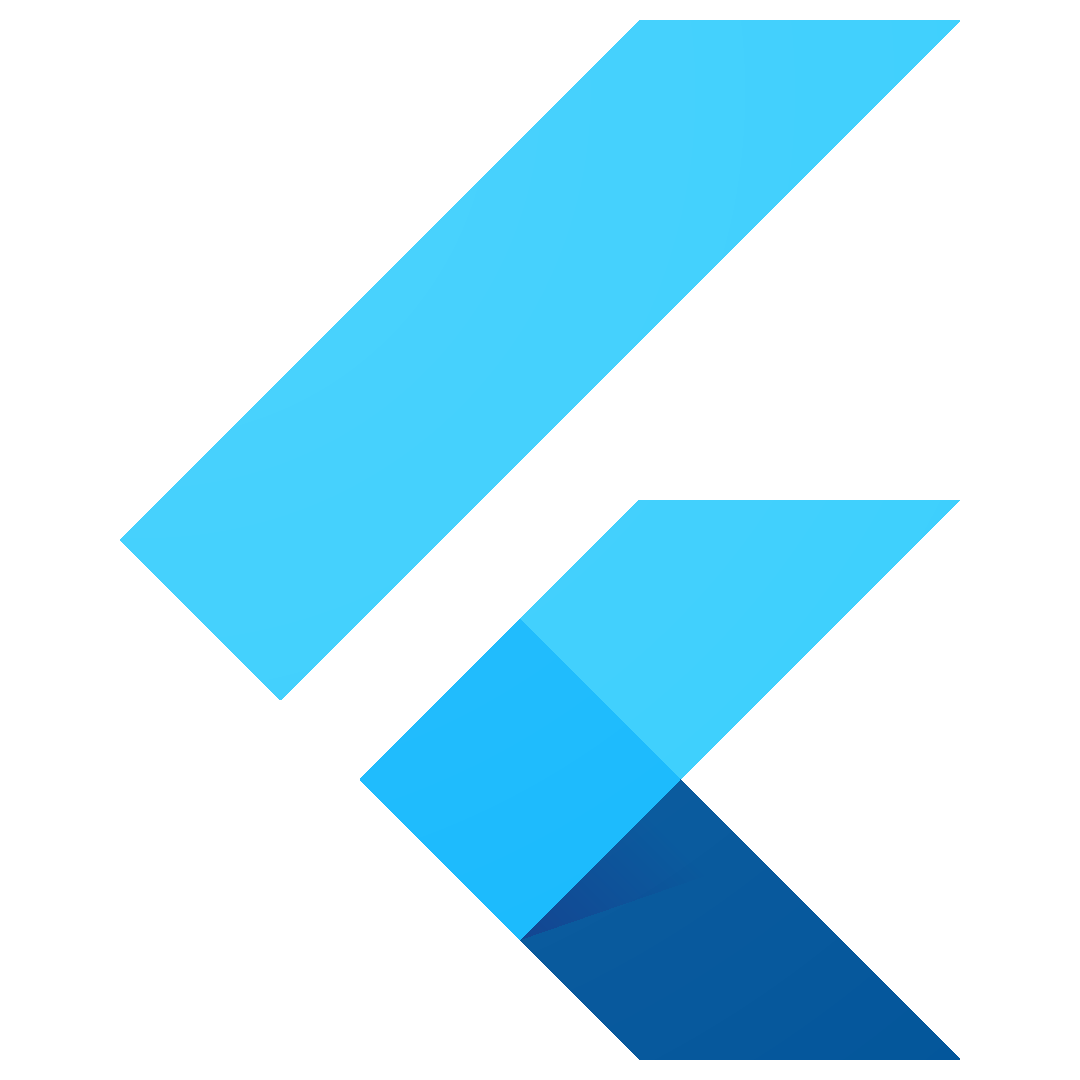
Create the parallax effect by building a list of cards with images that 'move'.
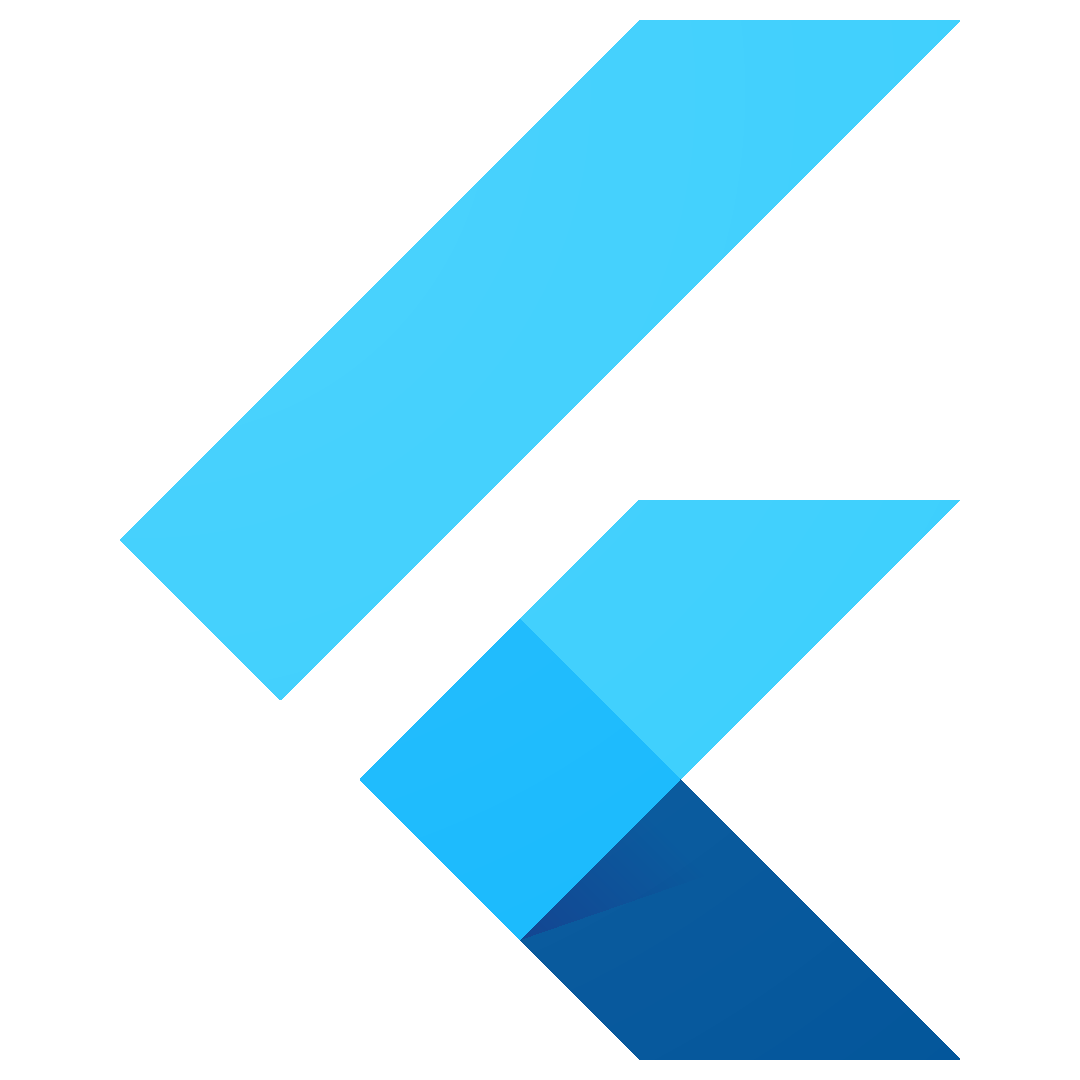
Communicate that data is loading with a chrome color shimmer on the screen.
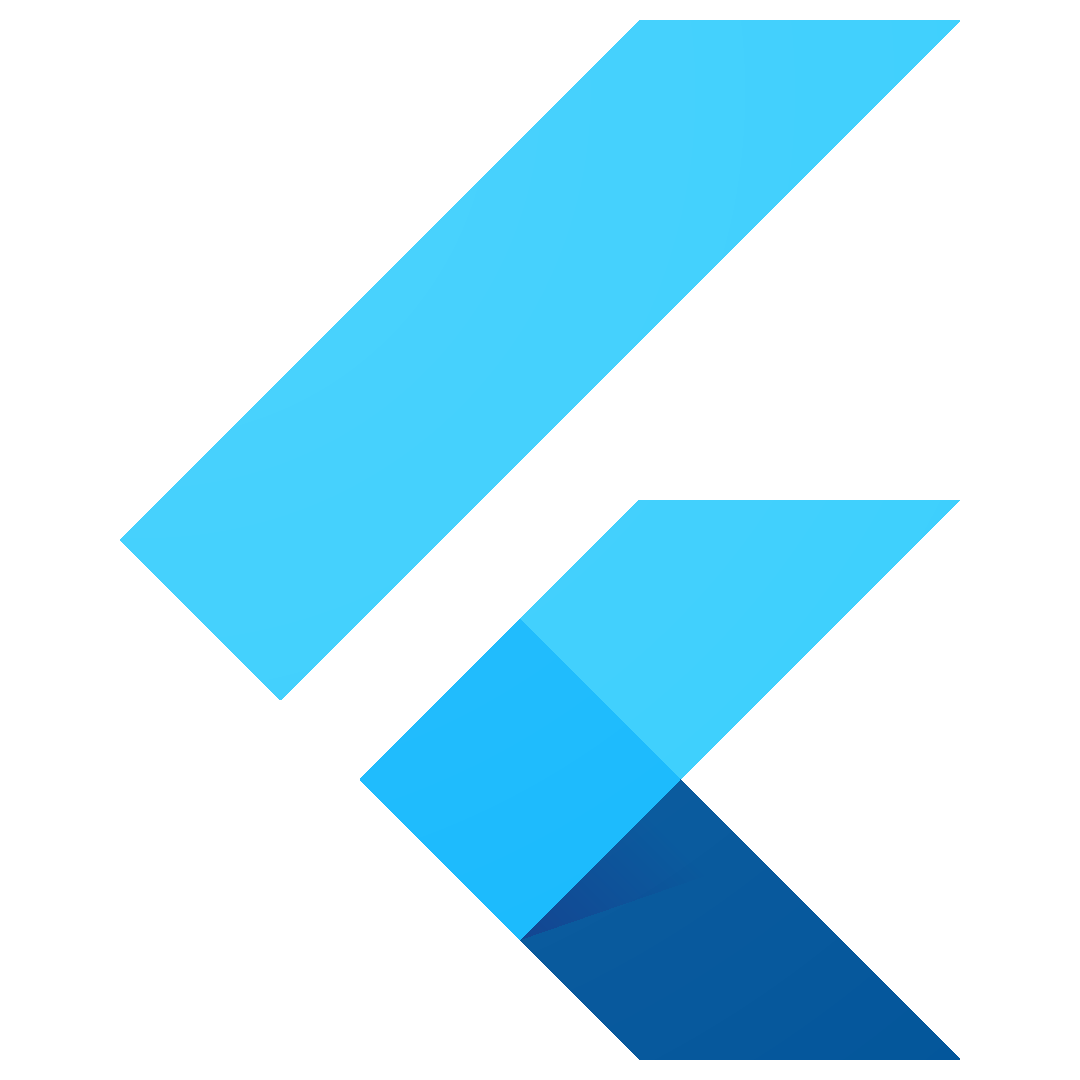
Build a drawer menu with animated content that is staggered and has a button that pops in at the bottom
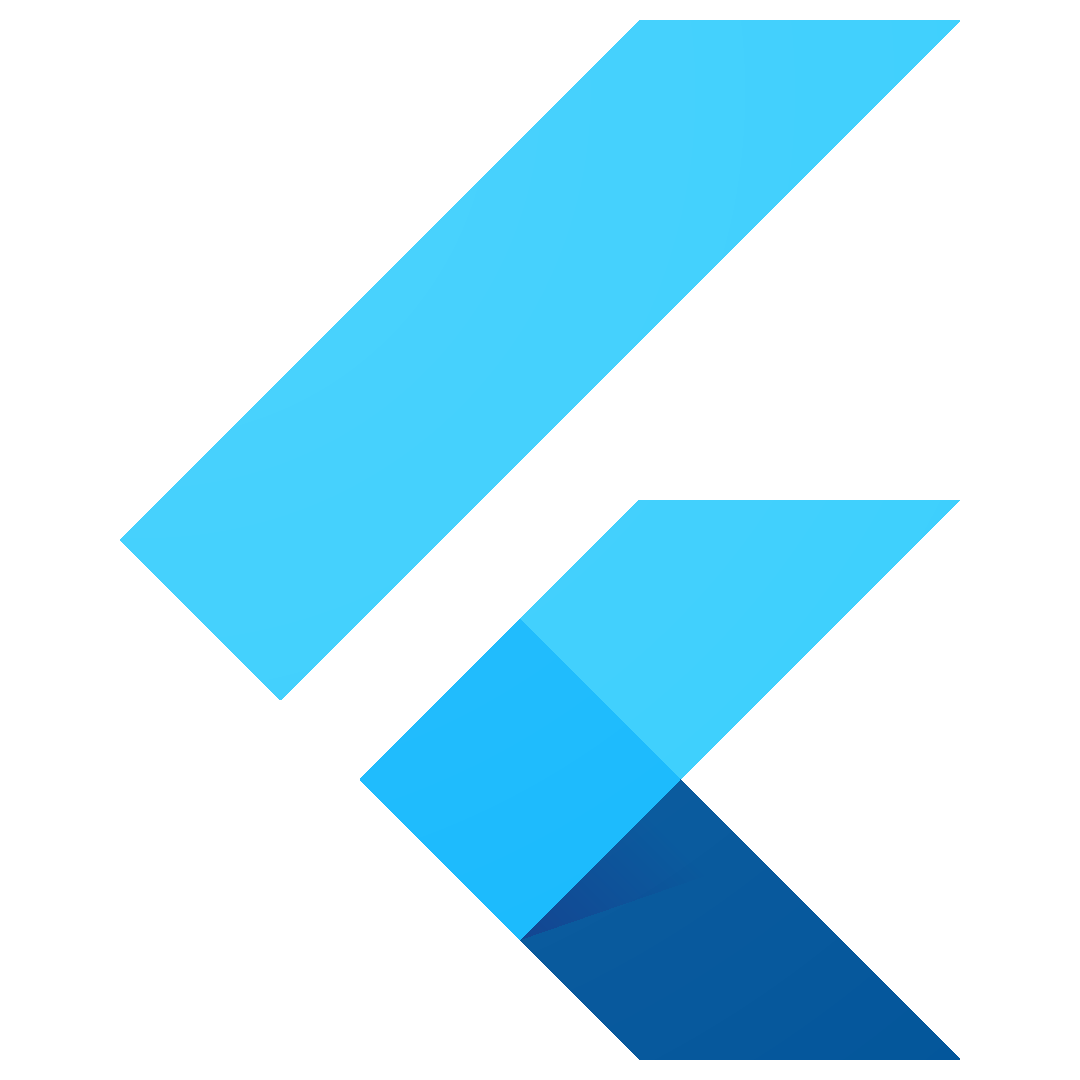
Build a speech bubble typing indicator that animates in and out of view.
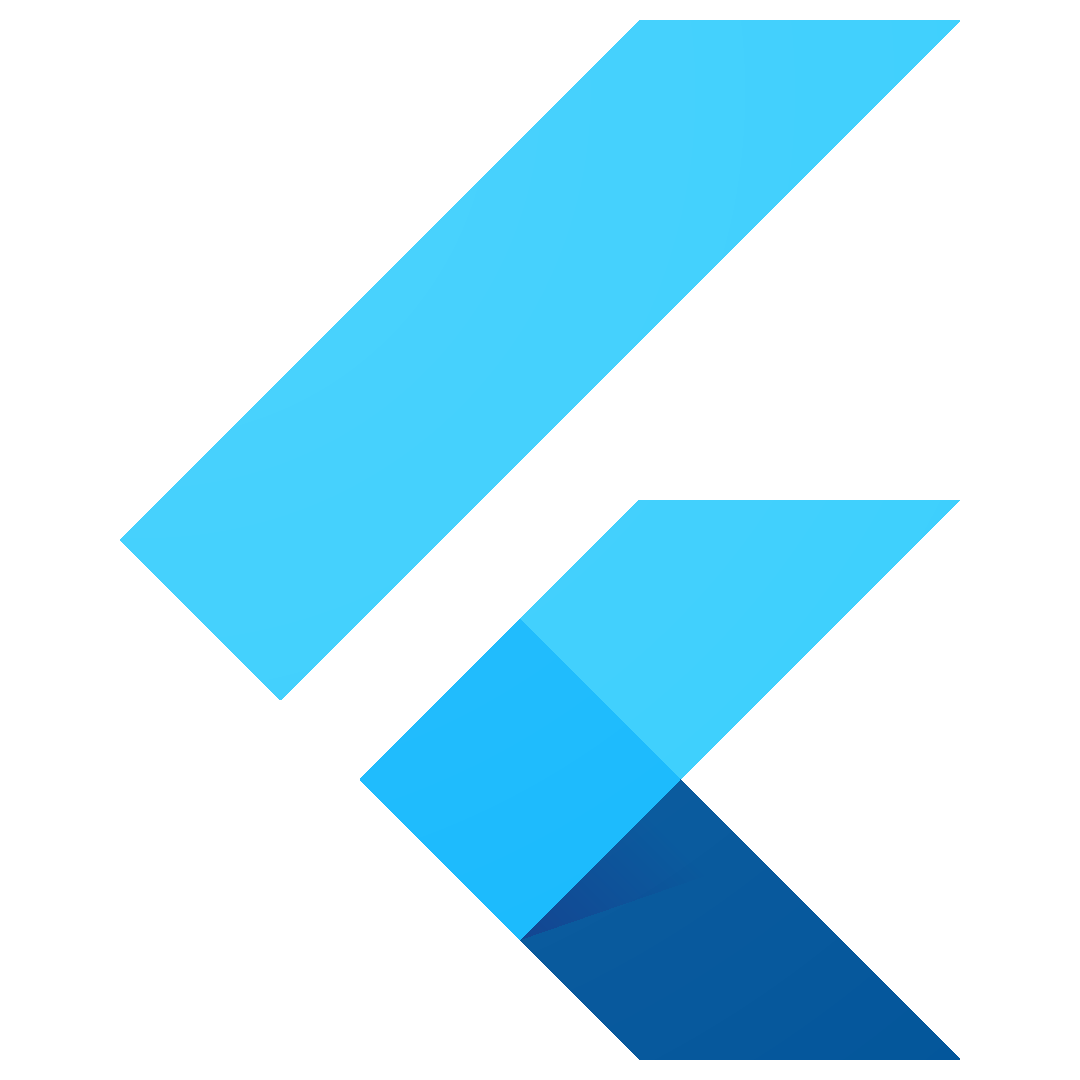
Create a floating action button that spawns other action buttons.
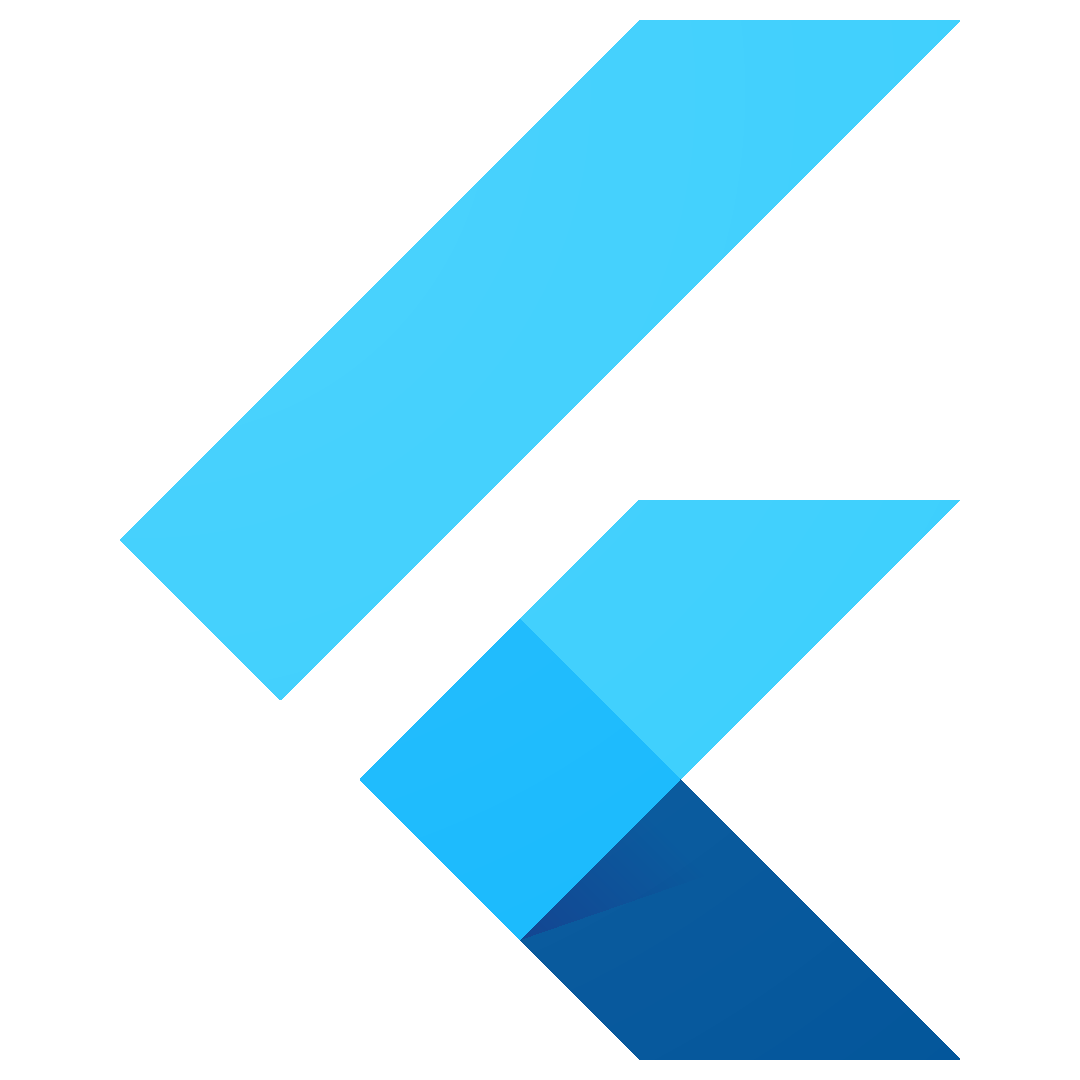
Build a drag-and-drop interaction when the user long presses.
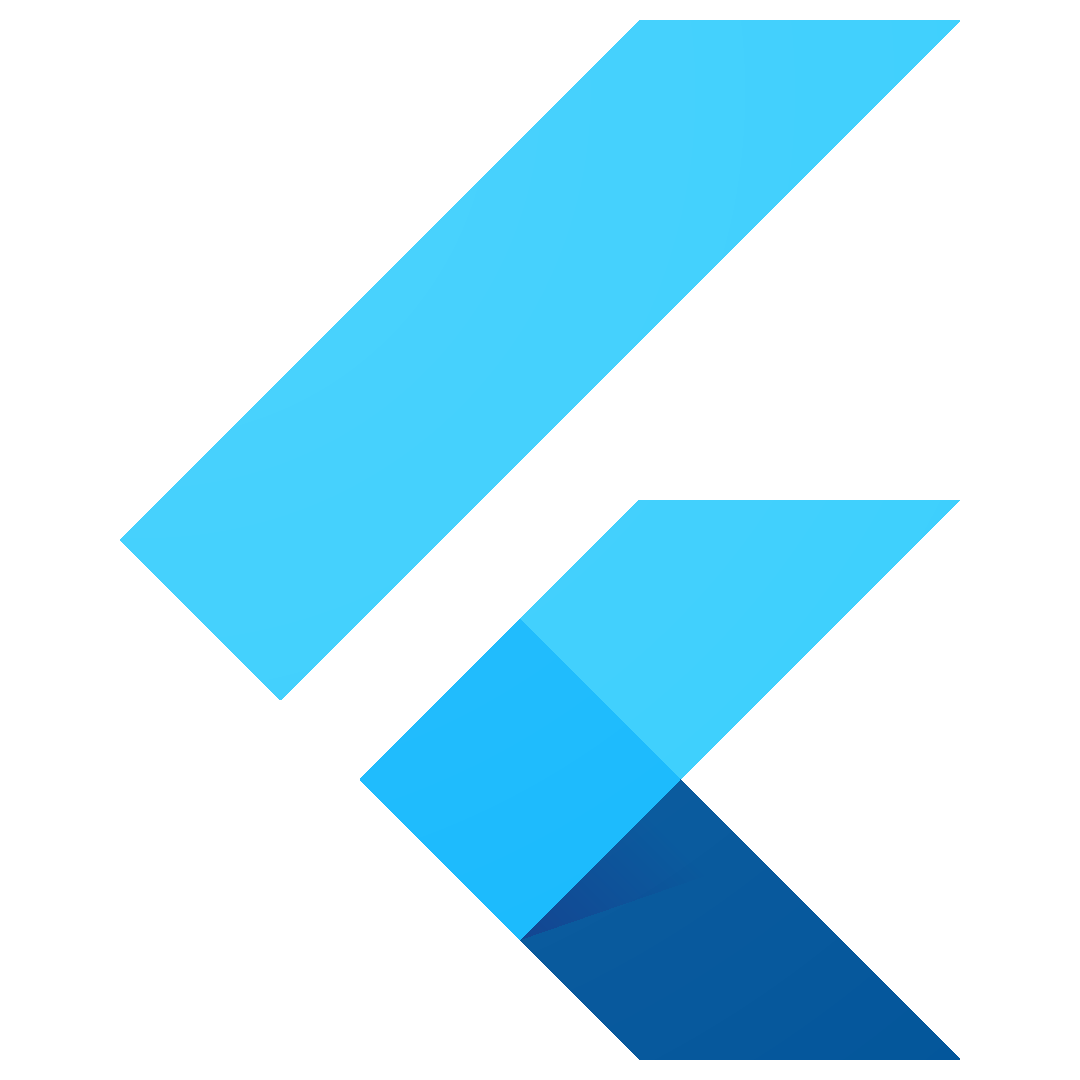
Learn how to add validation to a form.
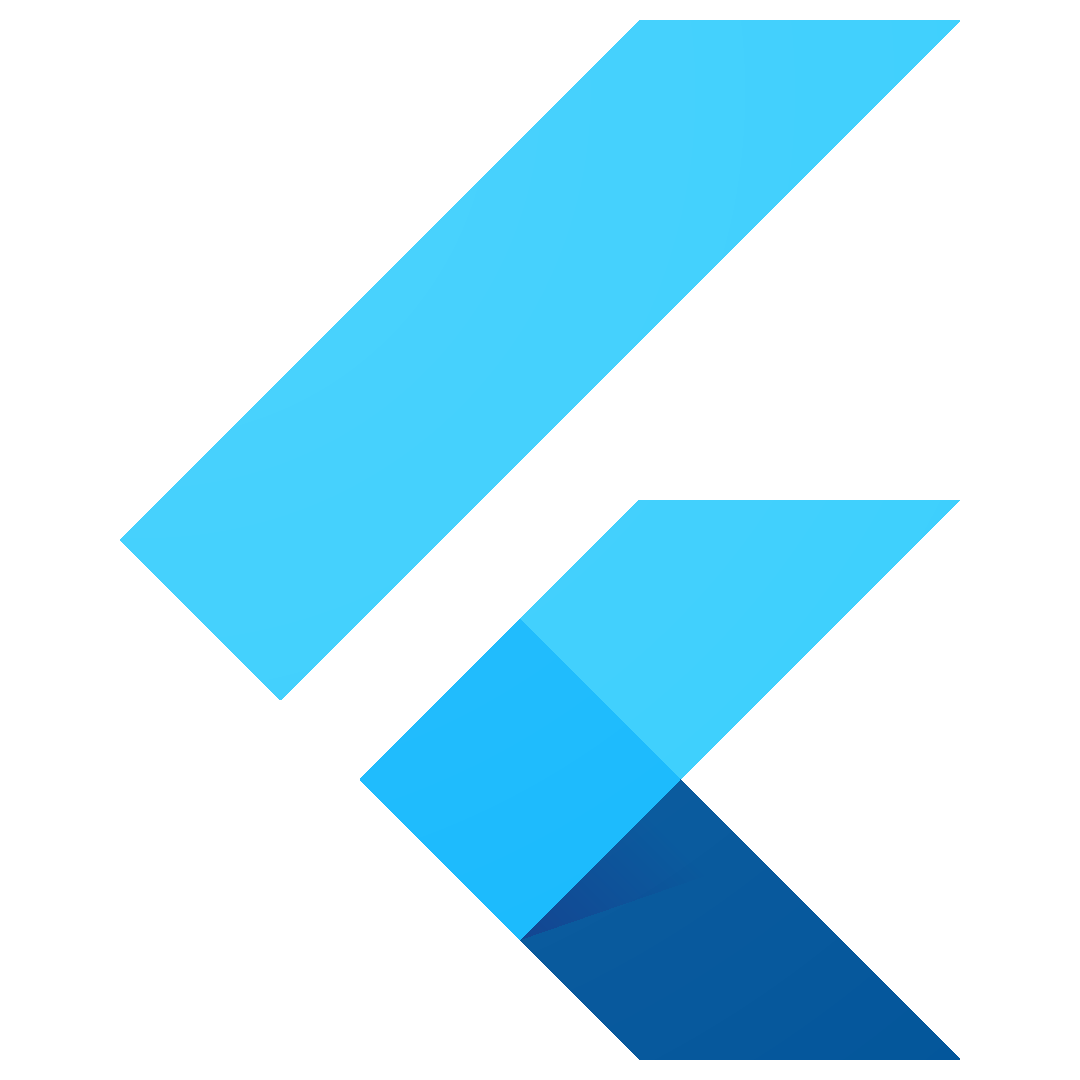
In this recipe, explore how to create and style text fields.
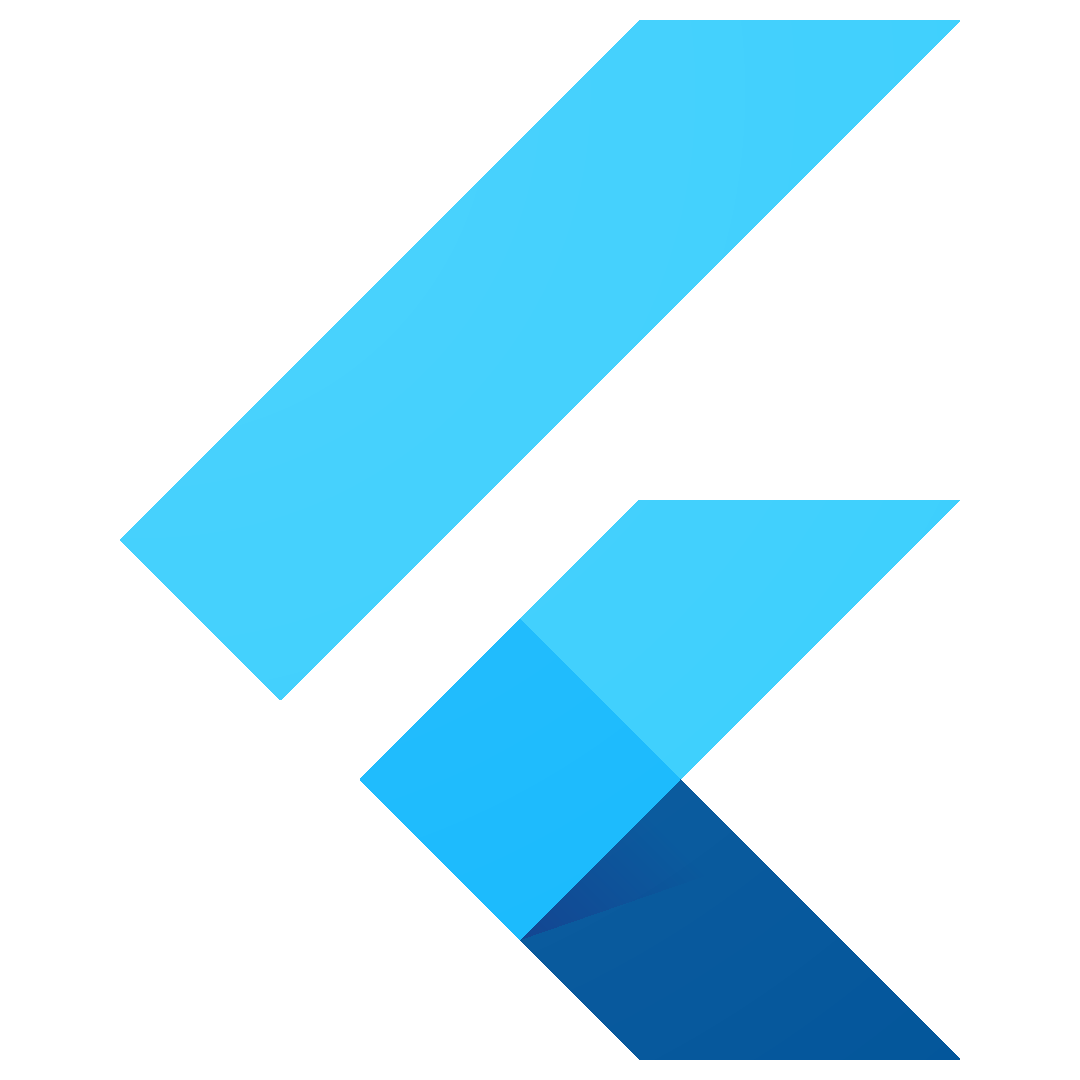
Shift focus to a text field programmatically.
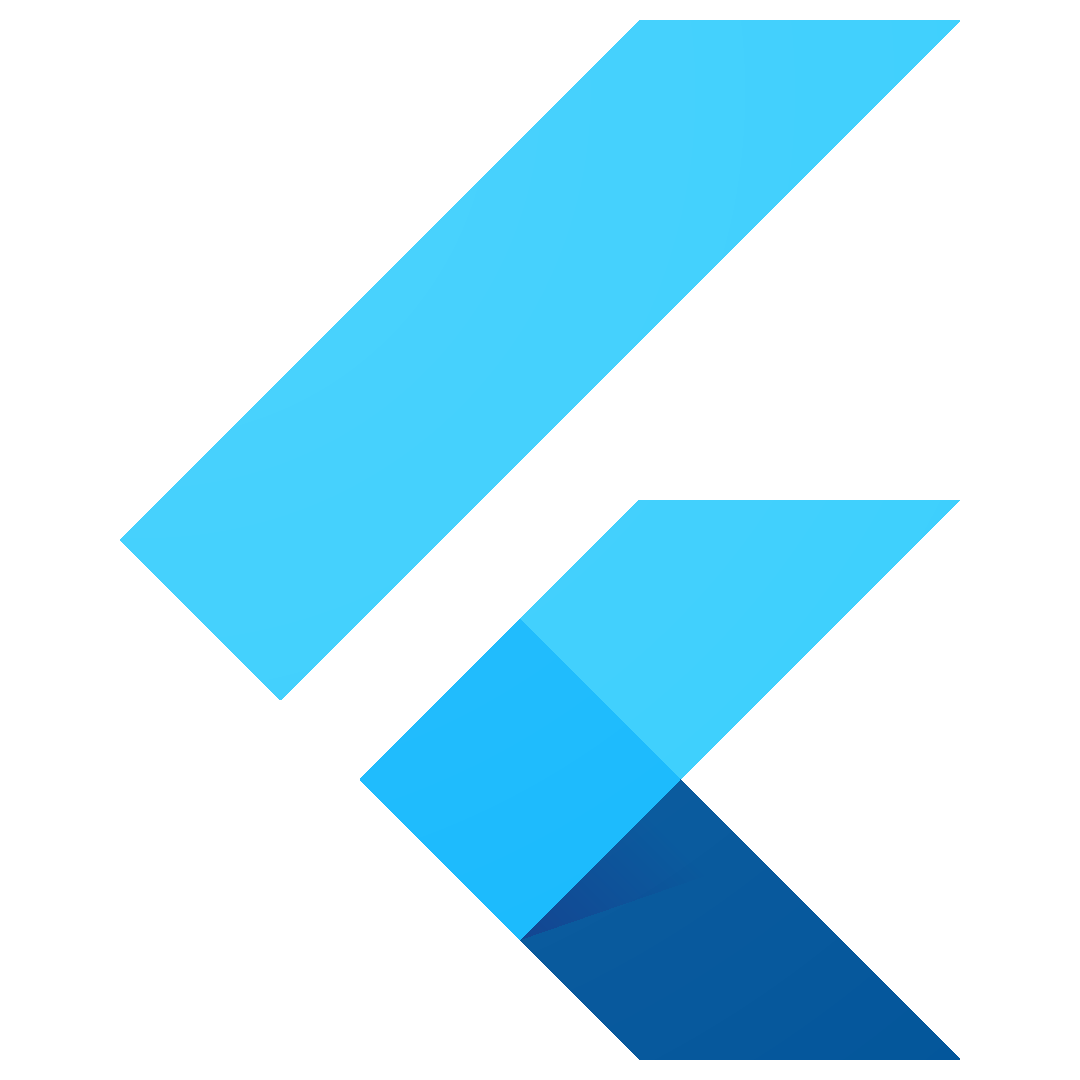
Listen for changes to a TextField using a callback.
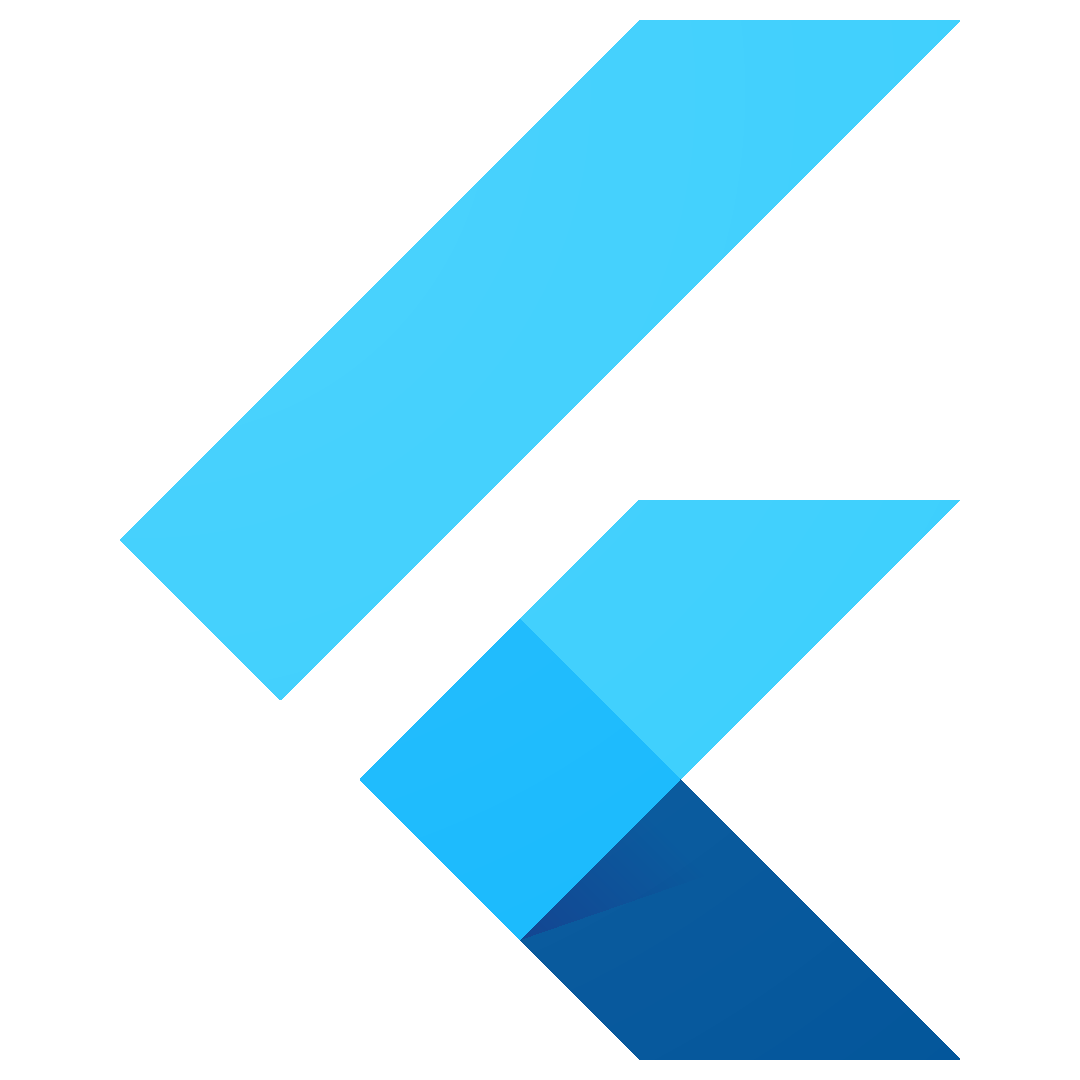
Learn how to retrieve the text a user has entered into a text field.
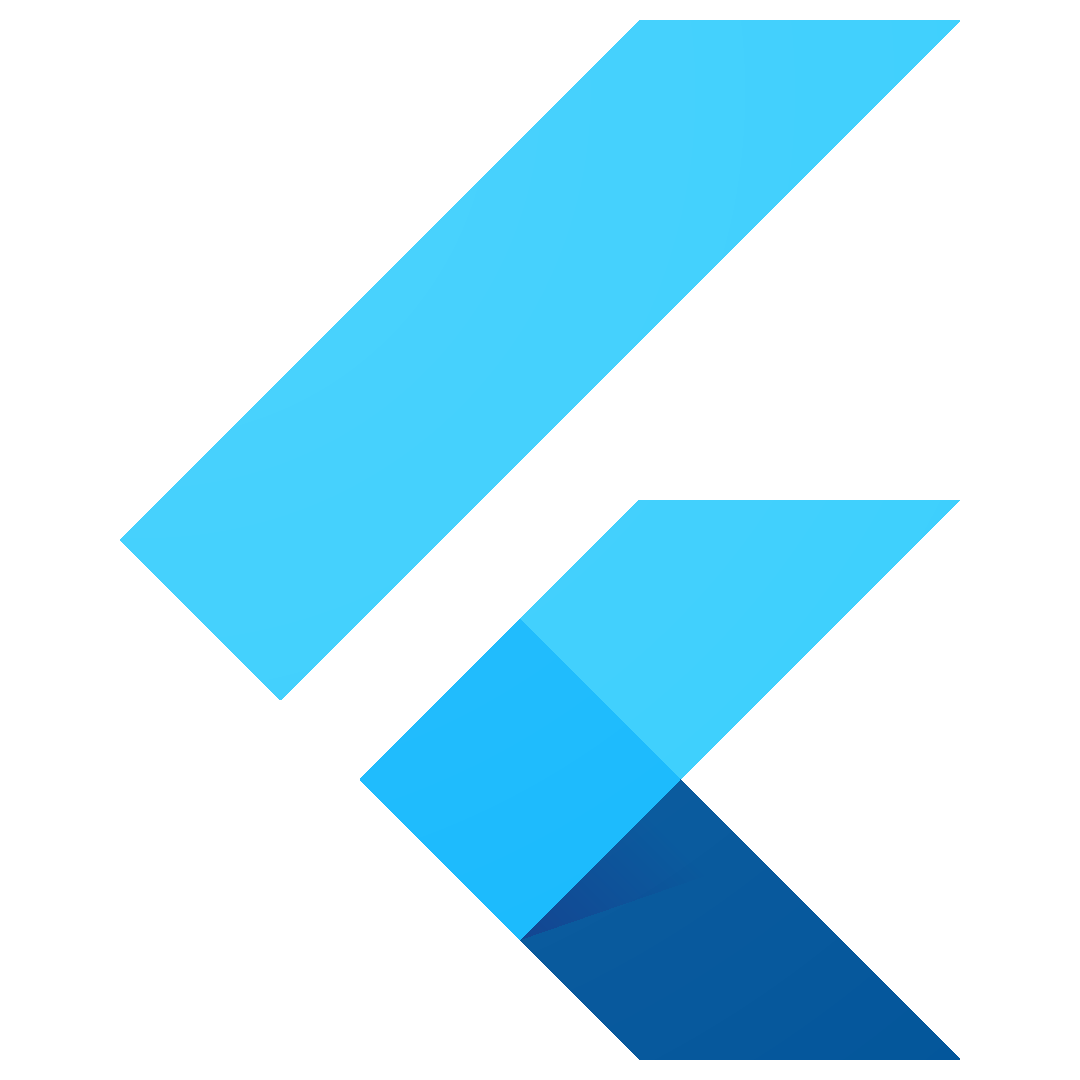
Use the games_services package to add leaderboard functionality to your mobile game.
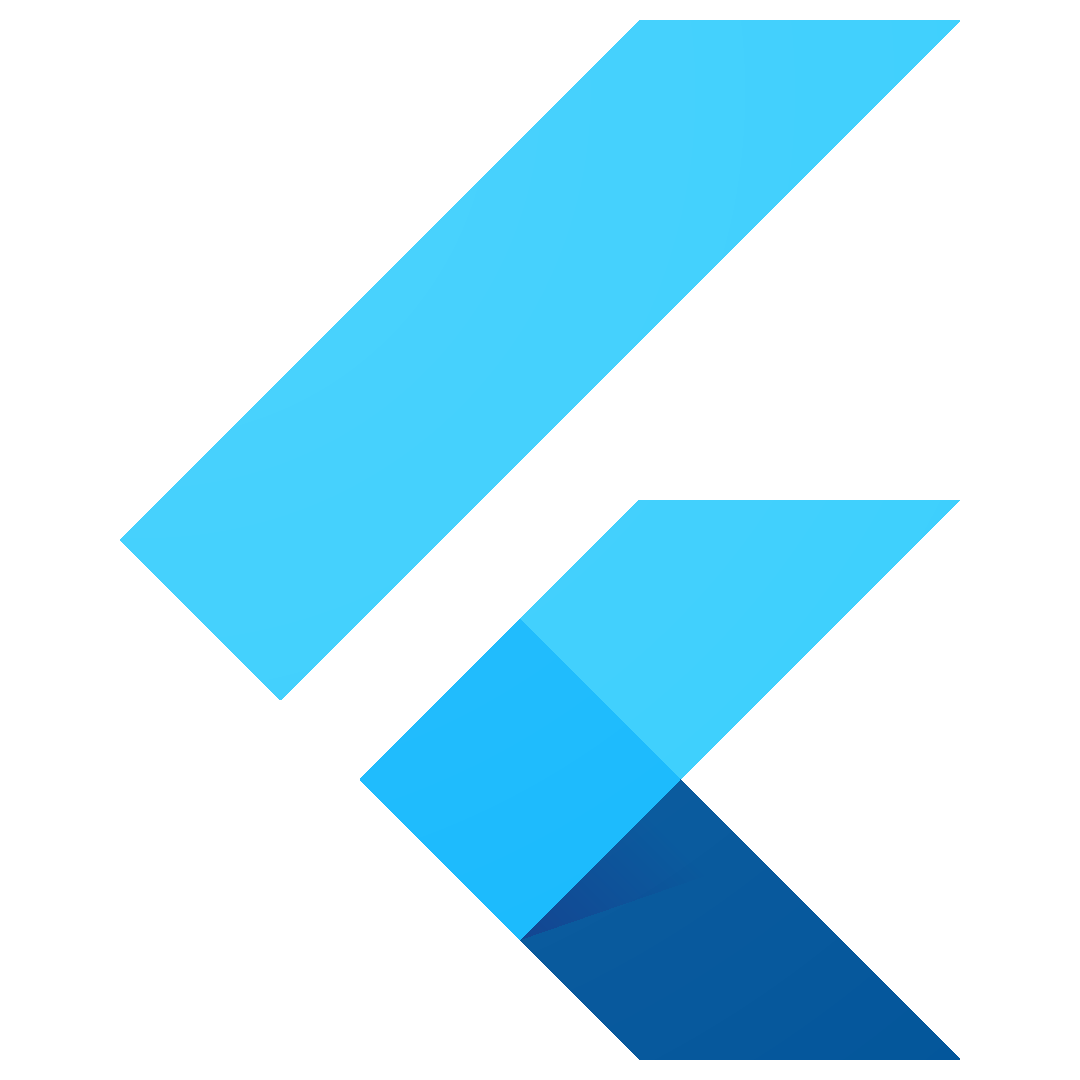
Use the cloud_firestore package to implement multiplayer capabilities in your game.
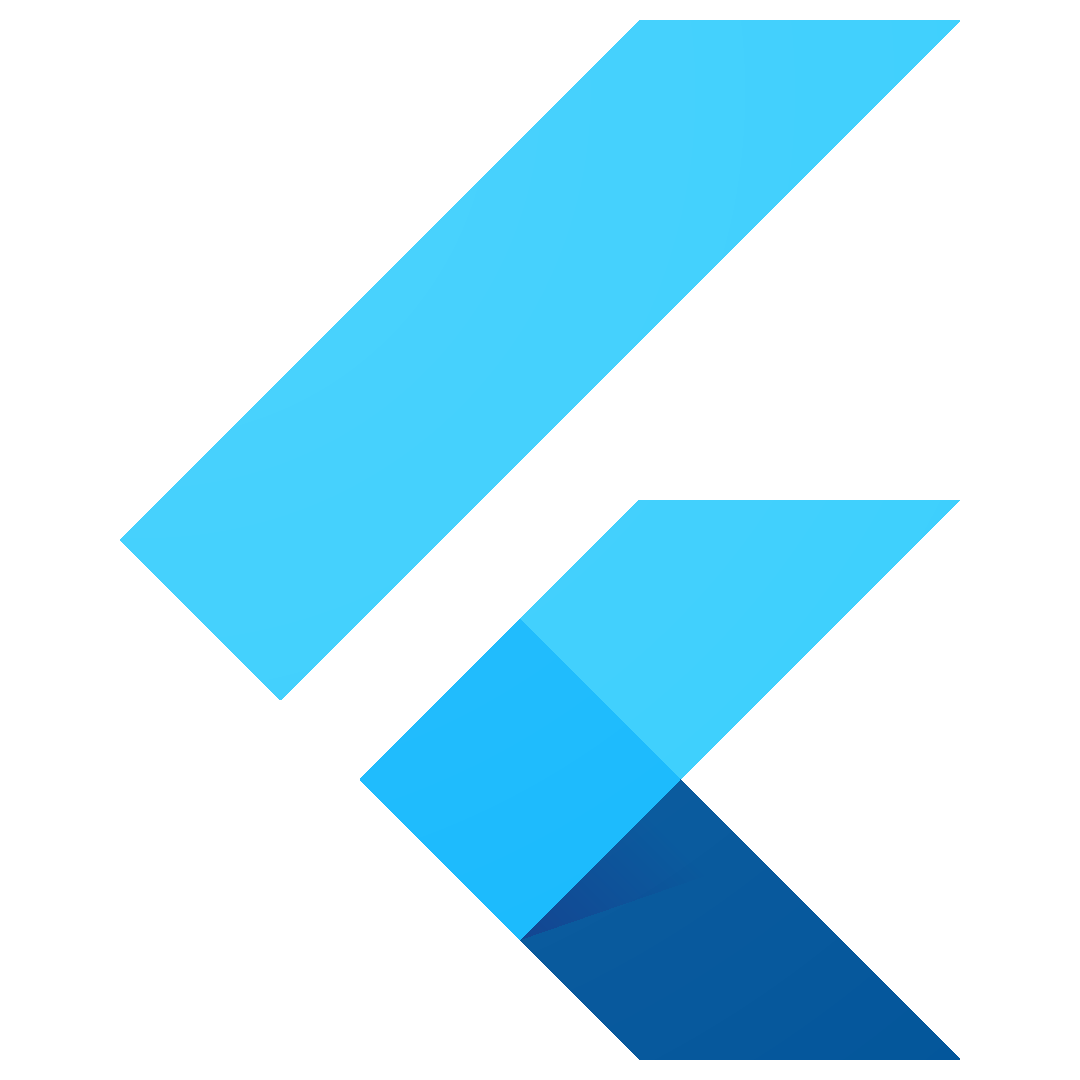
Use the google_mobile_ads package to add a banner ad to your app or game.
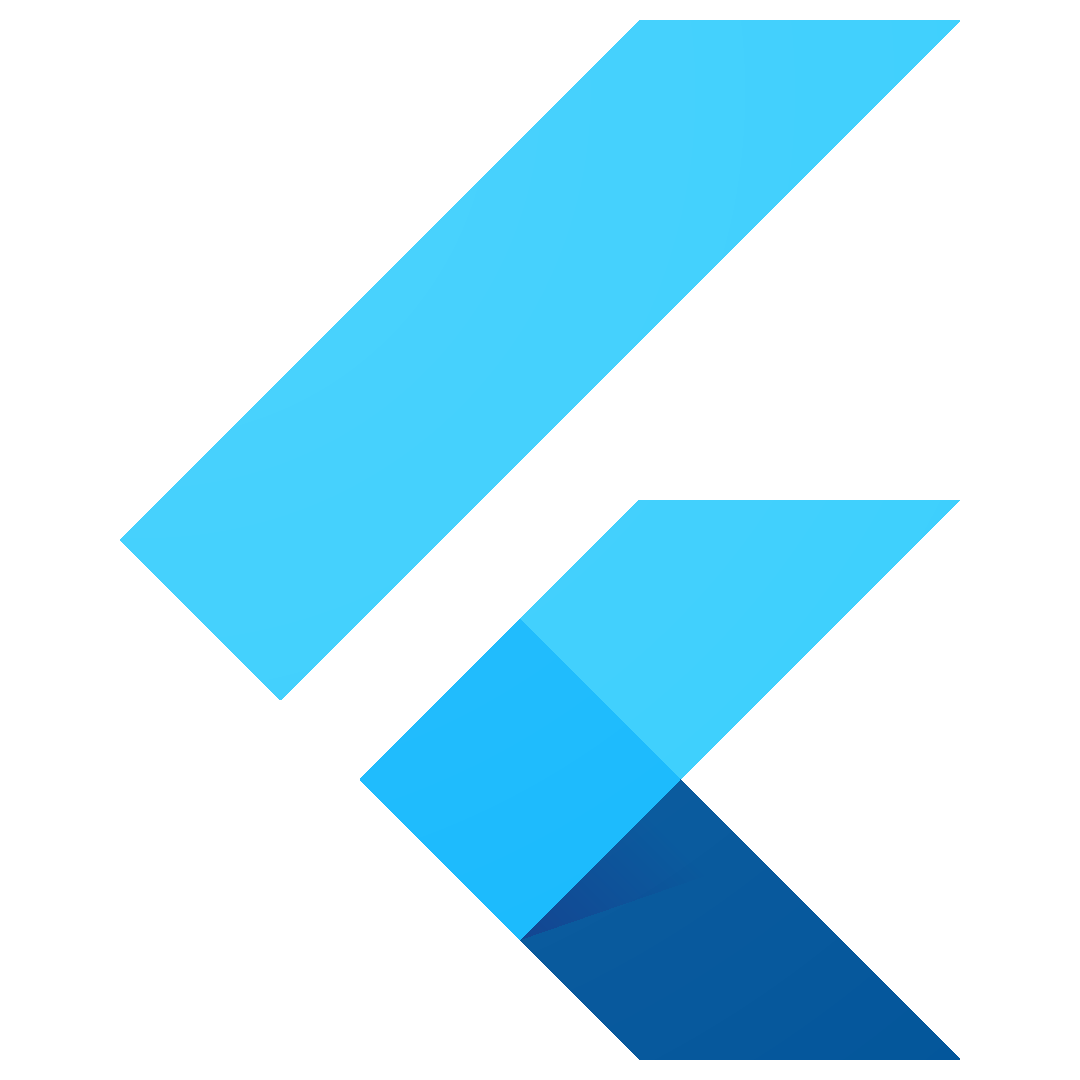
Use the Inkwell widget to display a ripple animation.
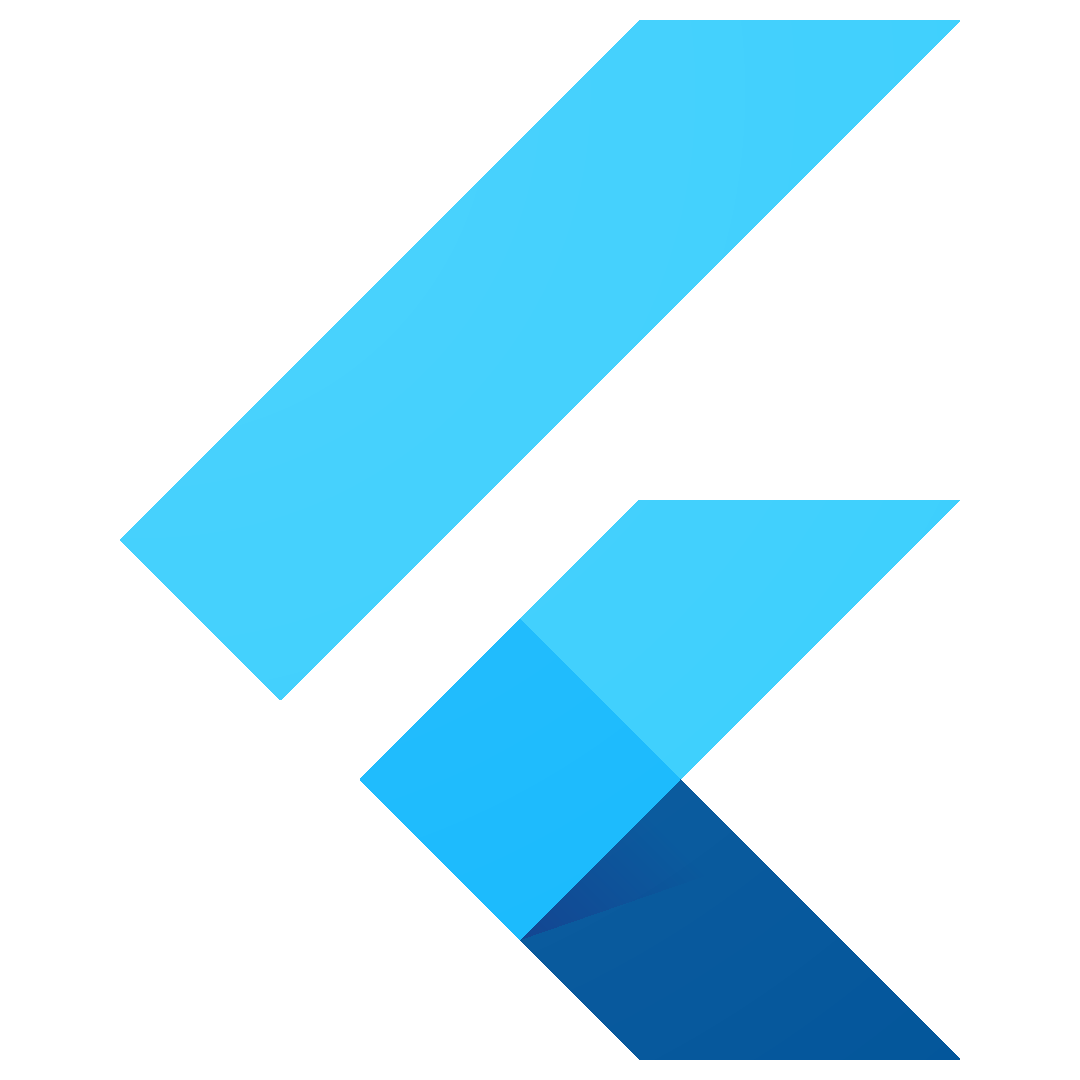
Use the GestureDetector widget to respond to fundamental actions, such as tapping and dragging.
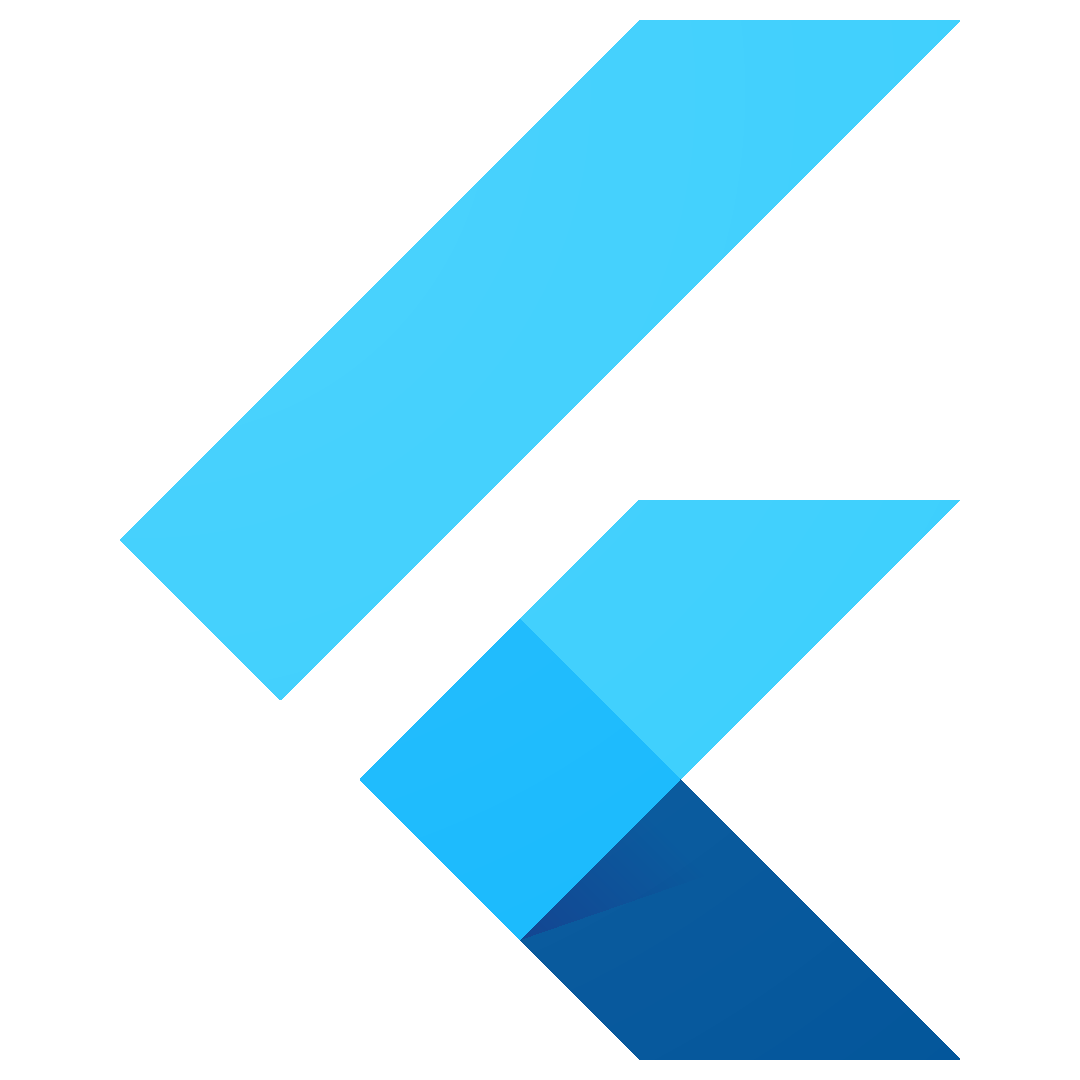
Learn how to use the Dismissible widget.
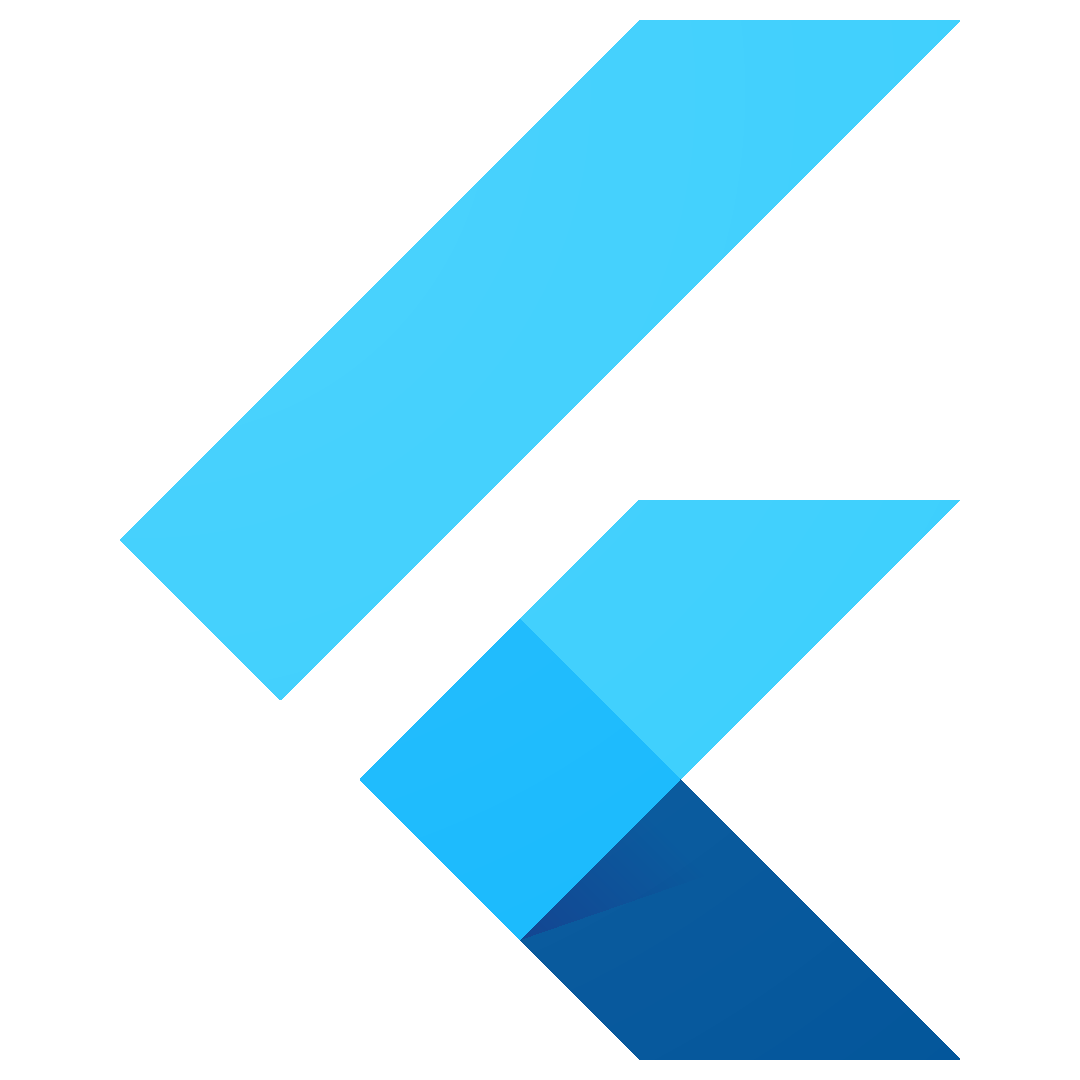
To work with images from a URL, use the Image.network() constructor.
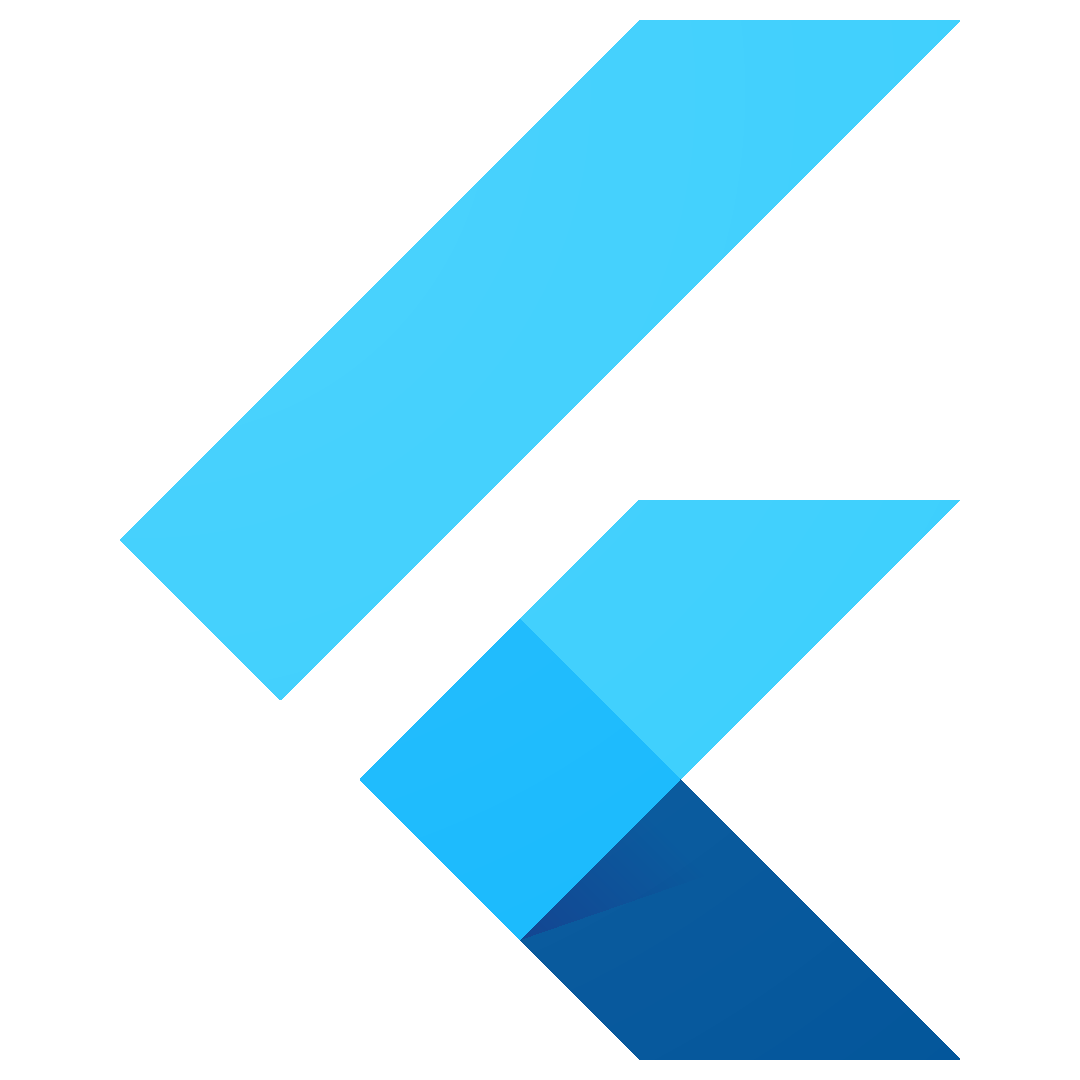
Use the FadeInImage widget to show a visual placeholder before an image loads.
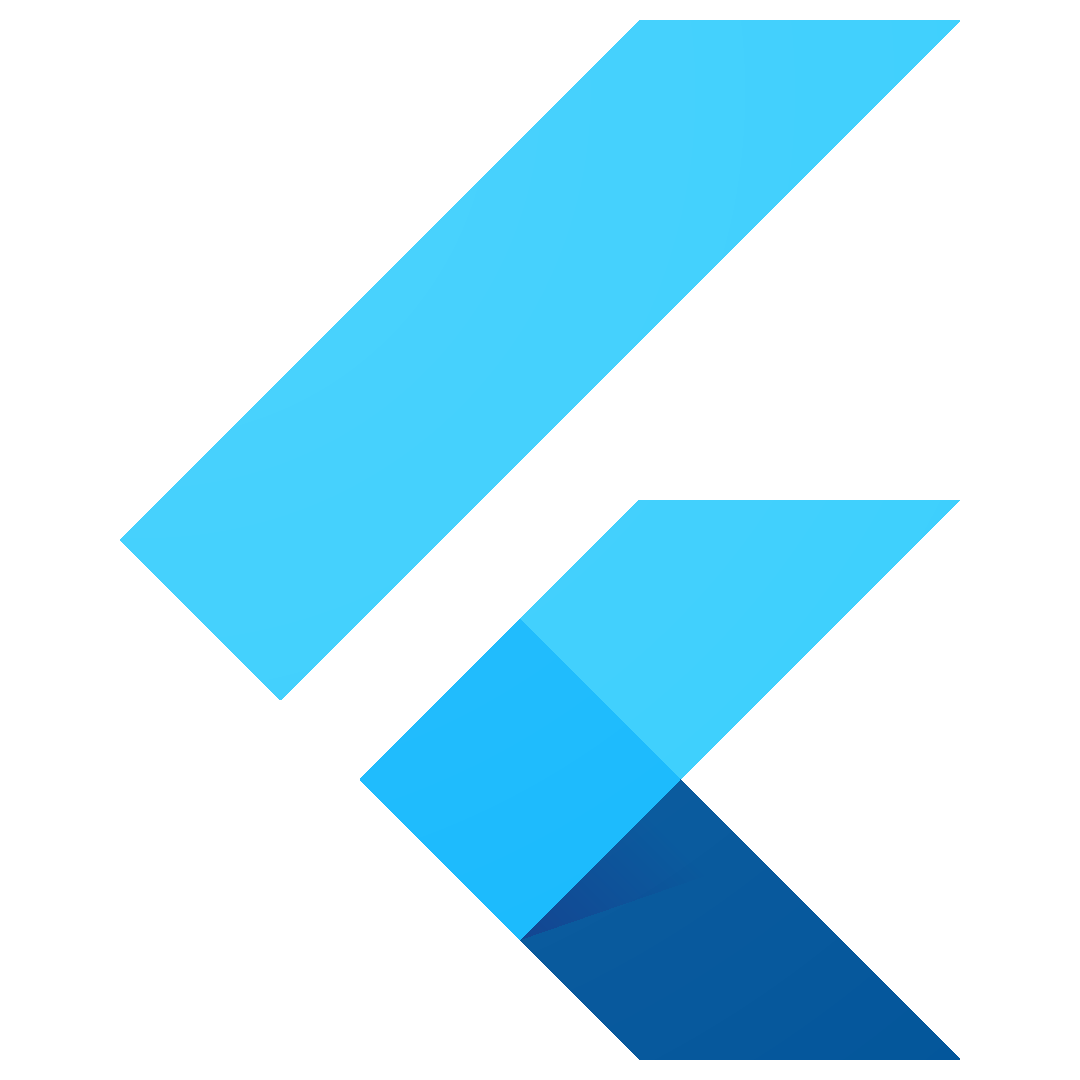
Learn to use a GridView widget.
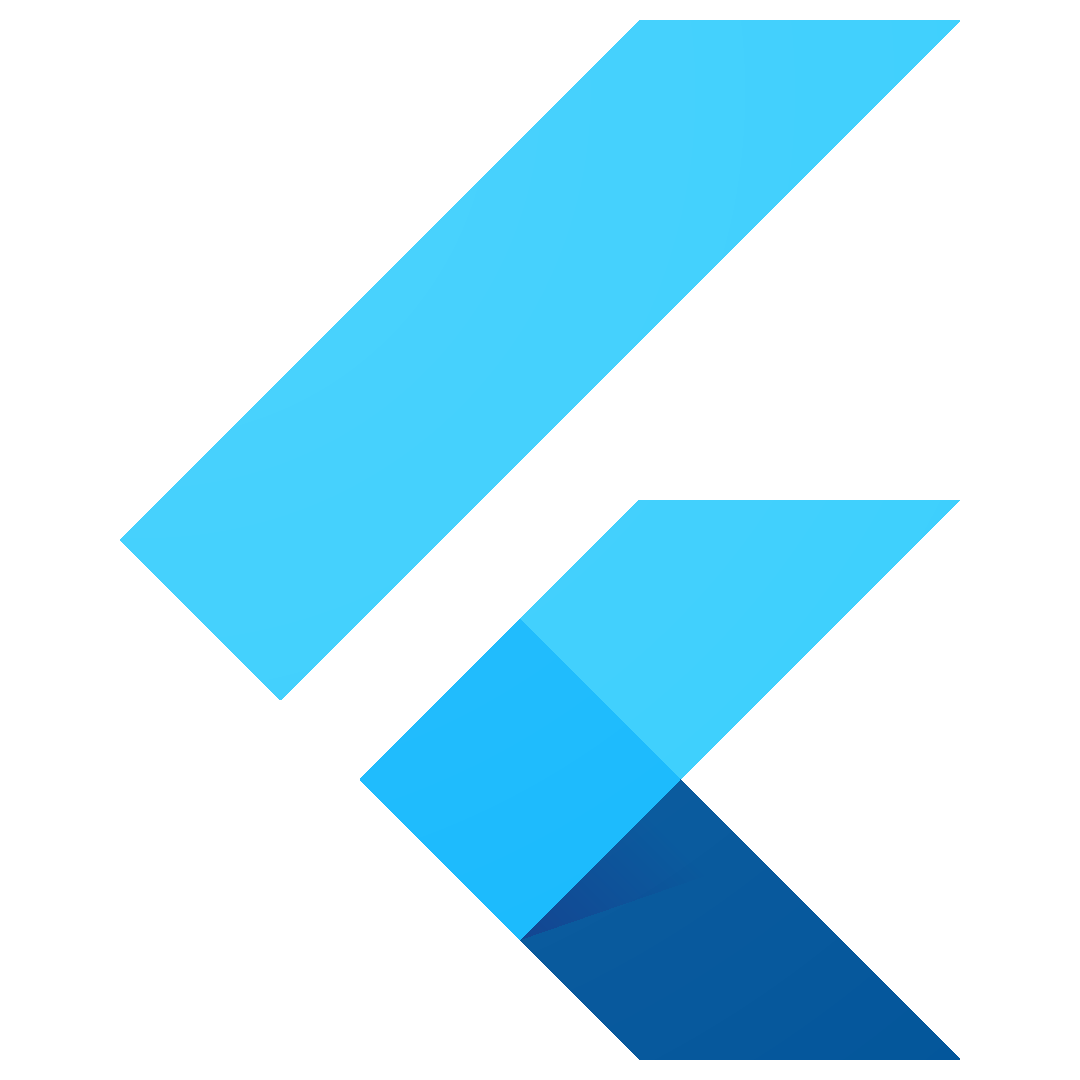
Learn to display items horizontally in a scrollable list.
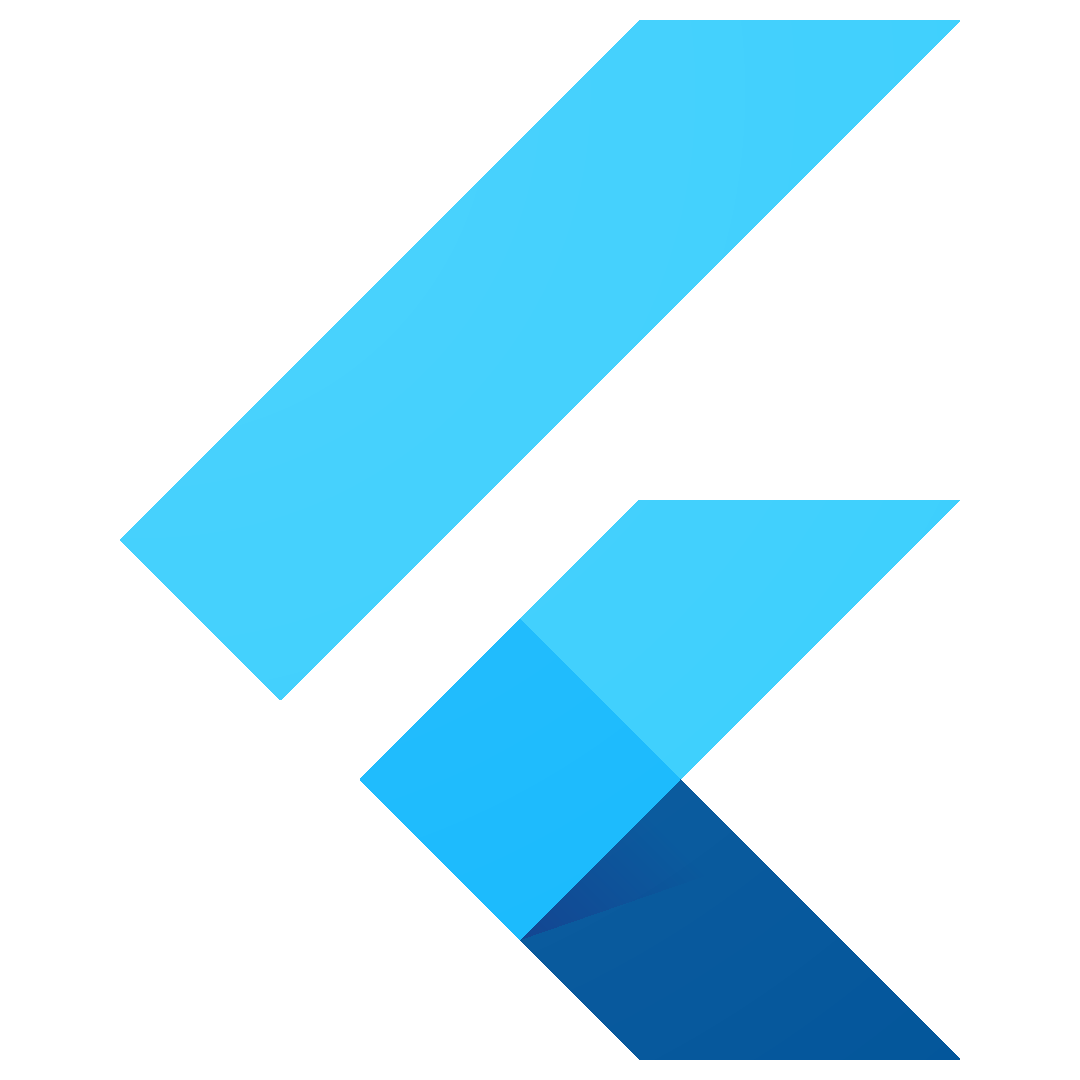
Create a list with headers followed by a few list items.
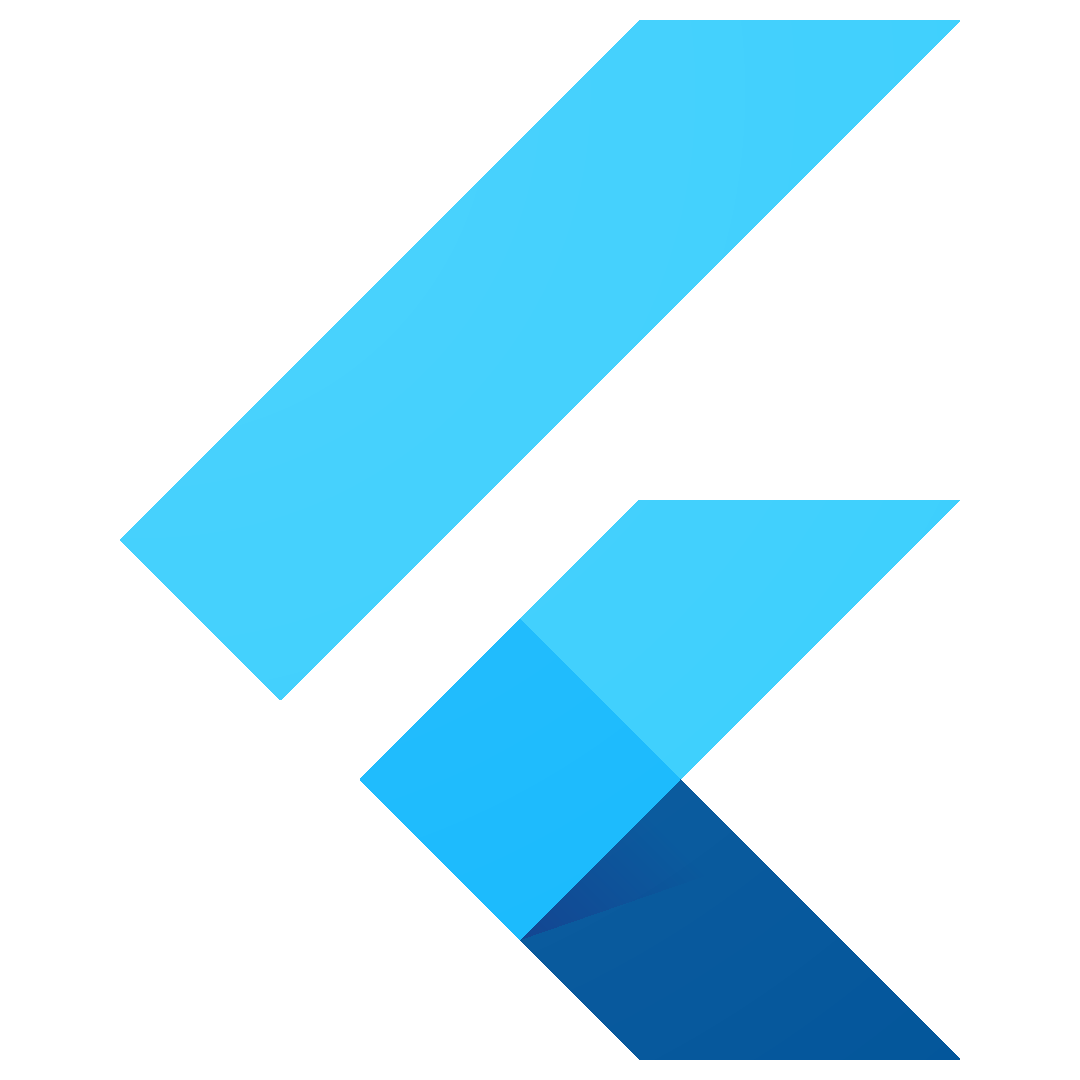
Place a floating app bar or navigation bar above a list.
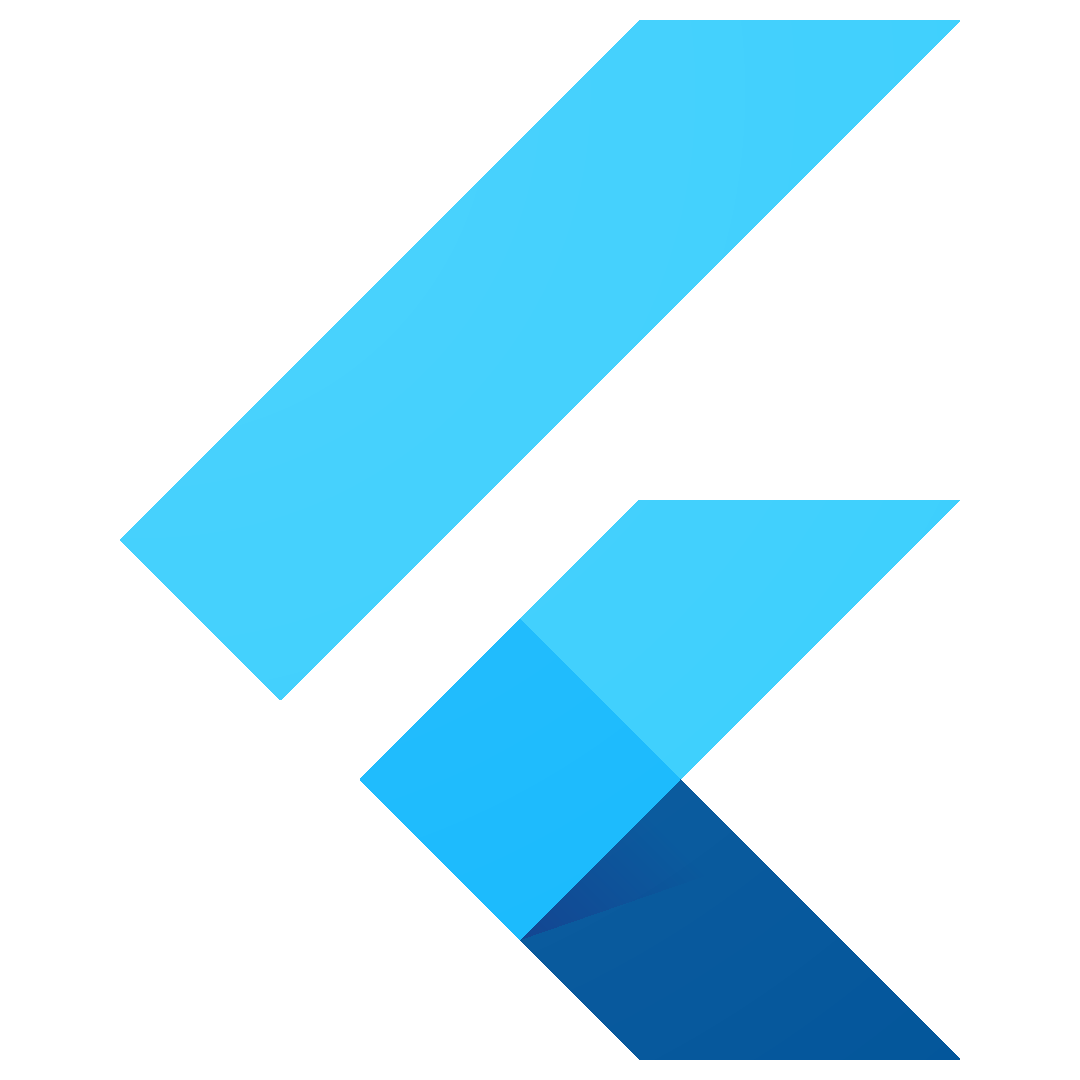
Learn to display items with the ListView widget.
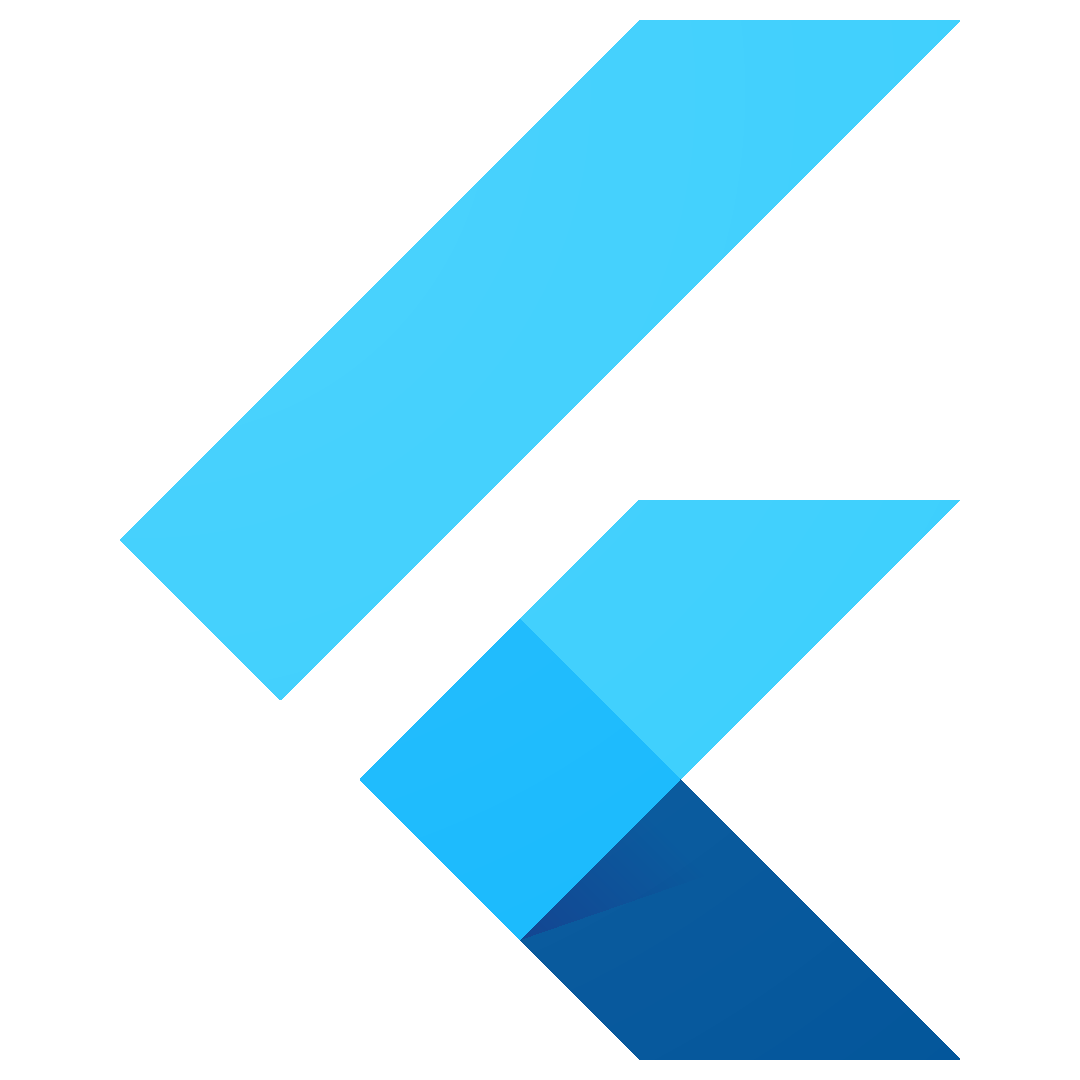
Work with longer lists with the Listview.builder constructor.
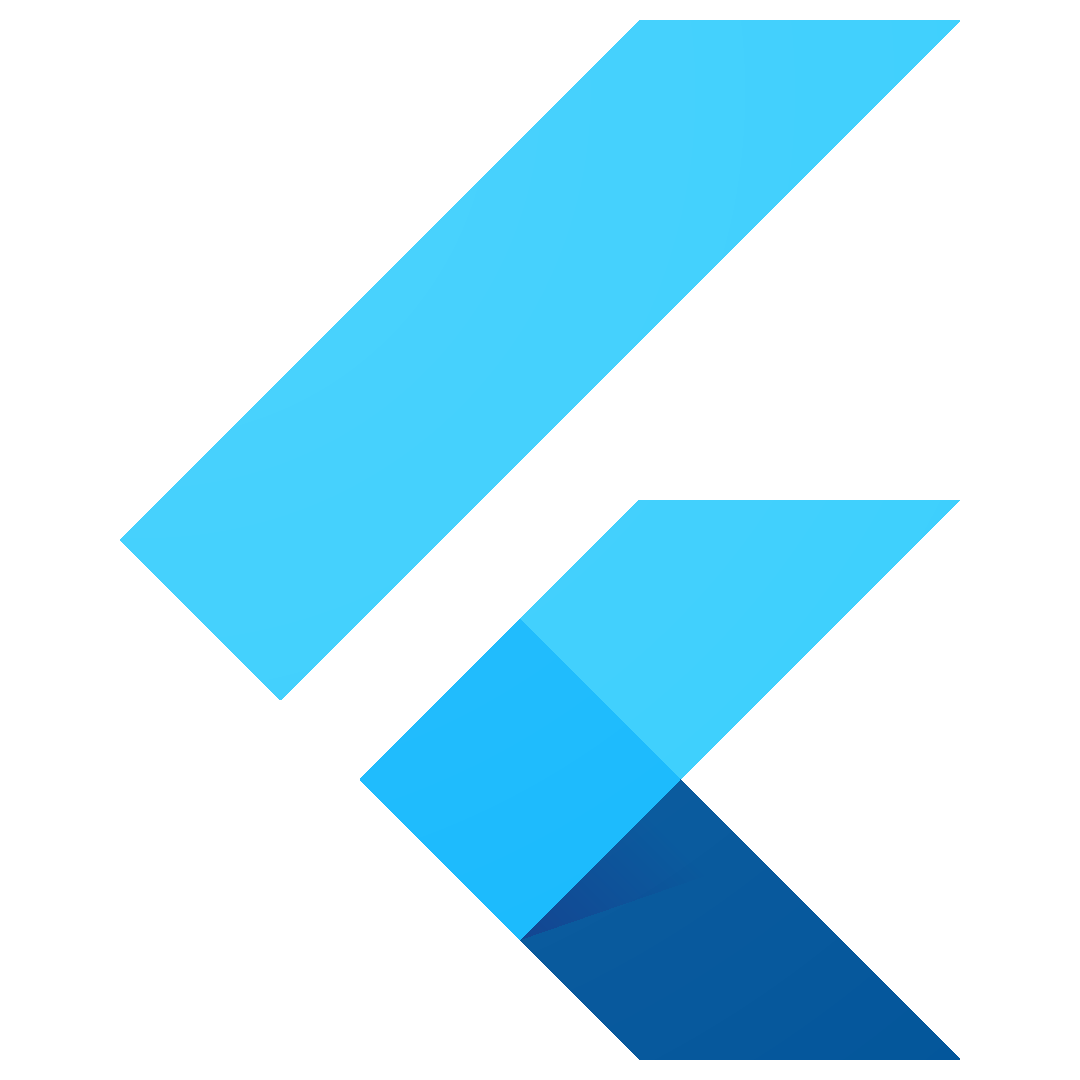
Create a list with padding between items.
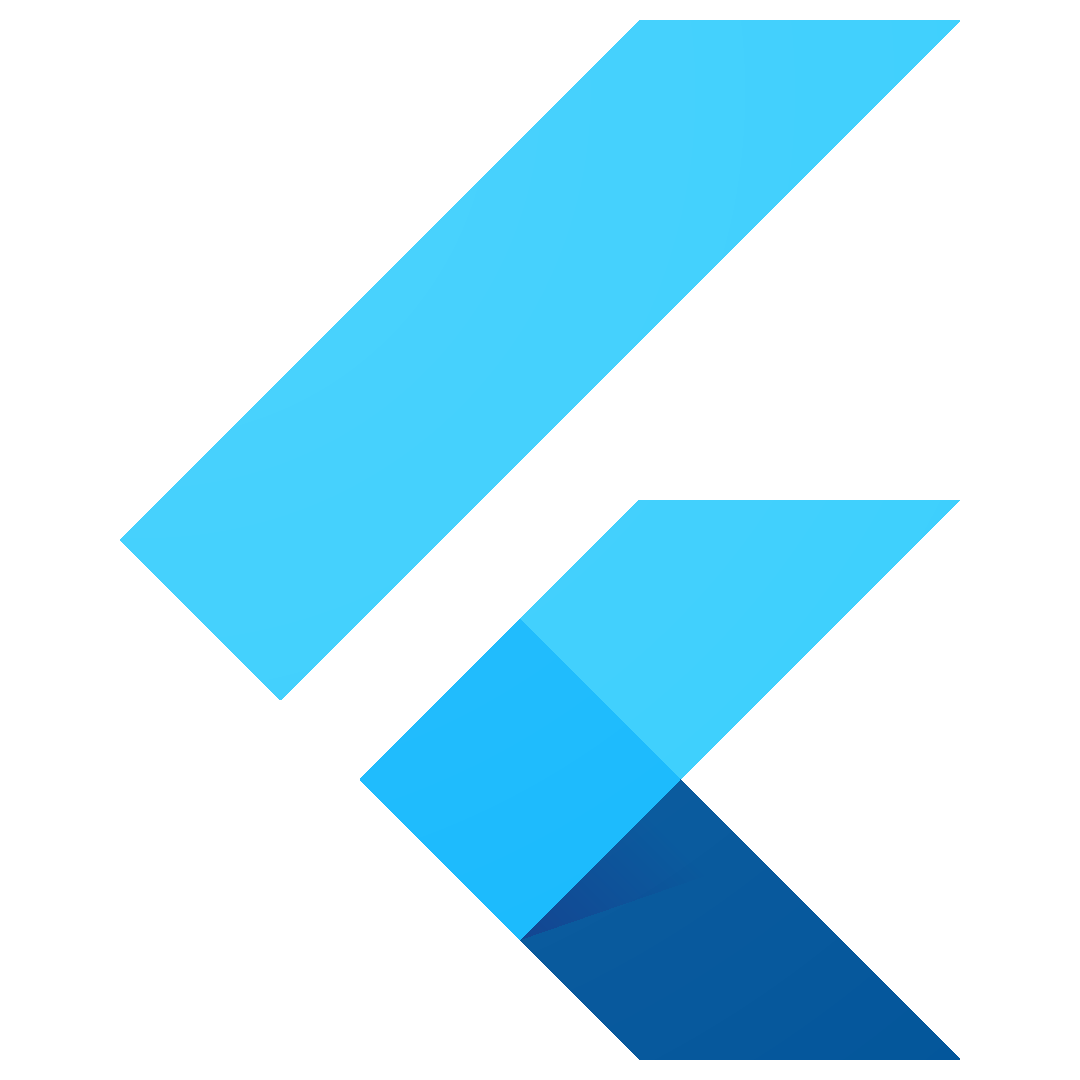
Use the Hero widget to animate a widget from one screen to the next.
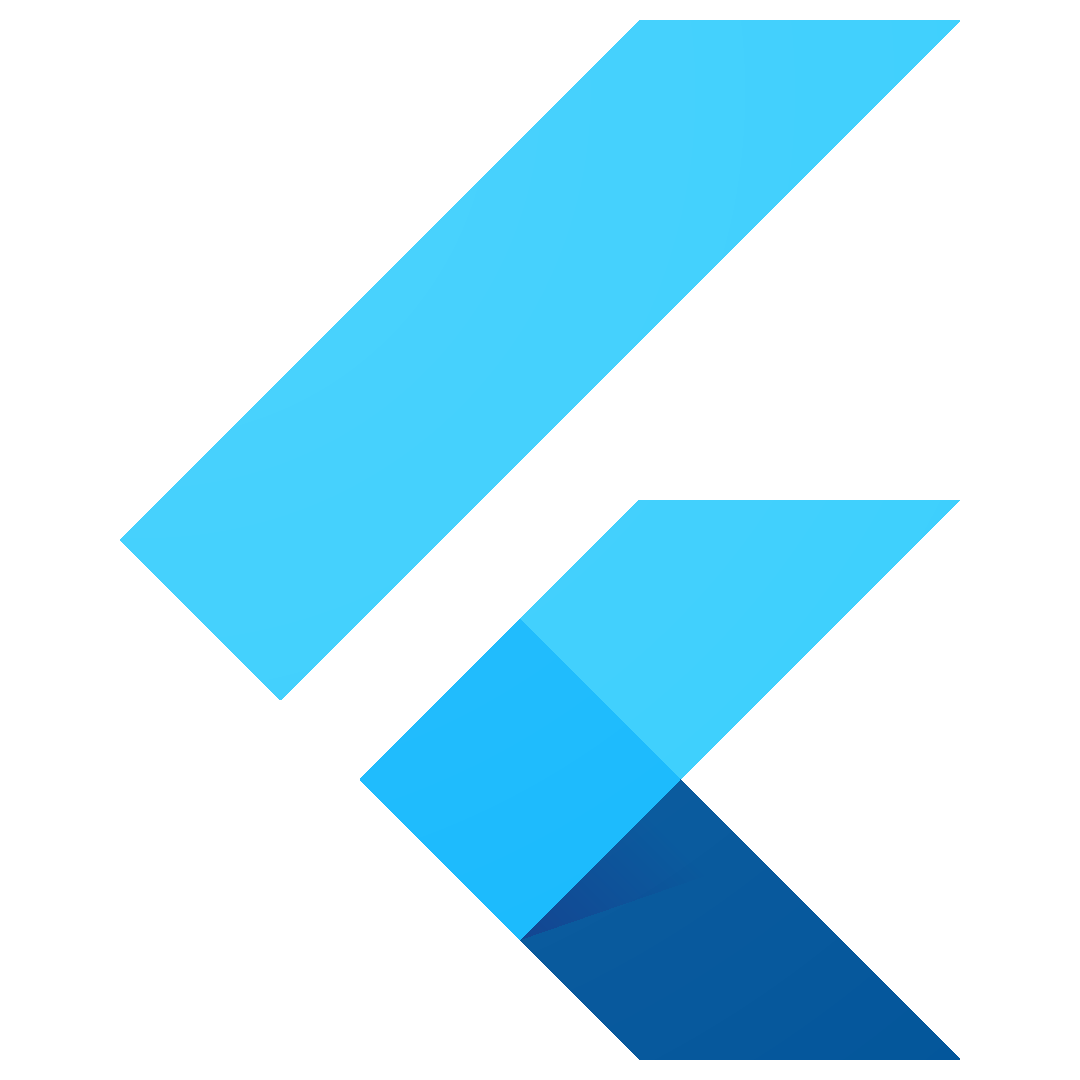
This recipe uses the Navigator to navigate to a new route.
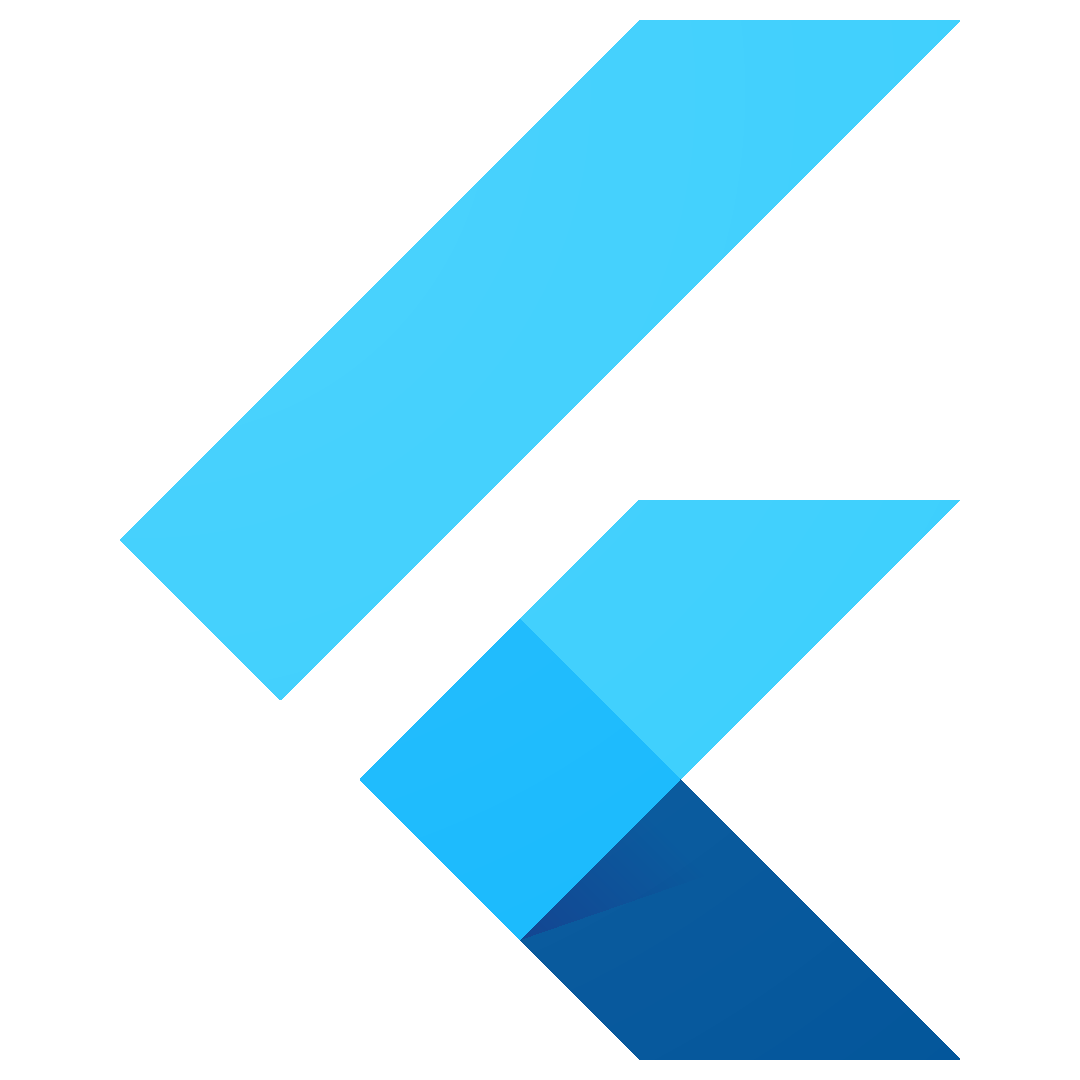
Create named routes and navigate to them.
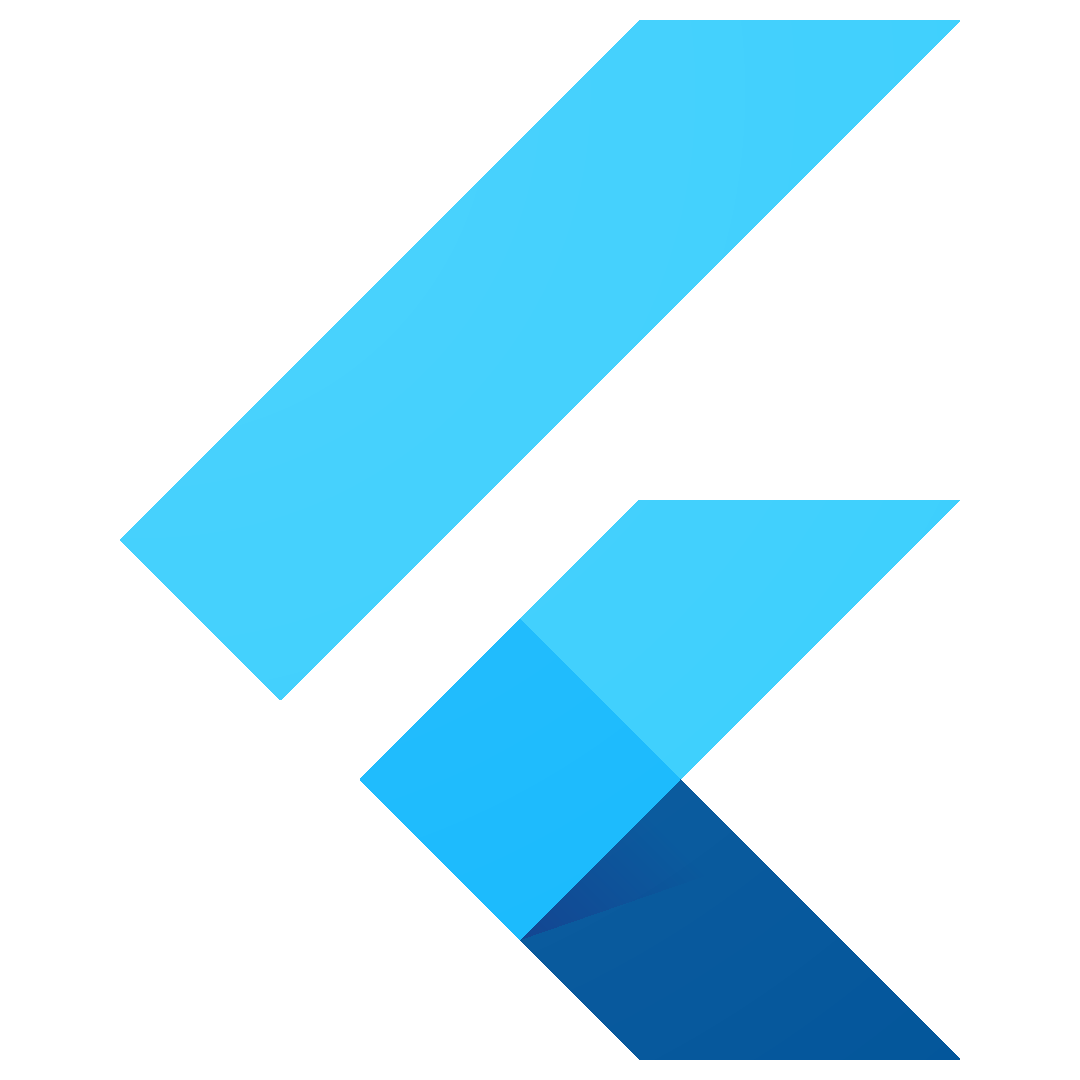
Pass arguments to a named route and read the arguments on that route.
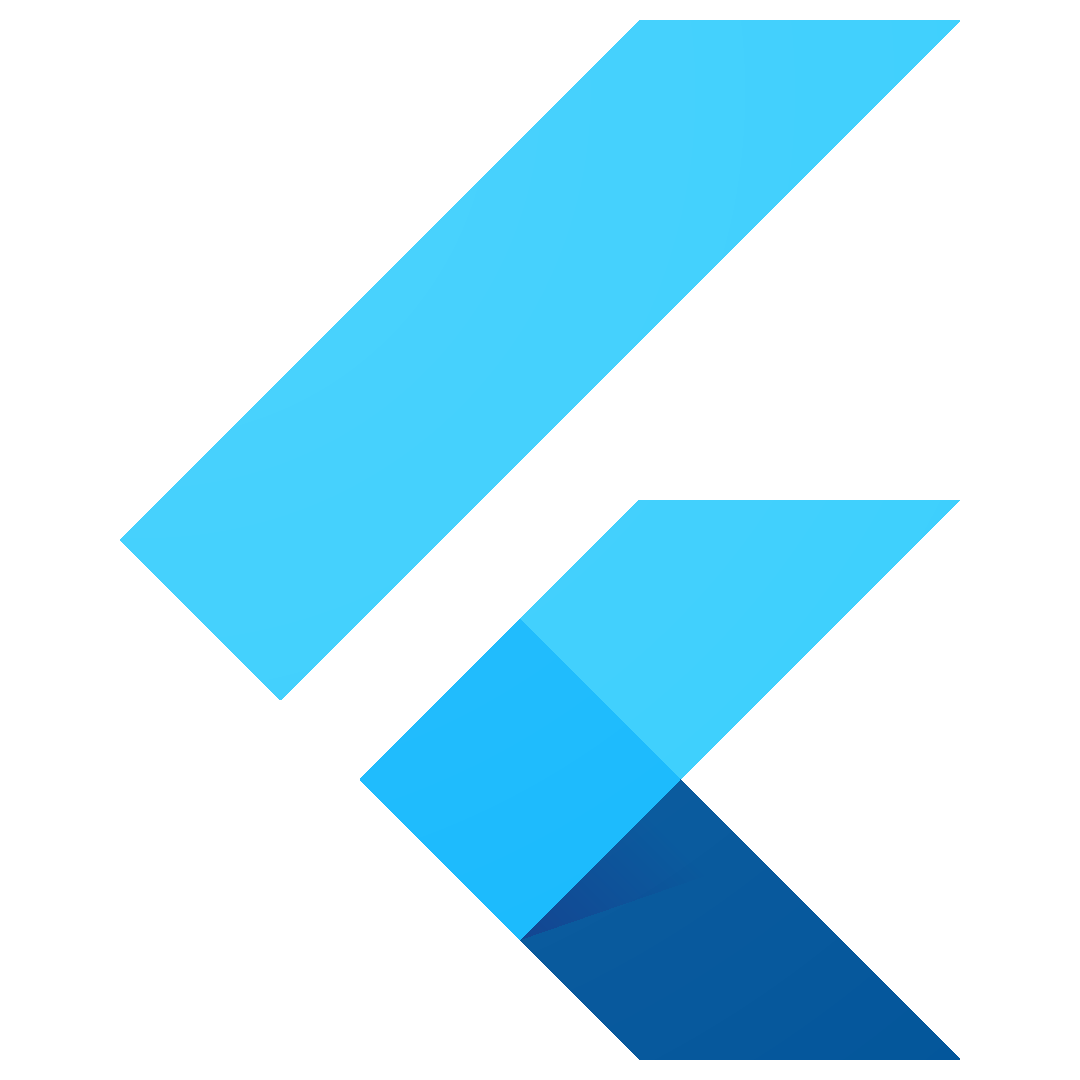
Set up deep linking on Android
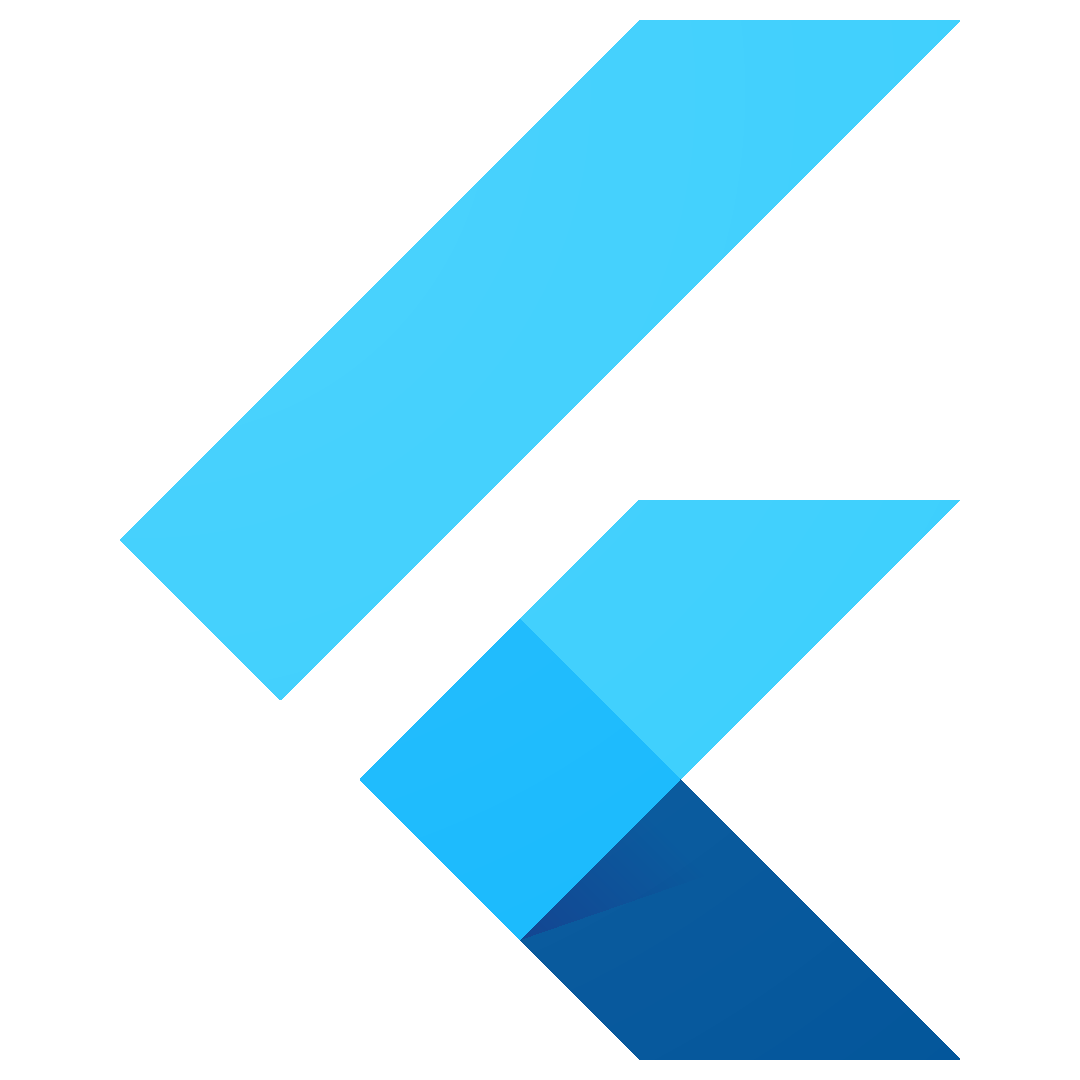
Set up deep linking on Android
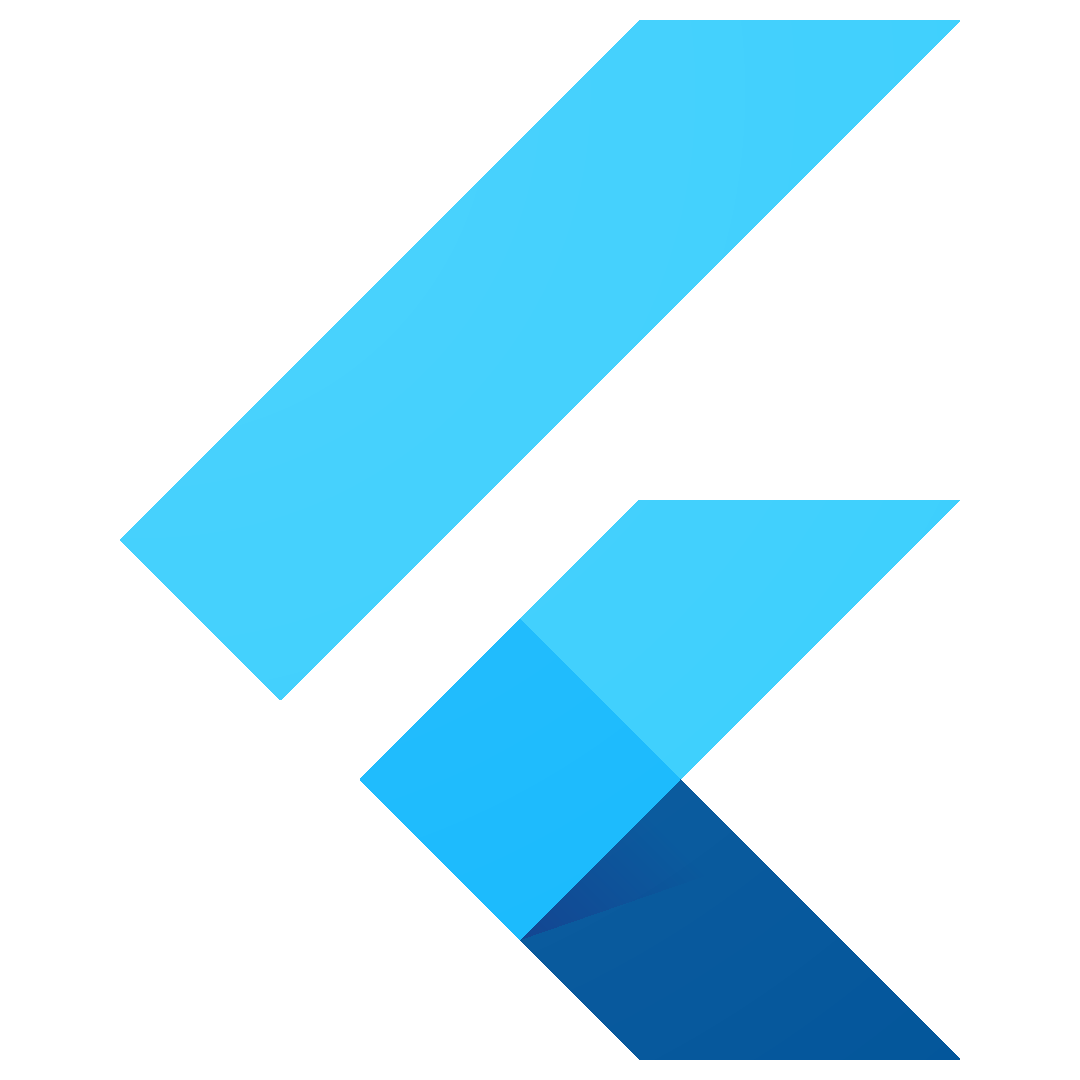
Return data from one screen to another with the Navigator.pop method.
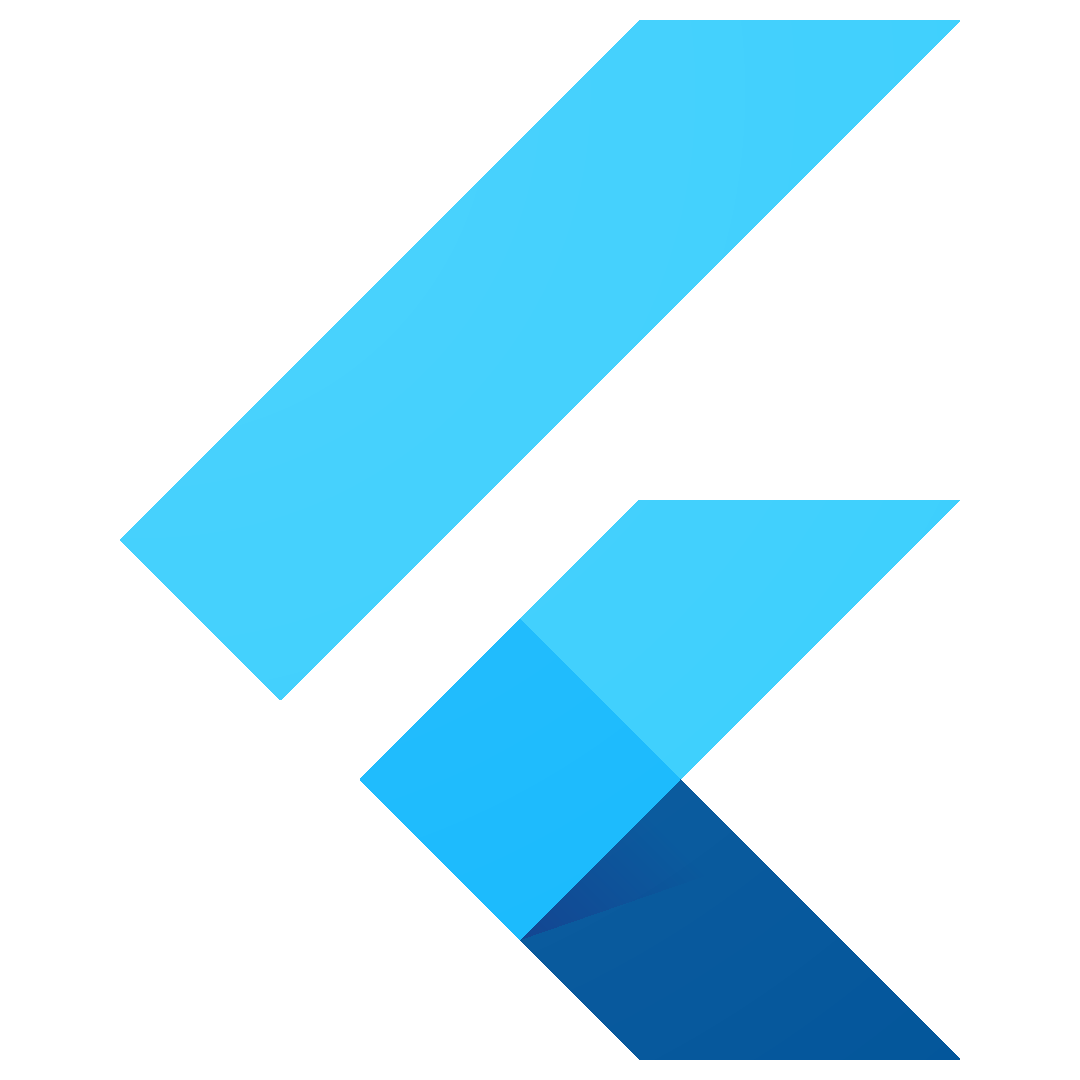
Send data from one screen to new one.
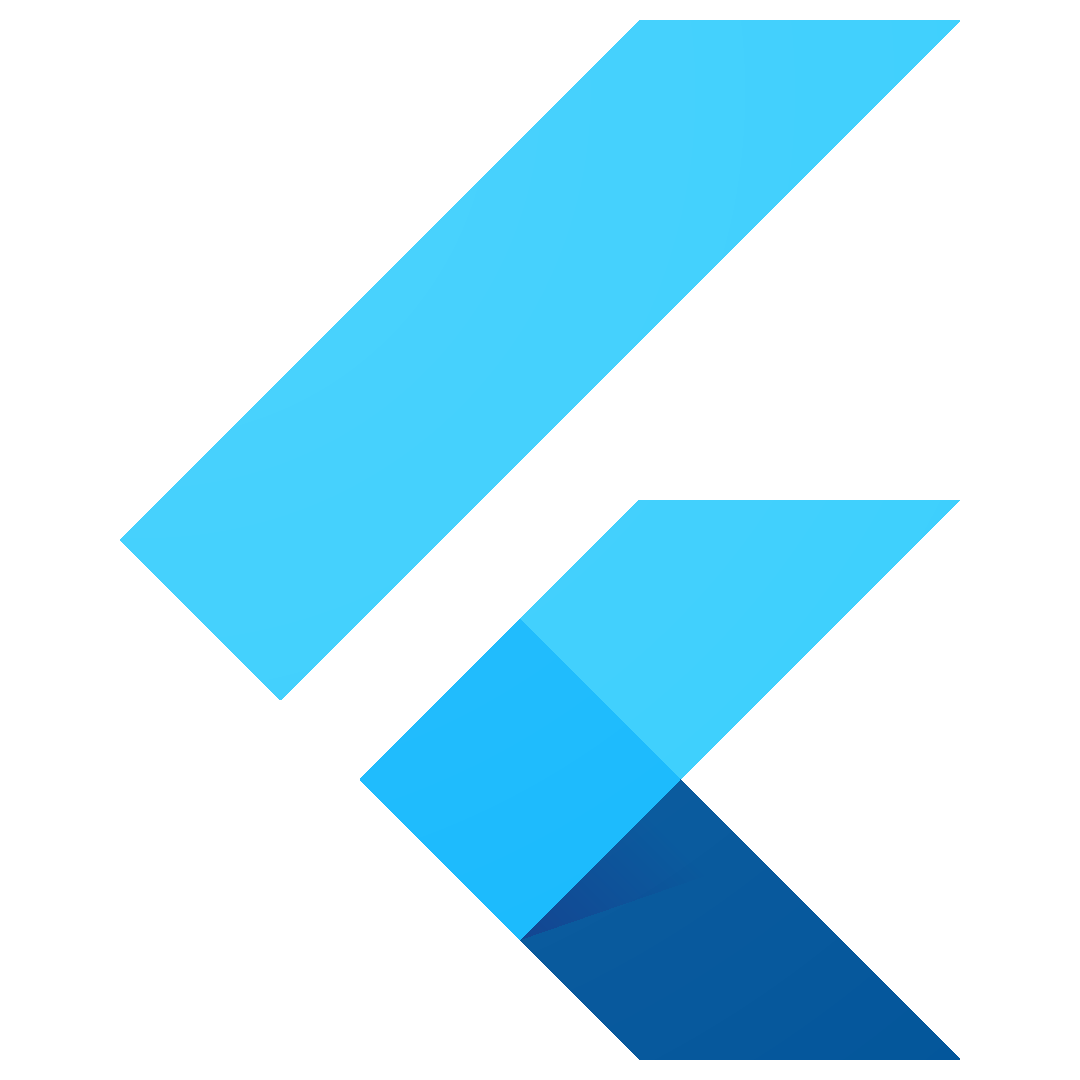
Learn to use HTTP in your app.
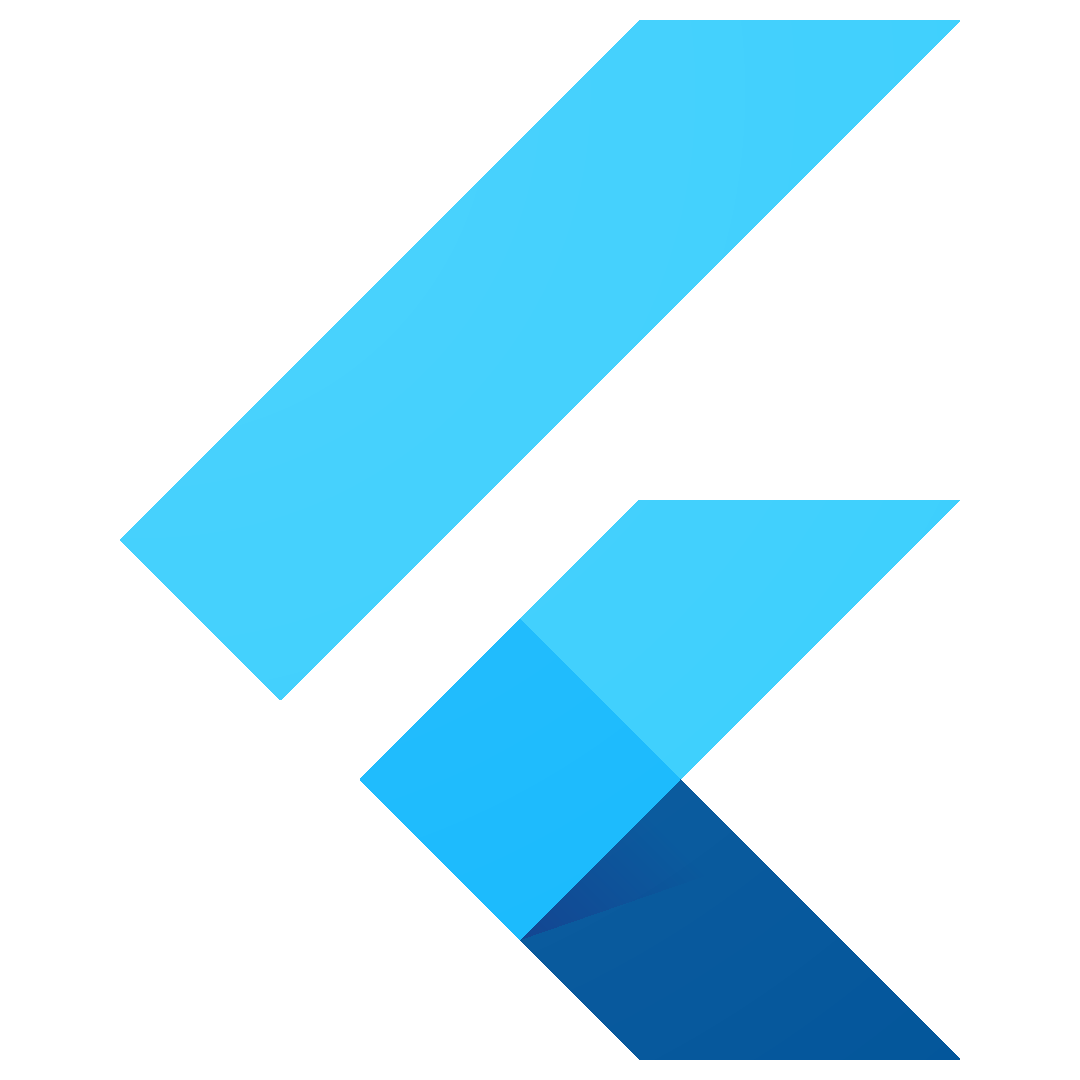
Authorization headers in HTTP
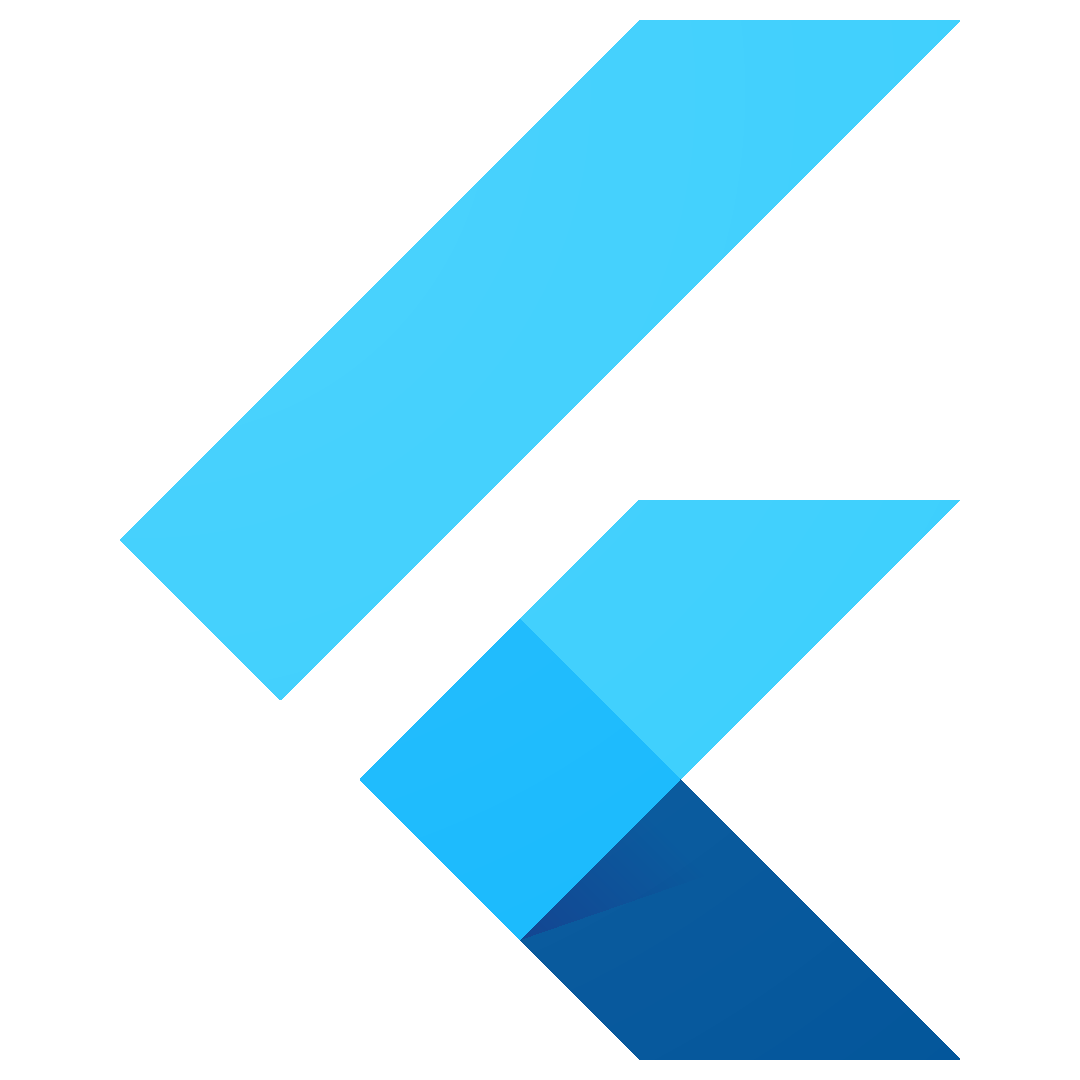
Send HTTP POST requests in your app.
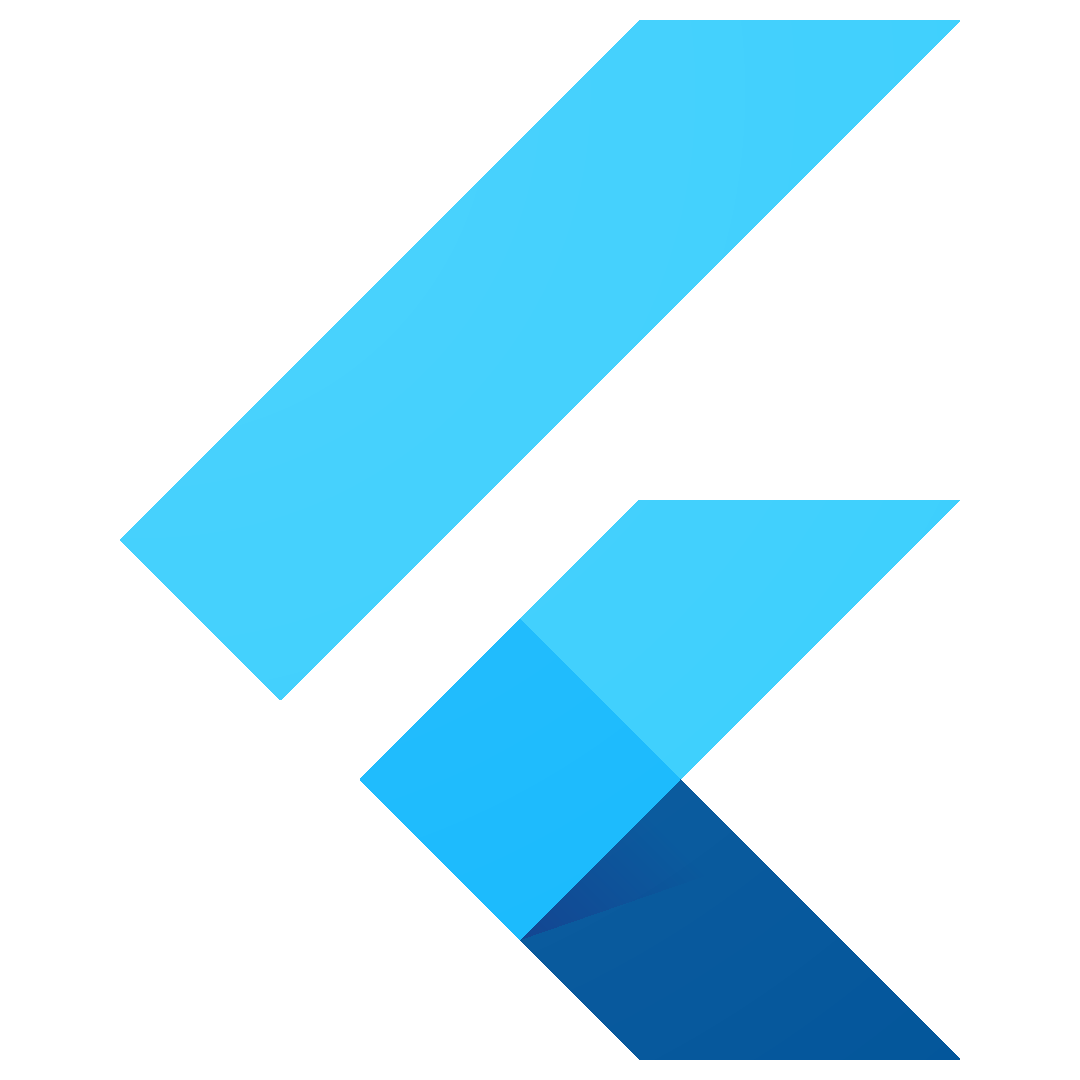
Send an HTTP put request.
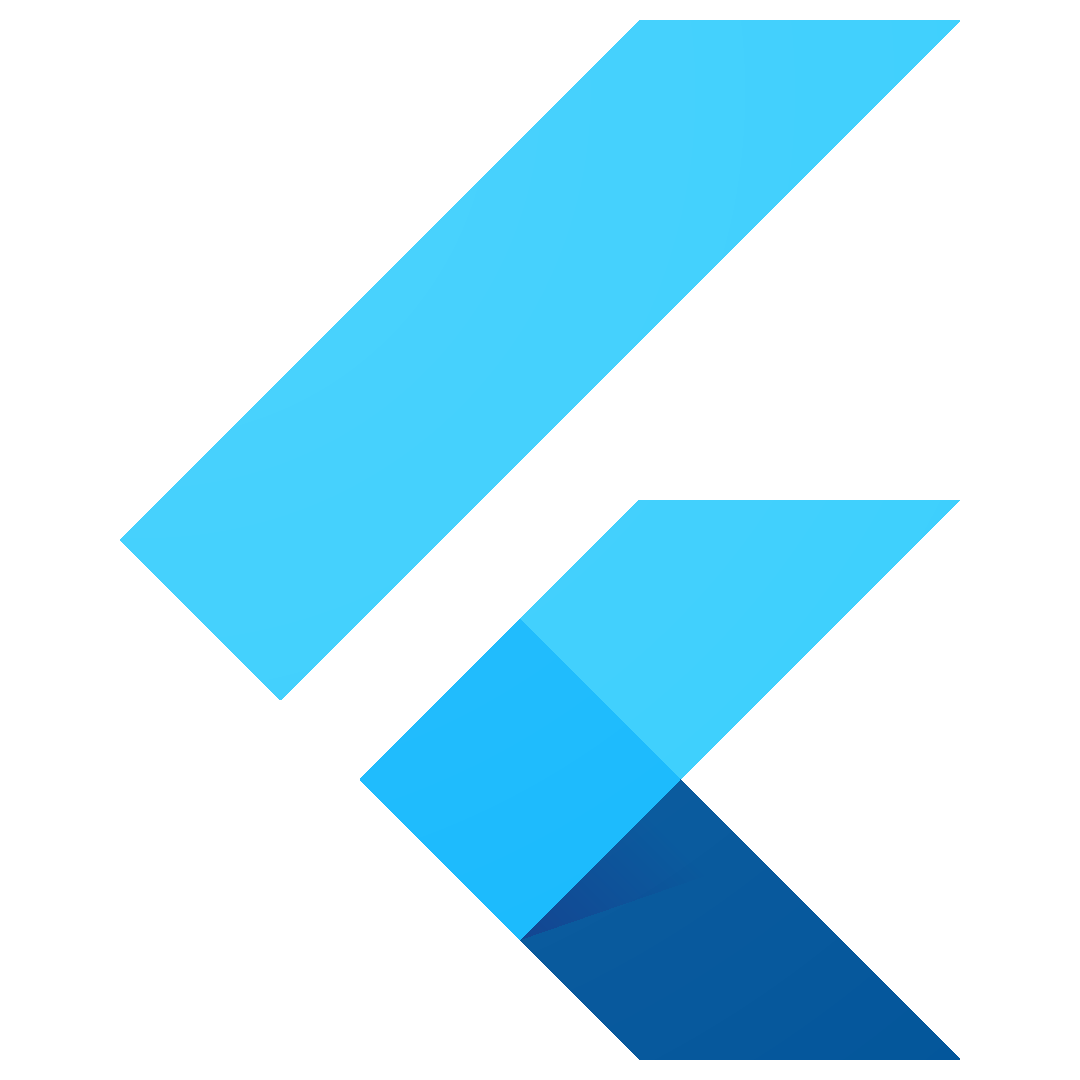
Send an HTTP delete request.
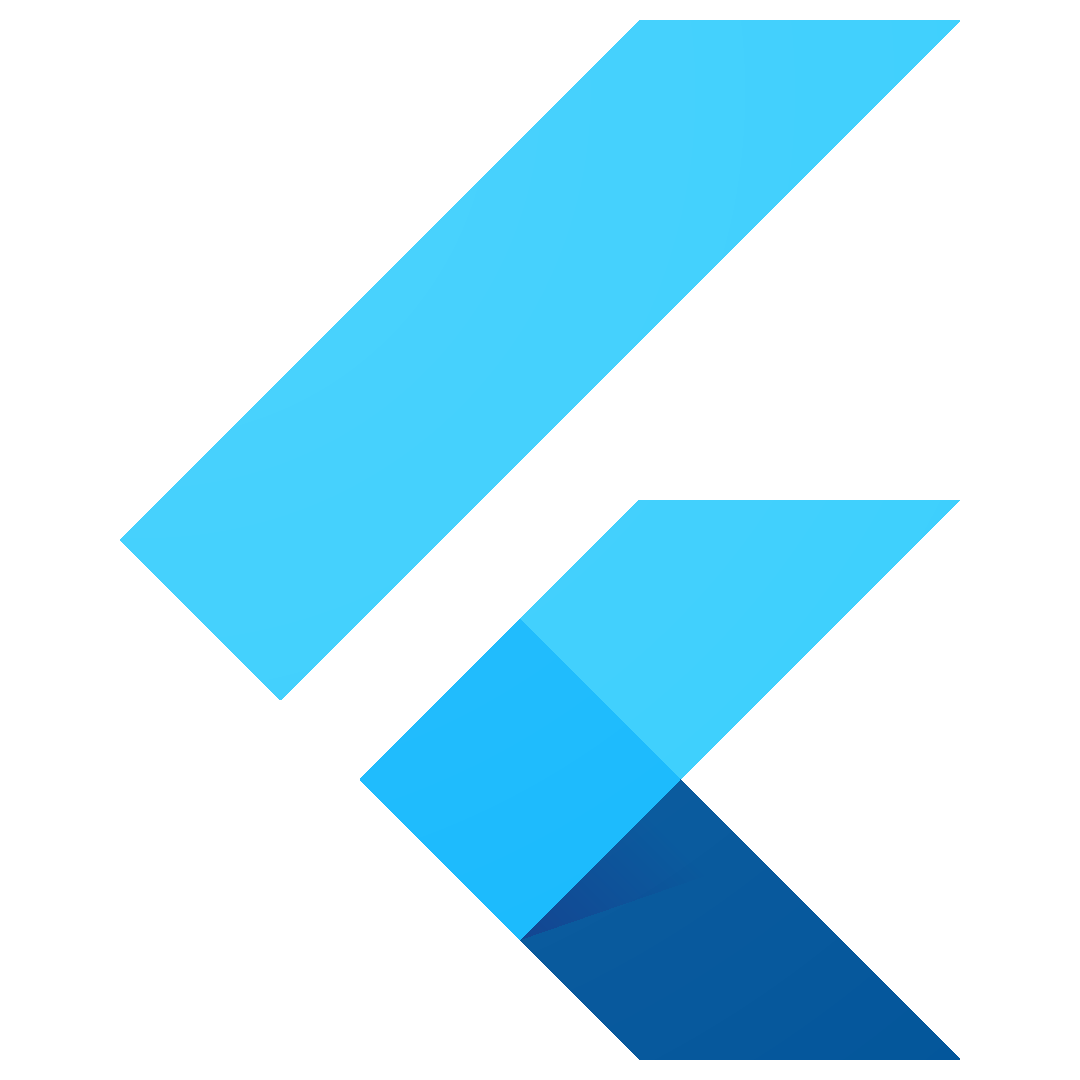
Connect to and communicate with a websocket.
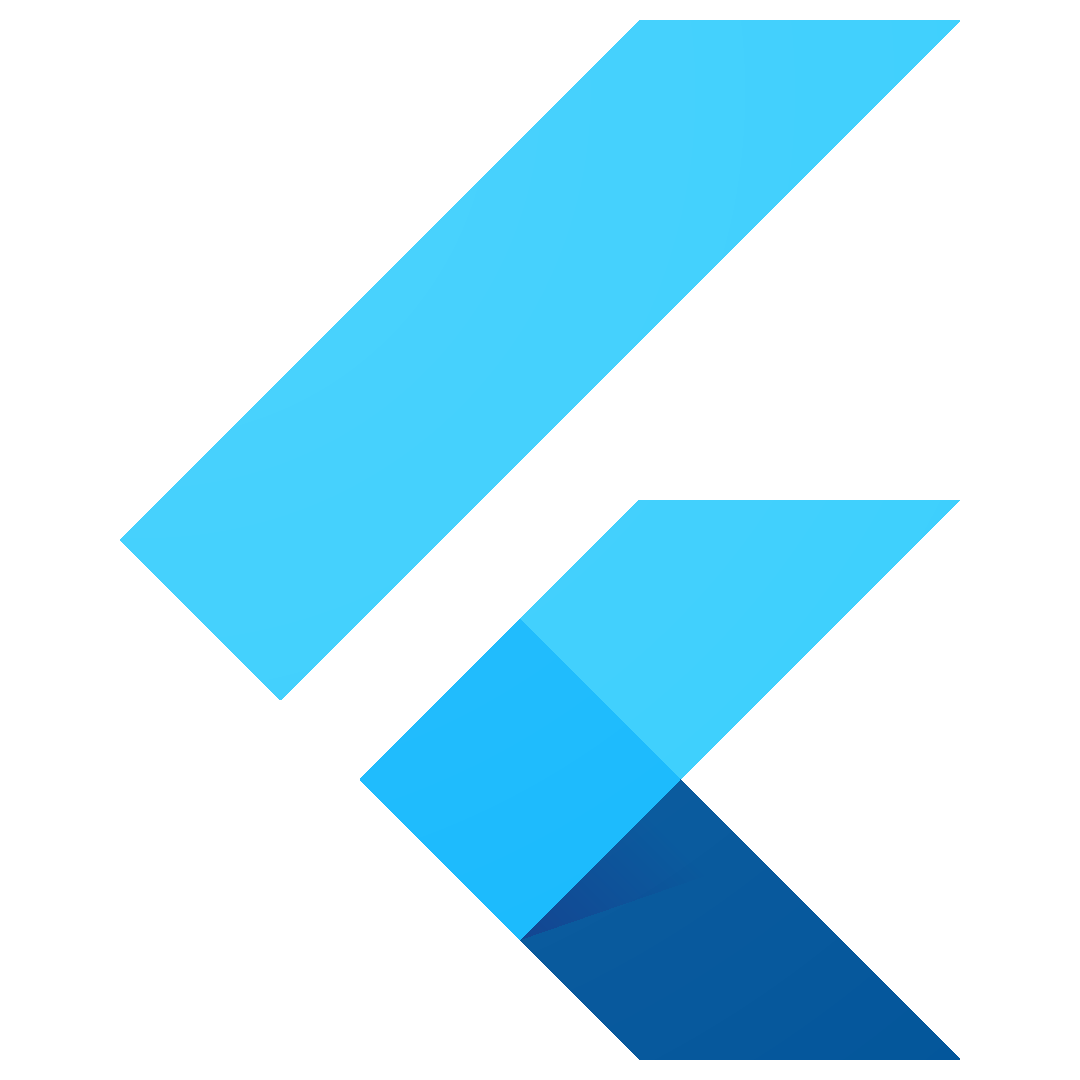
Learn to use Dart's Isolate objects
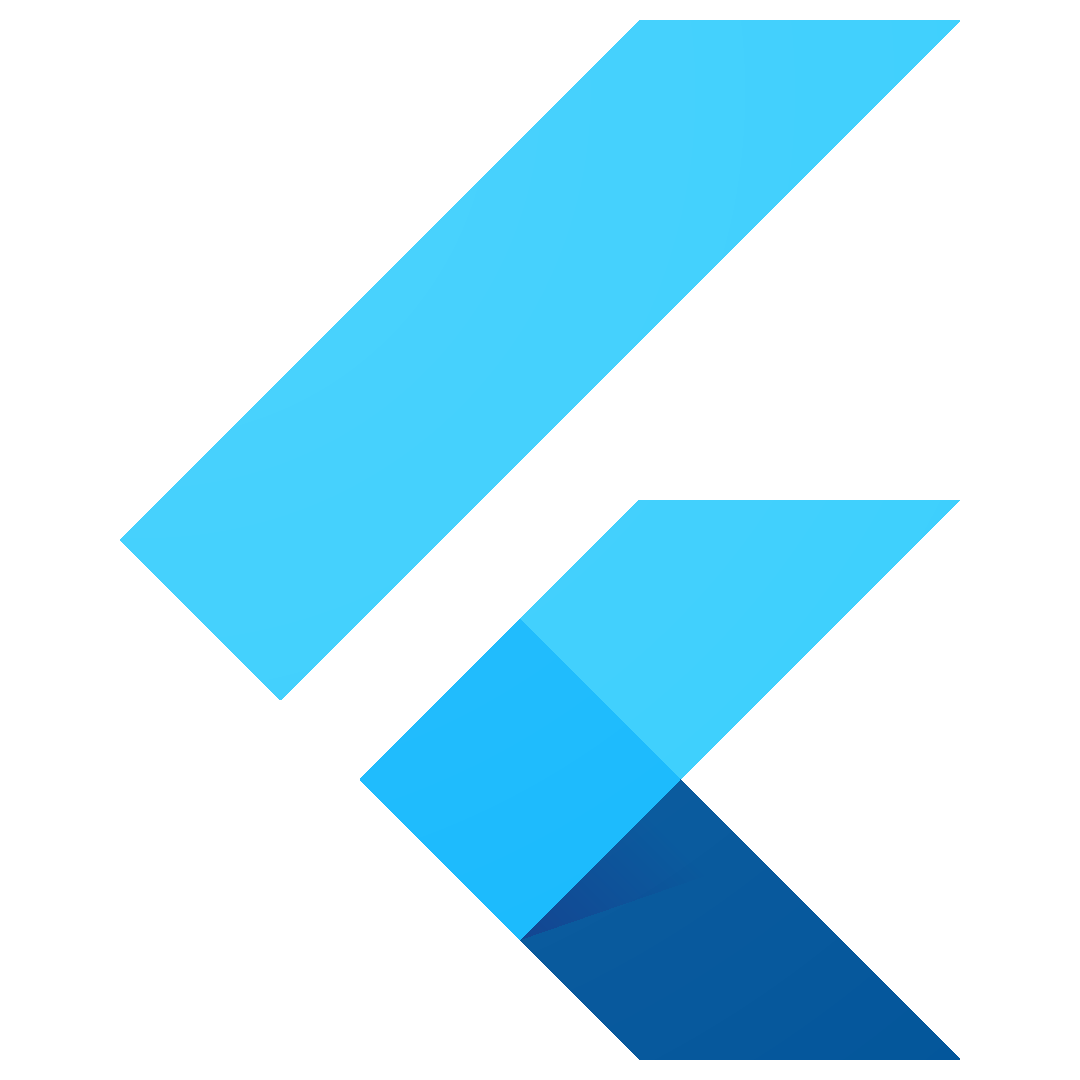
Use the sqflite package.
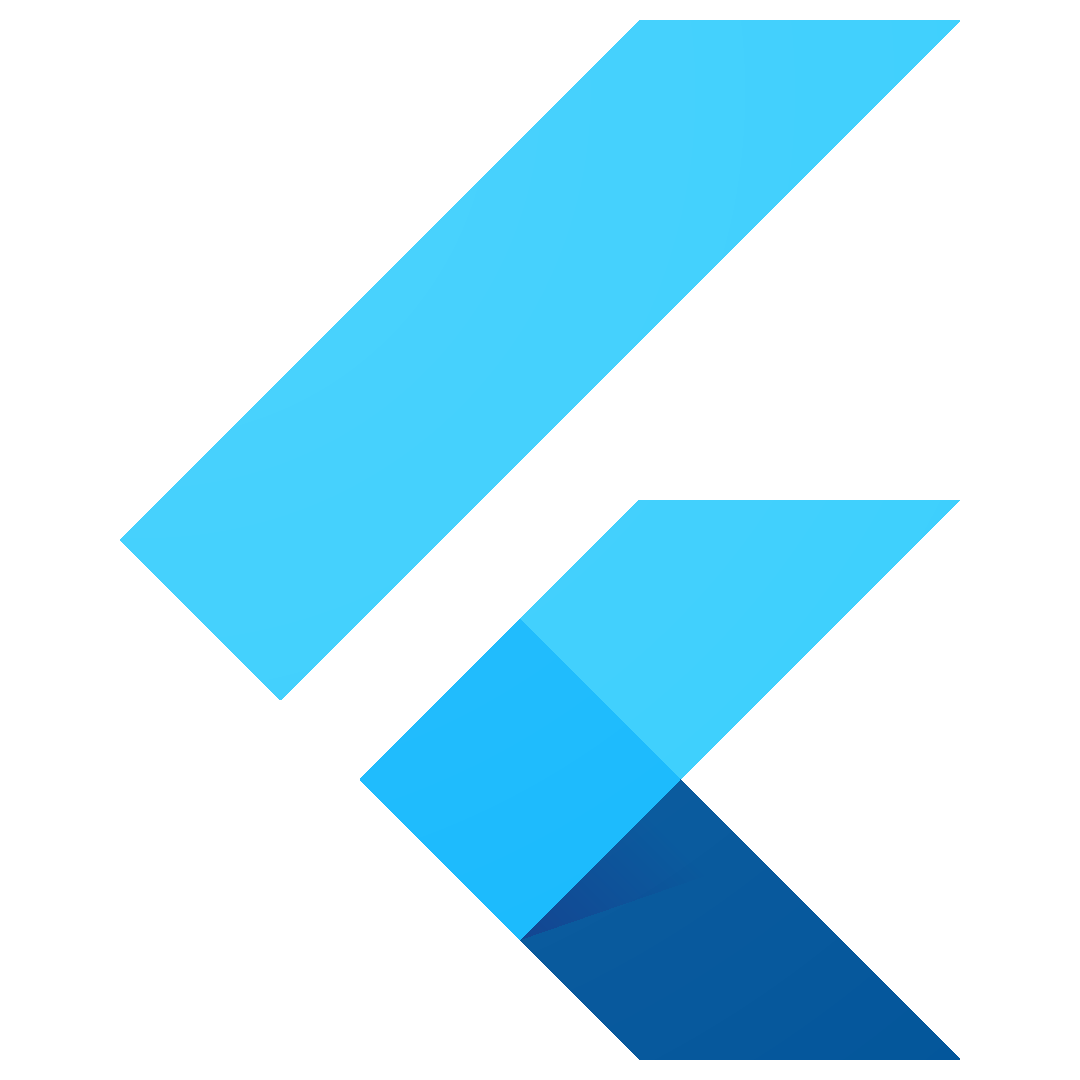
Use the dart:io library and path_provider plugin to save files to disk.
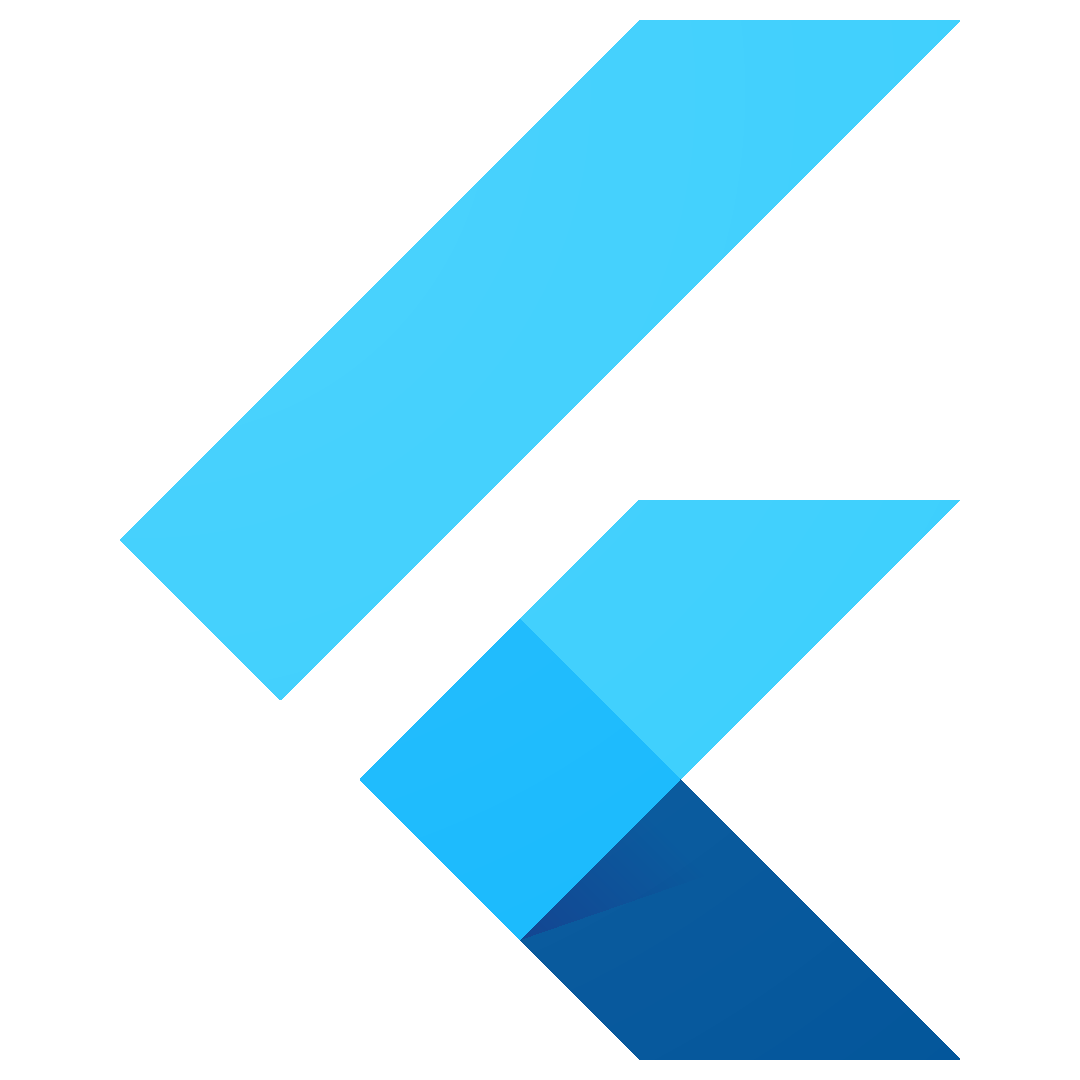
Persist data with shared_preferences
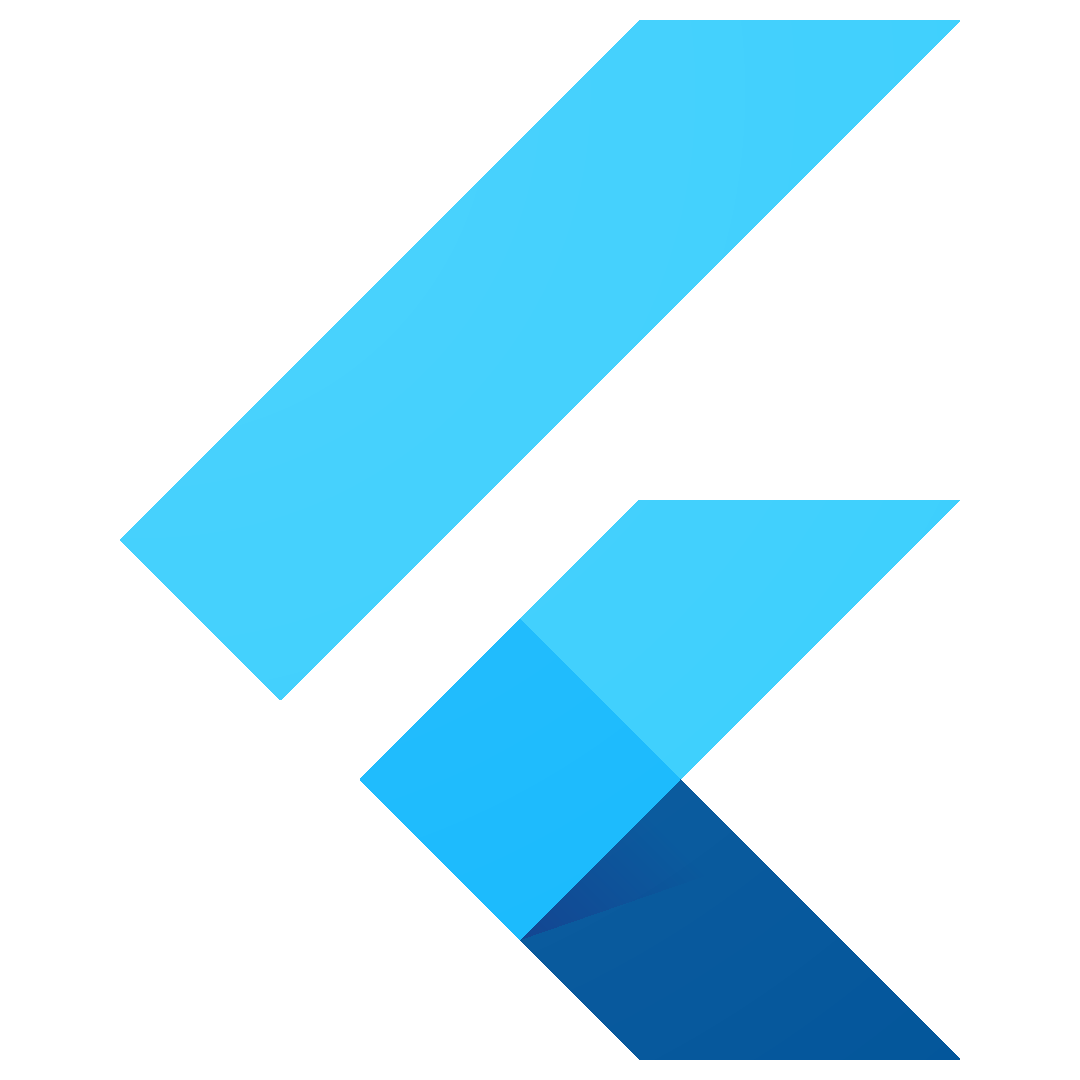
Play videos stored on the file system, as an asset, or from the internet.
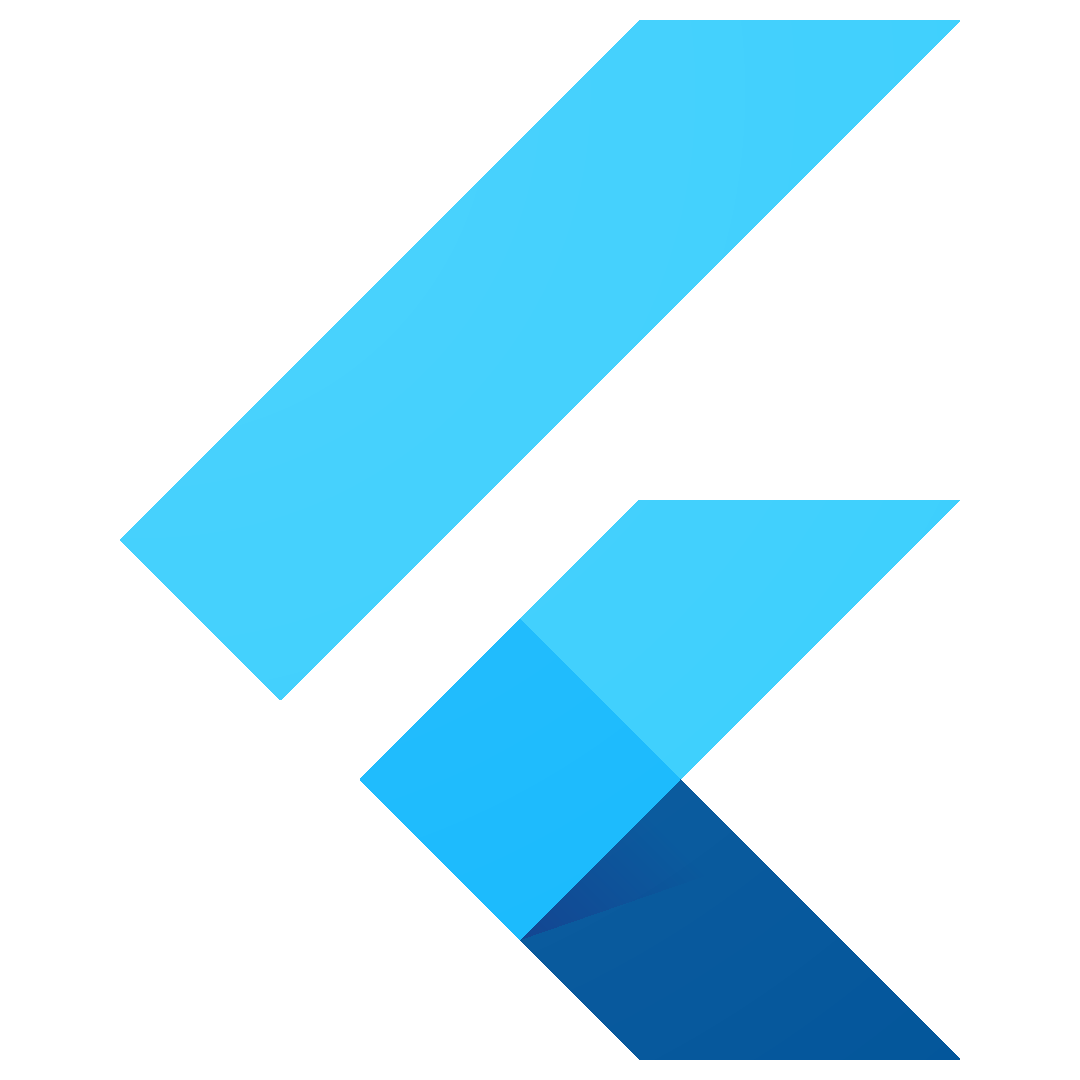
Learn to use a devices camera.
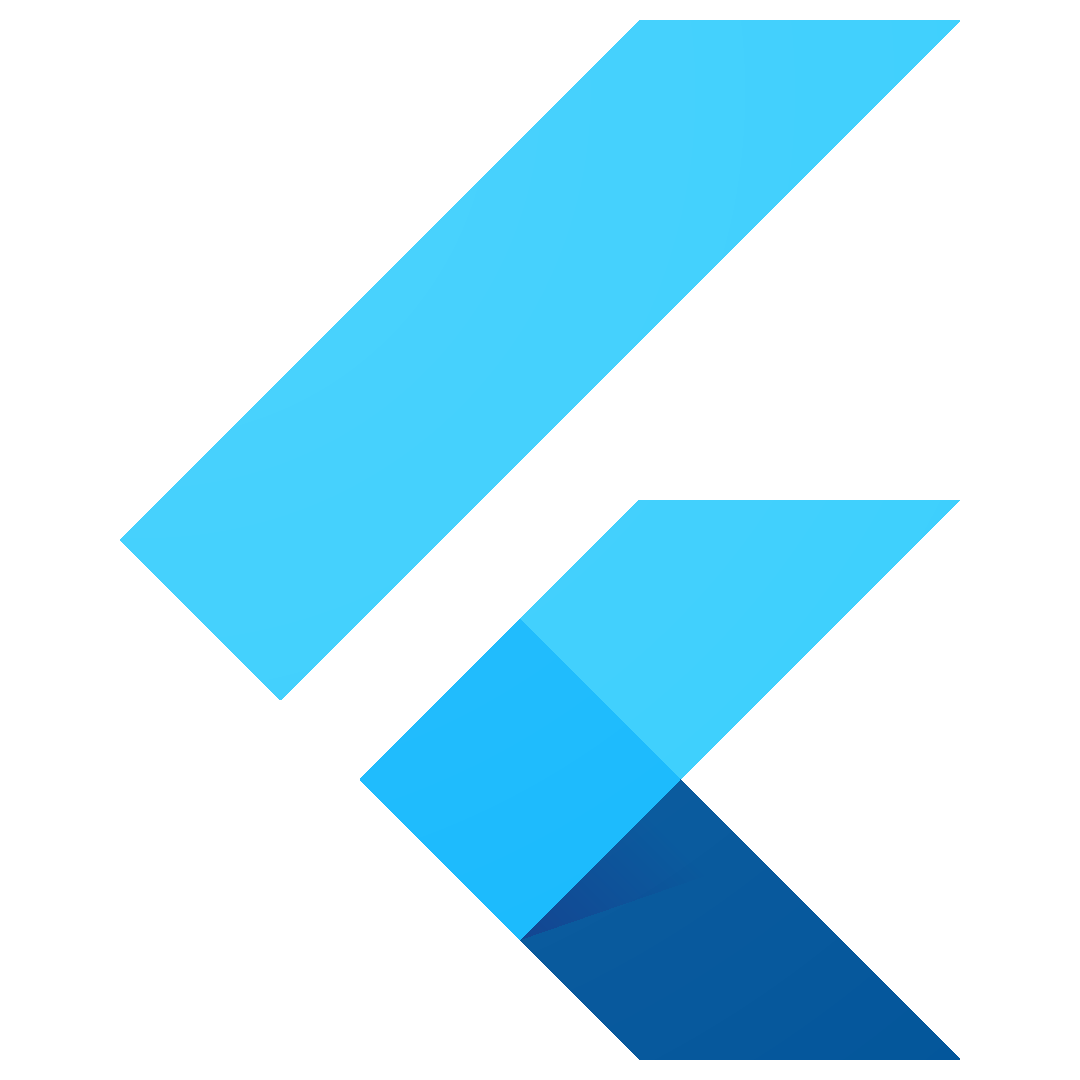
Report errors to Sentry crash reporting.
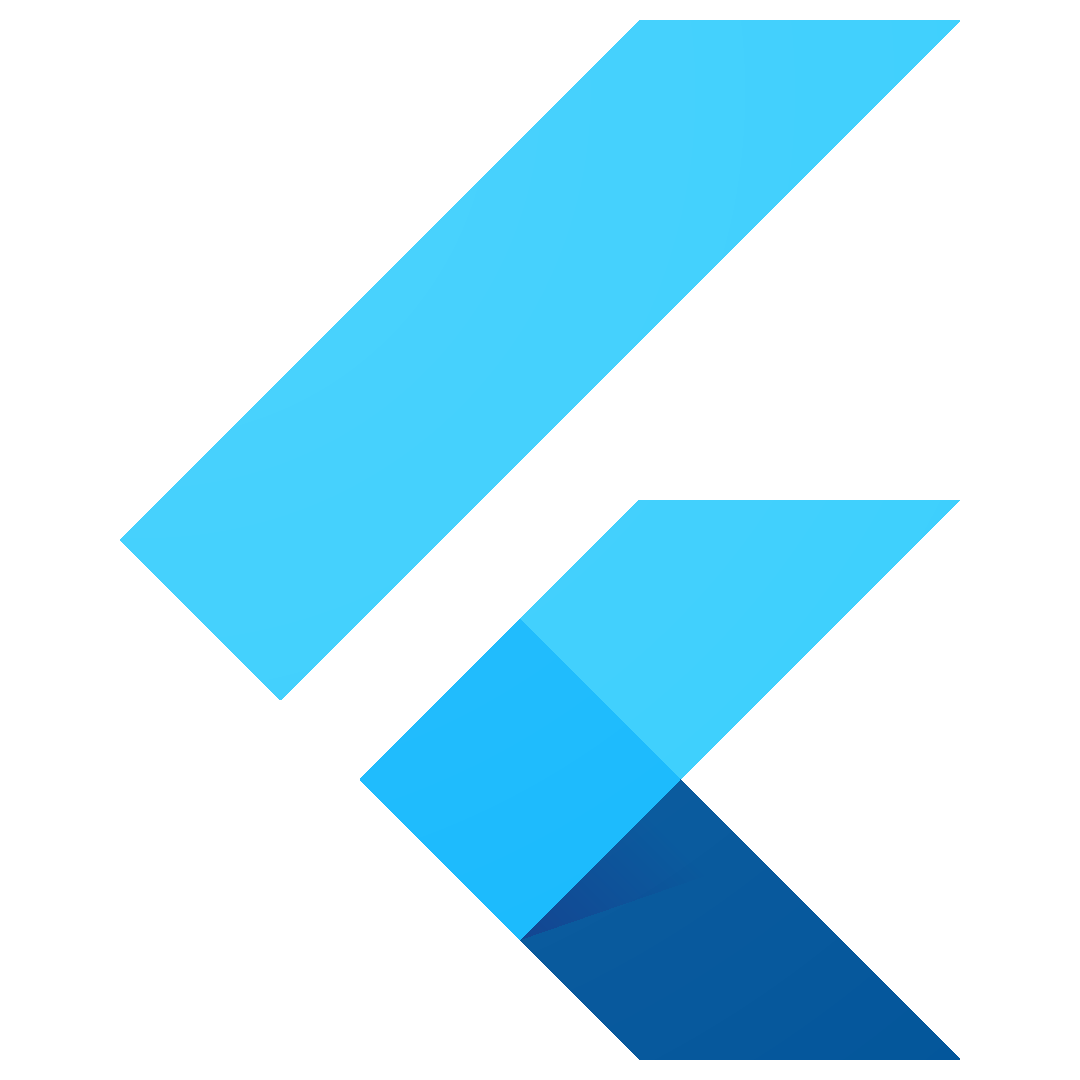
Write a test that records a performance timeline.
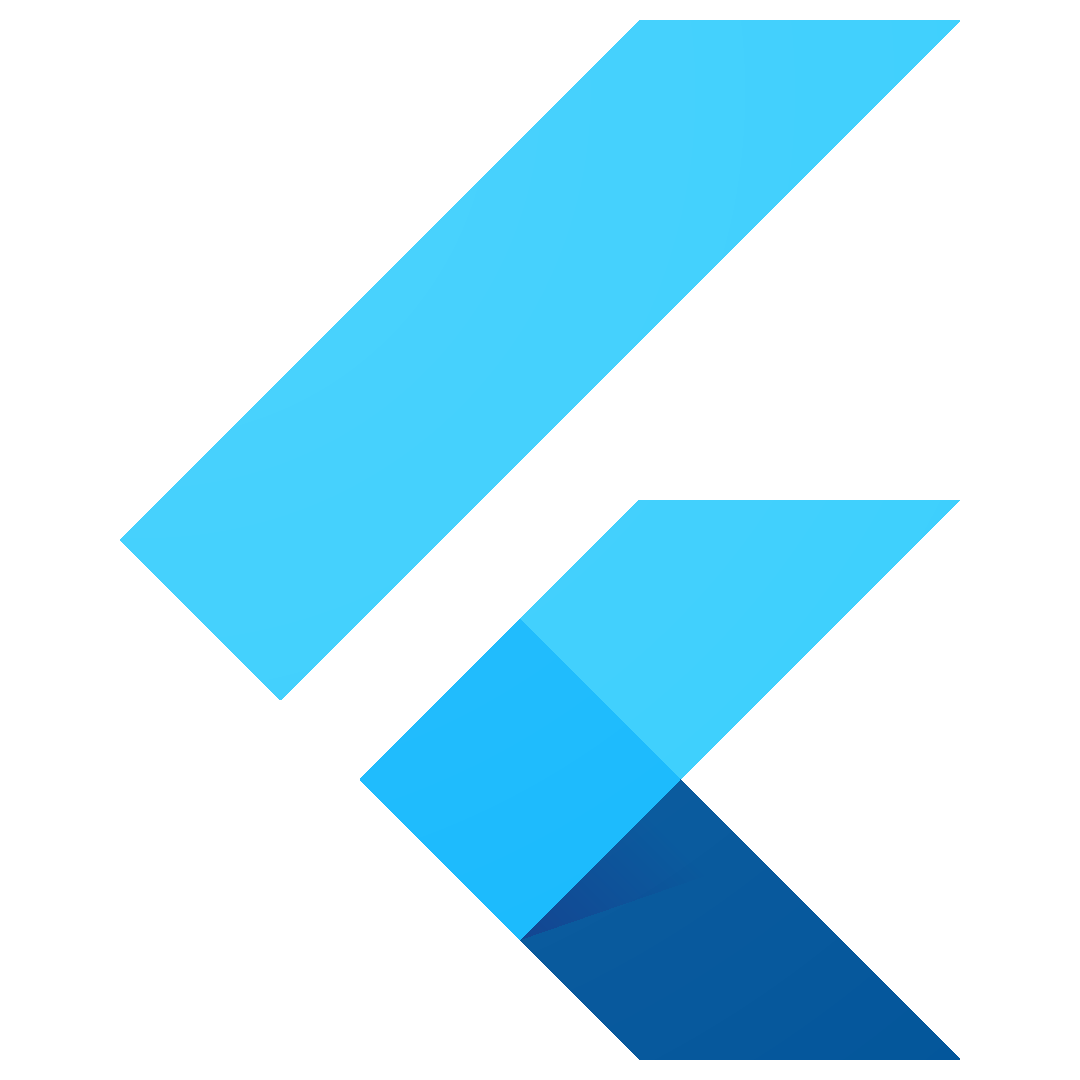
An introduction to writing unit tests.
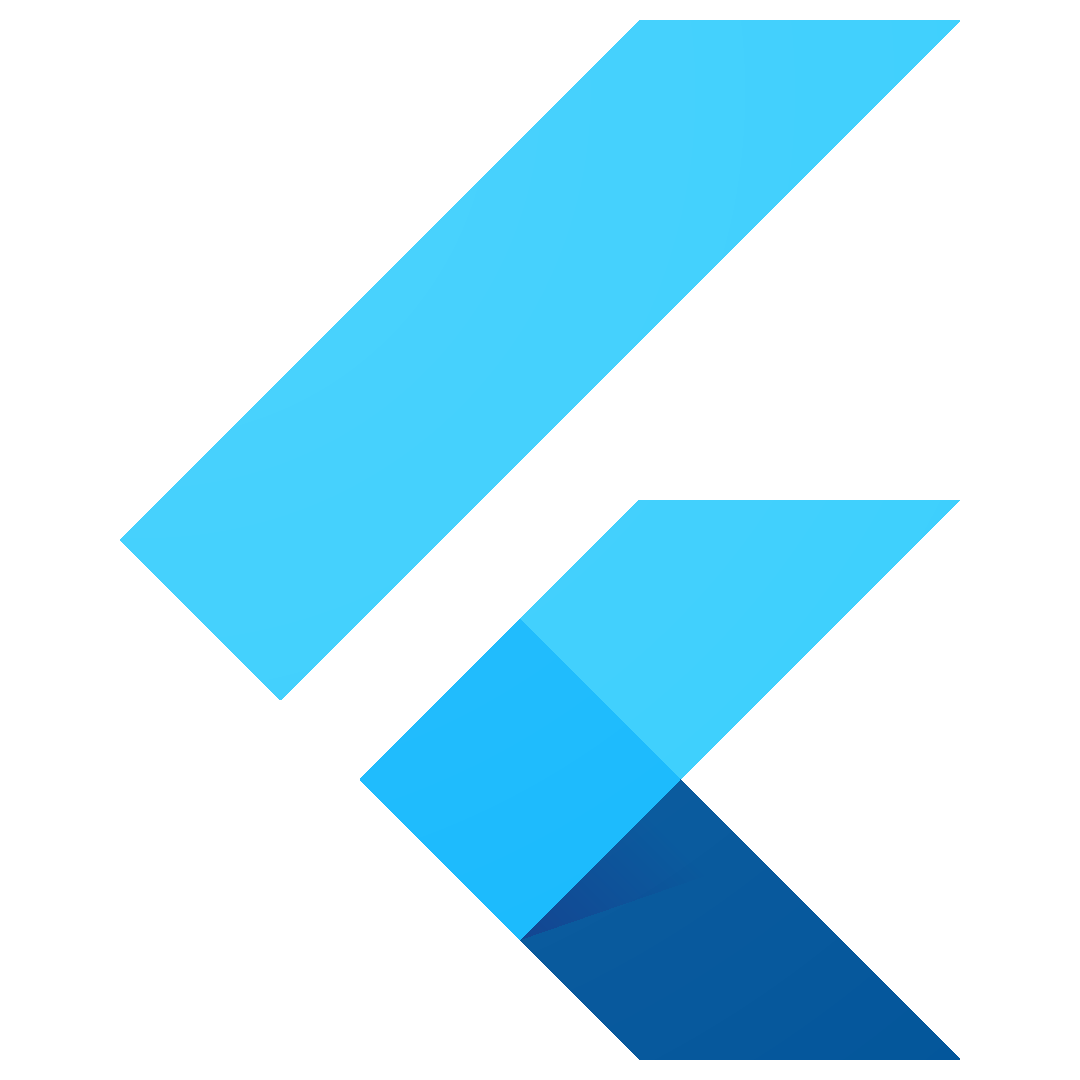
An introduction to writing widget tests.
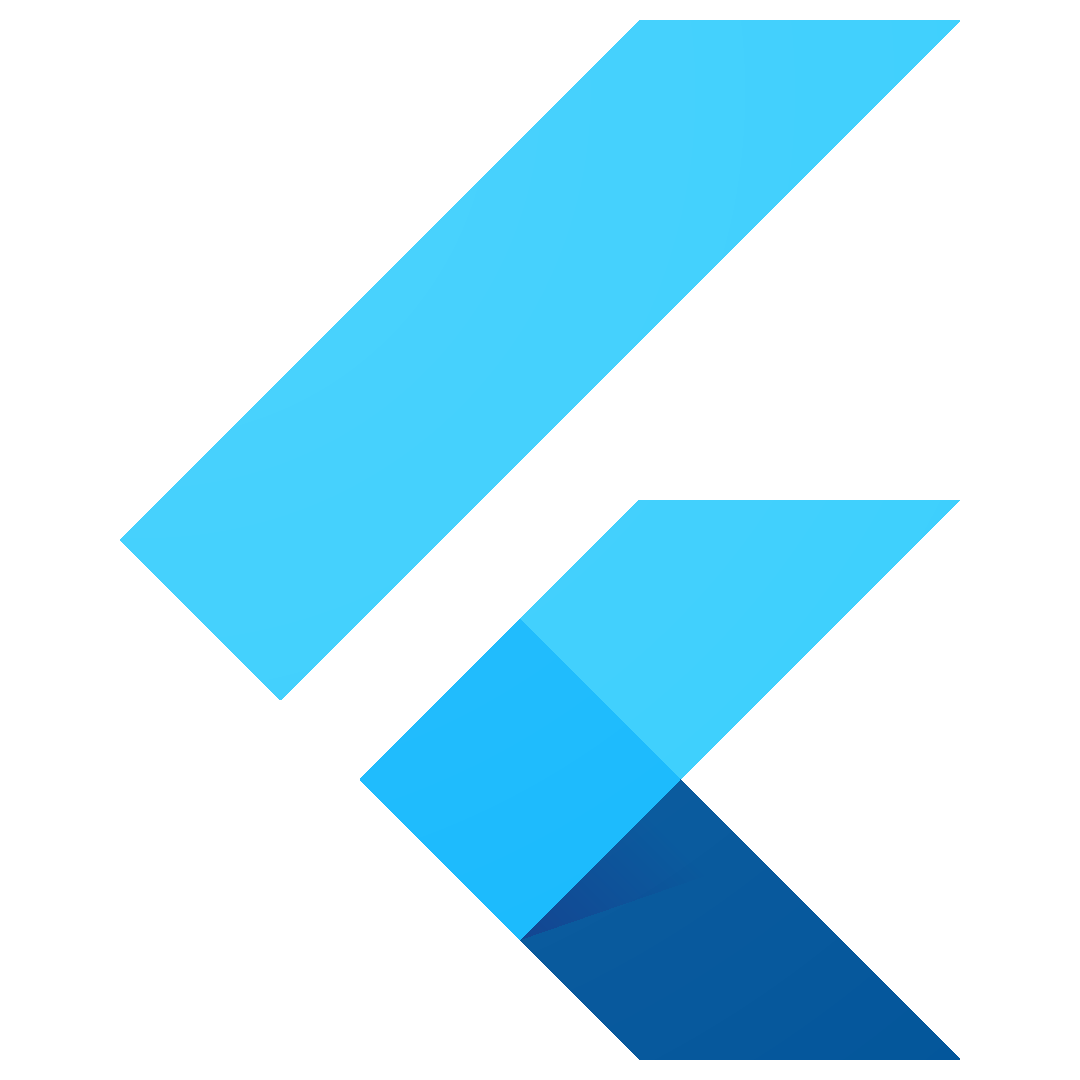
The basics of mocking with the Mockito package.
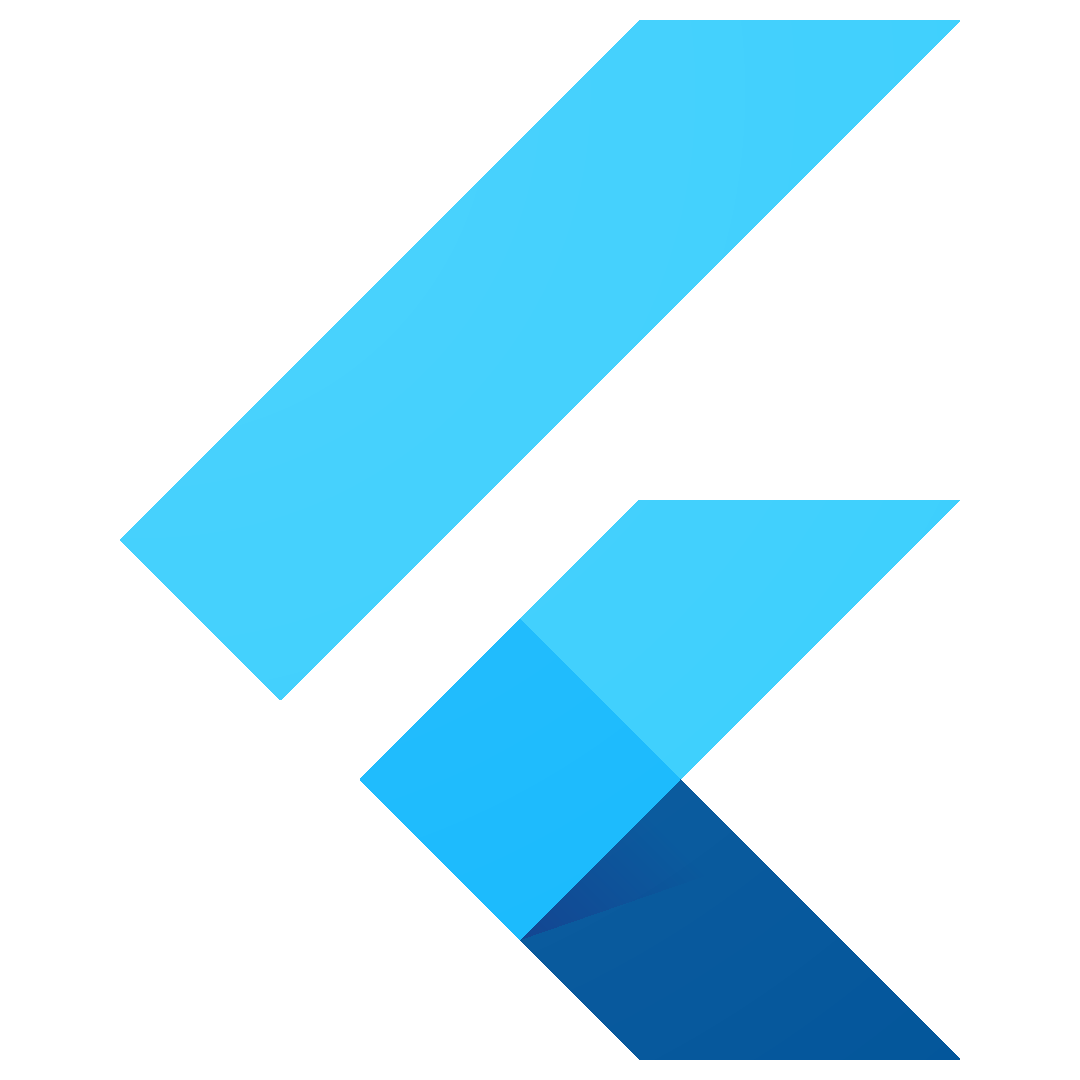
This recipe looks at the 'find' constant provided by the flutter_test package.
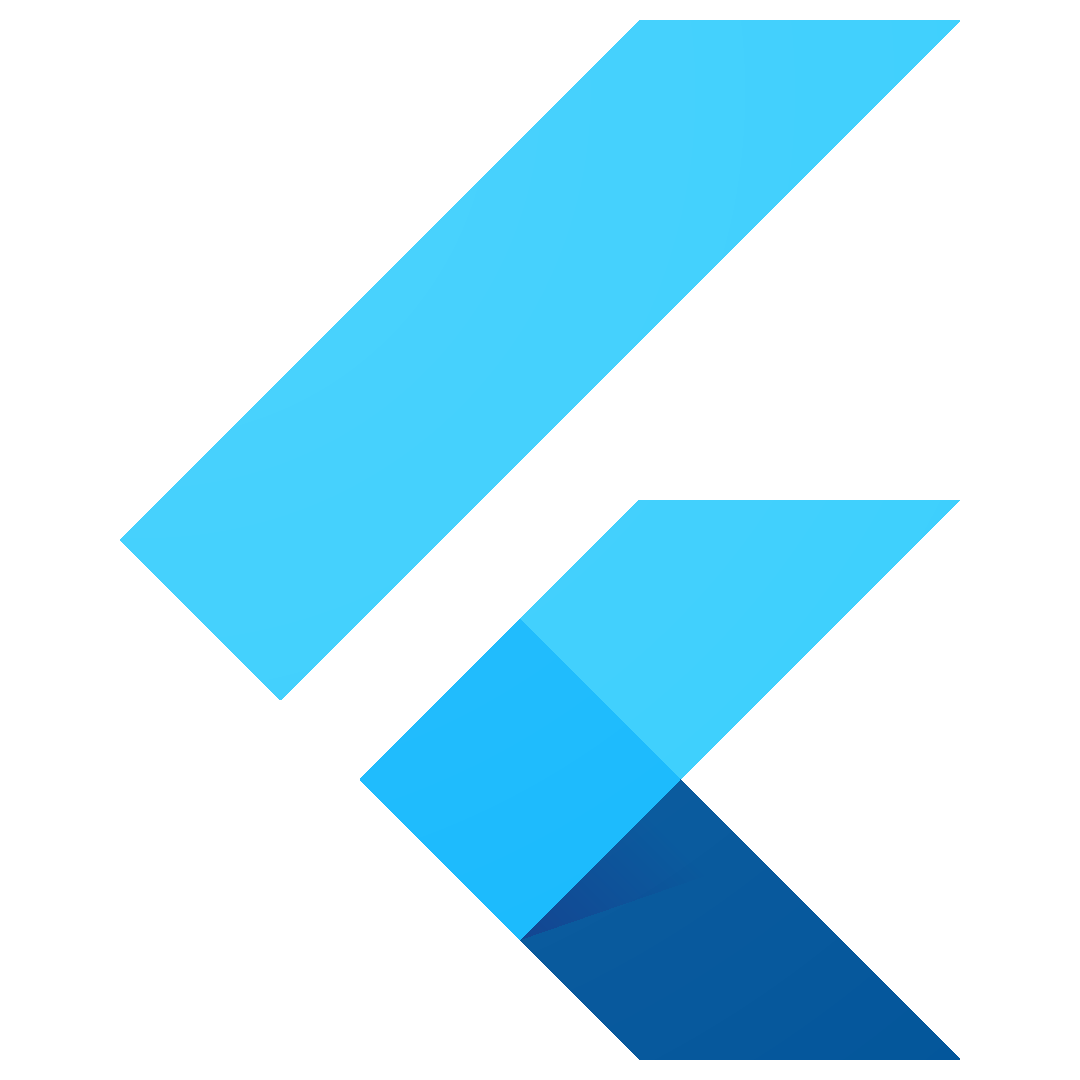
Learn how to scroll in widget tests.
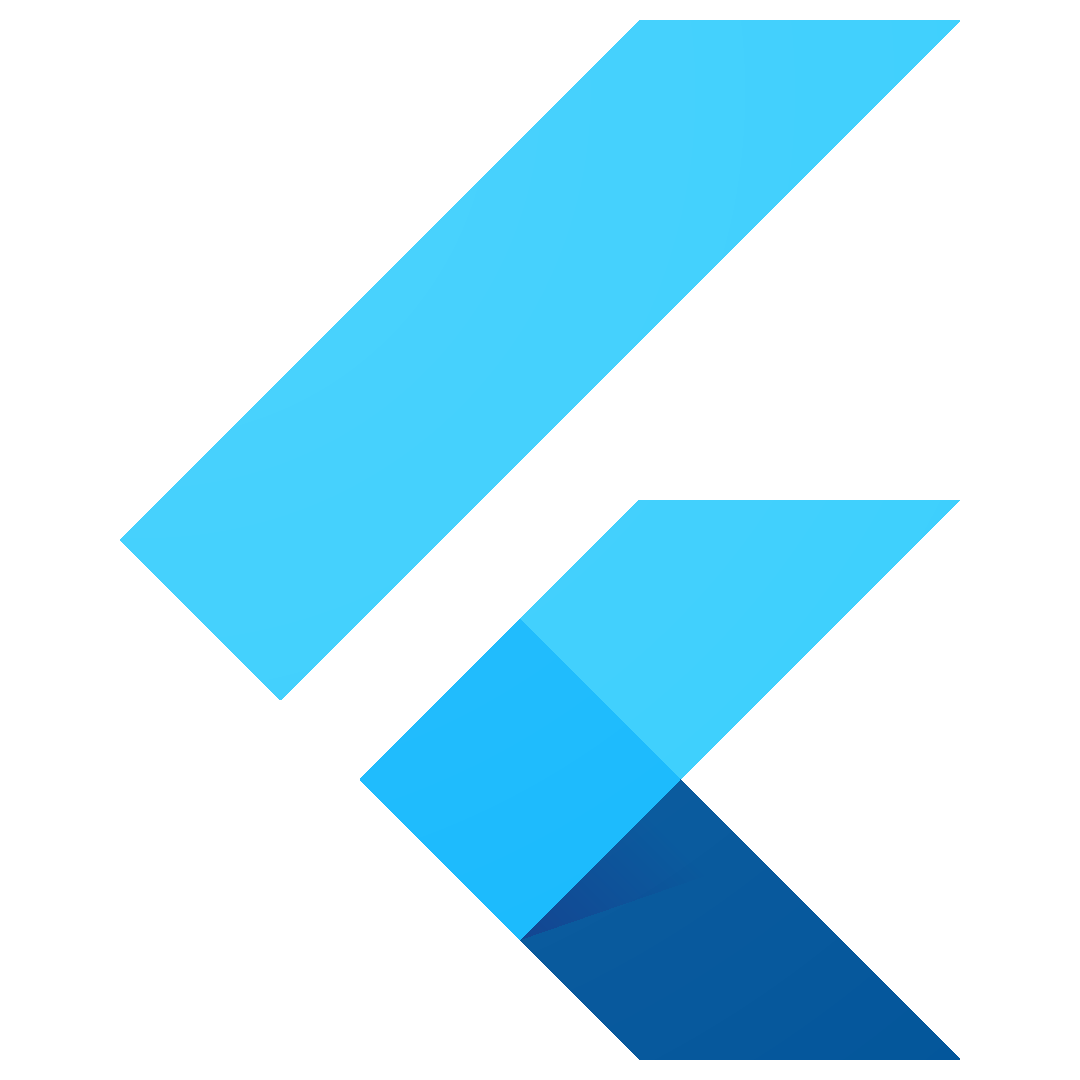
Learn how to check app orientation in widget tests.
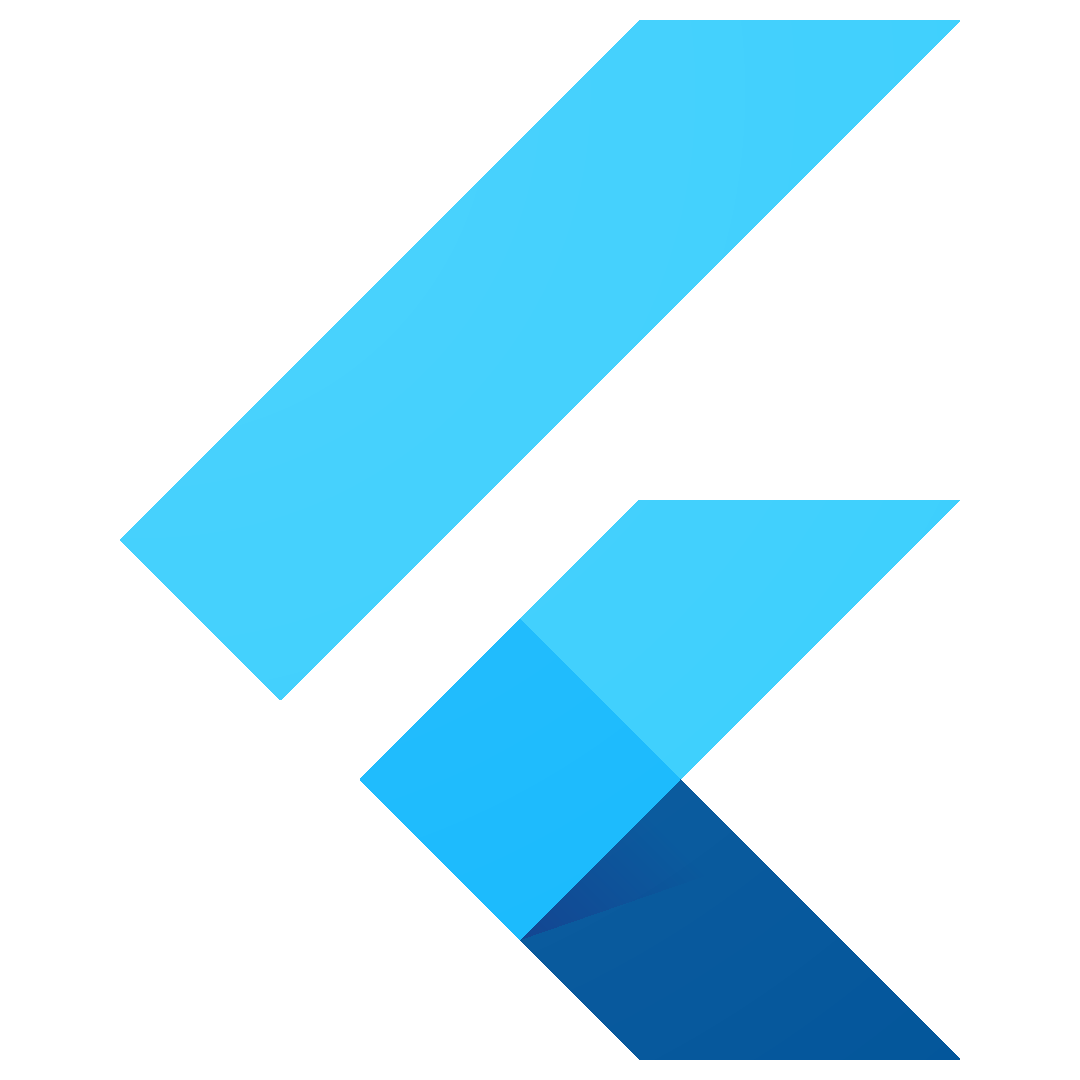
Interact with widgets in widget tests.
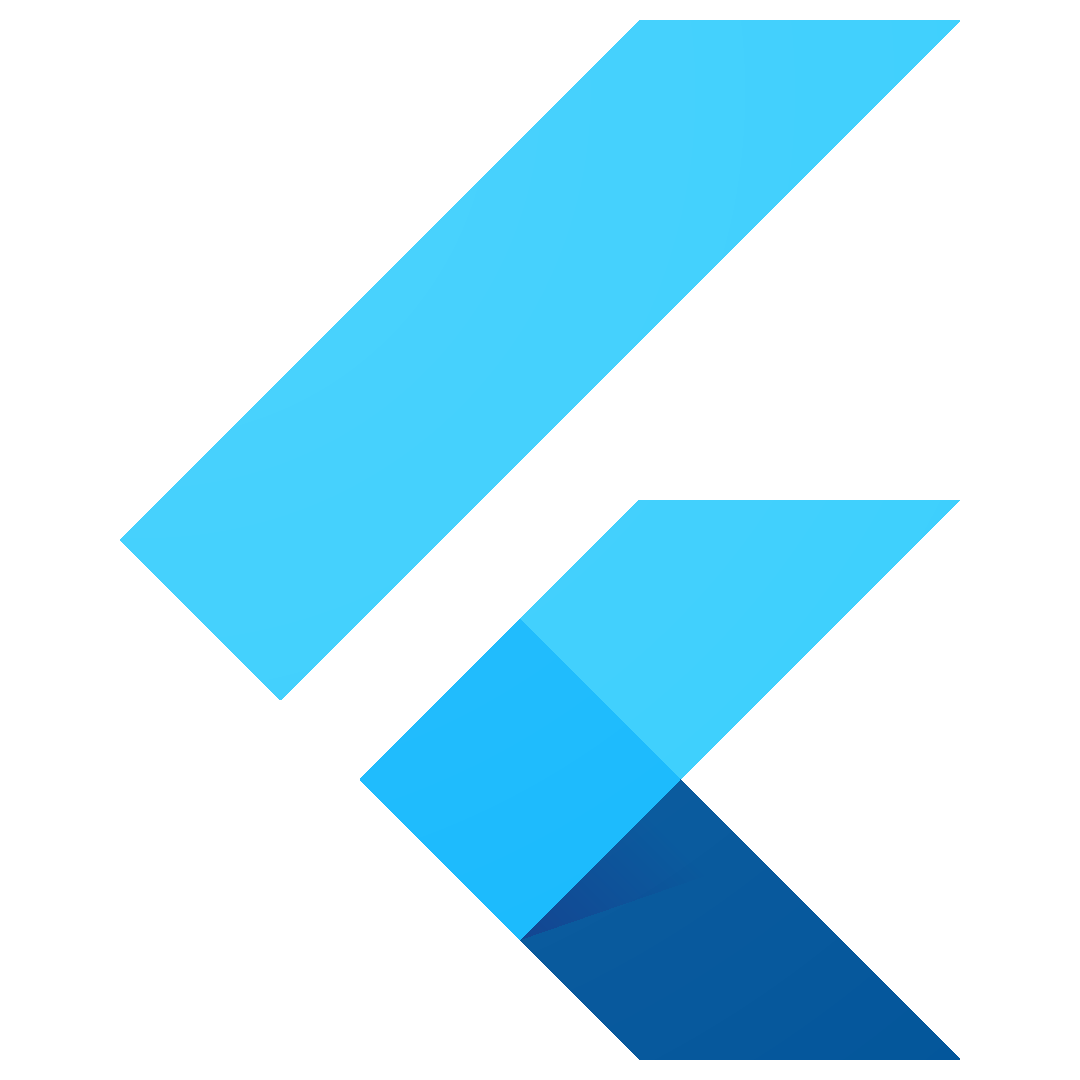
Save complex application data to a user's device with SQL.
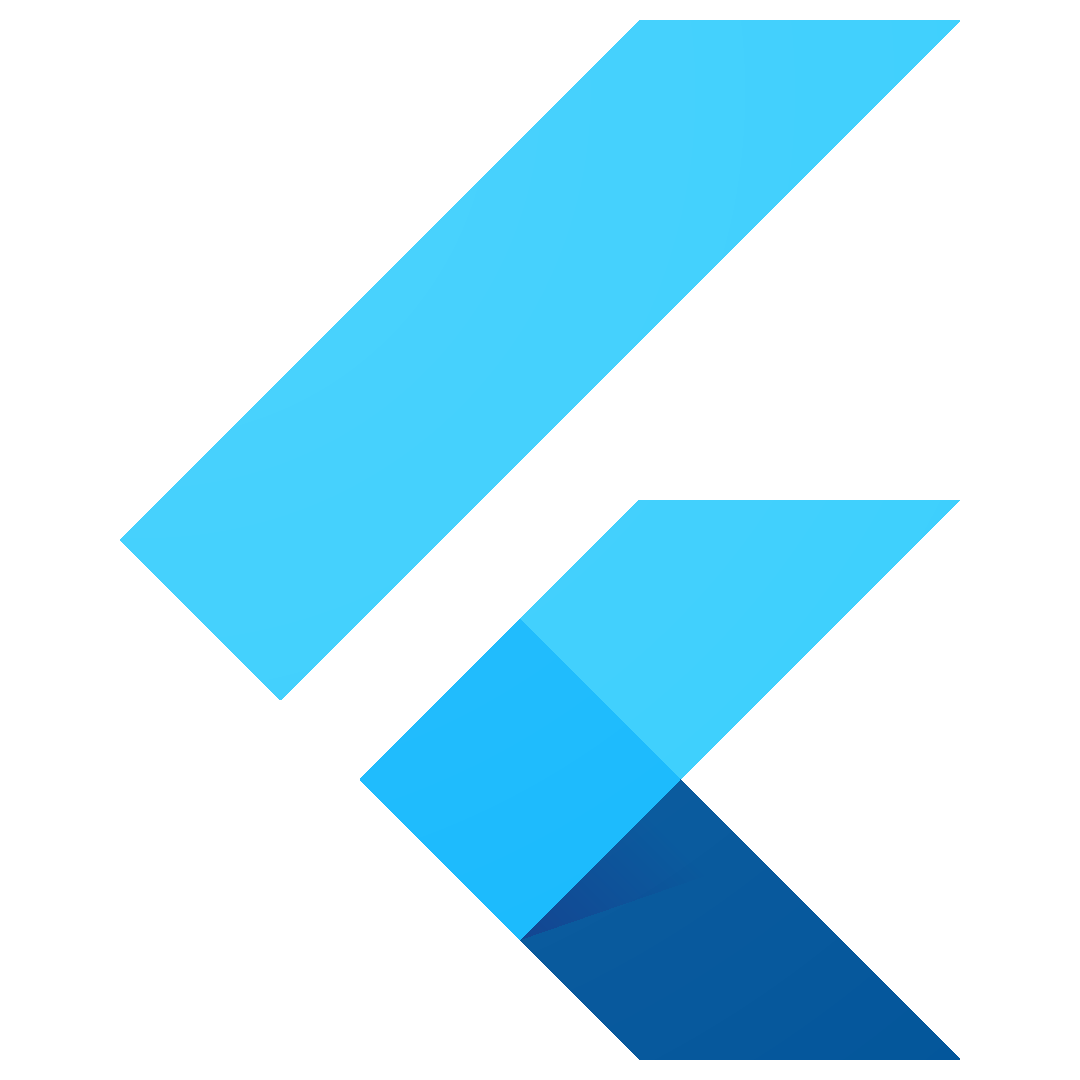
Improve error handling across classes with Result objects.
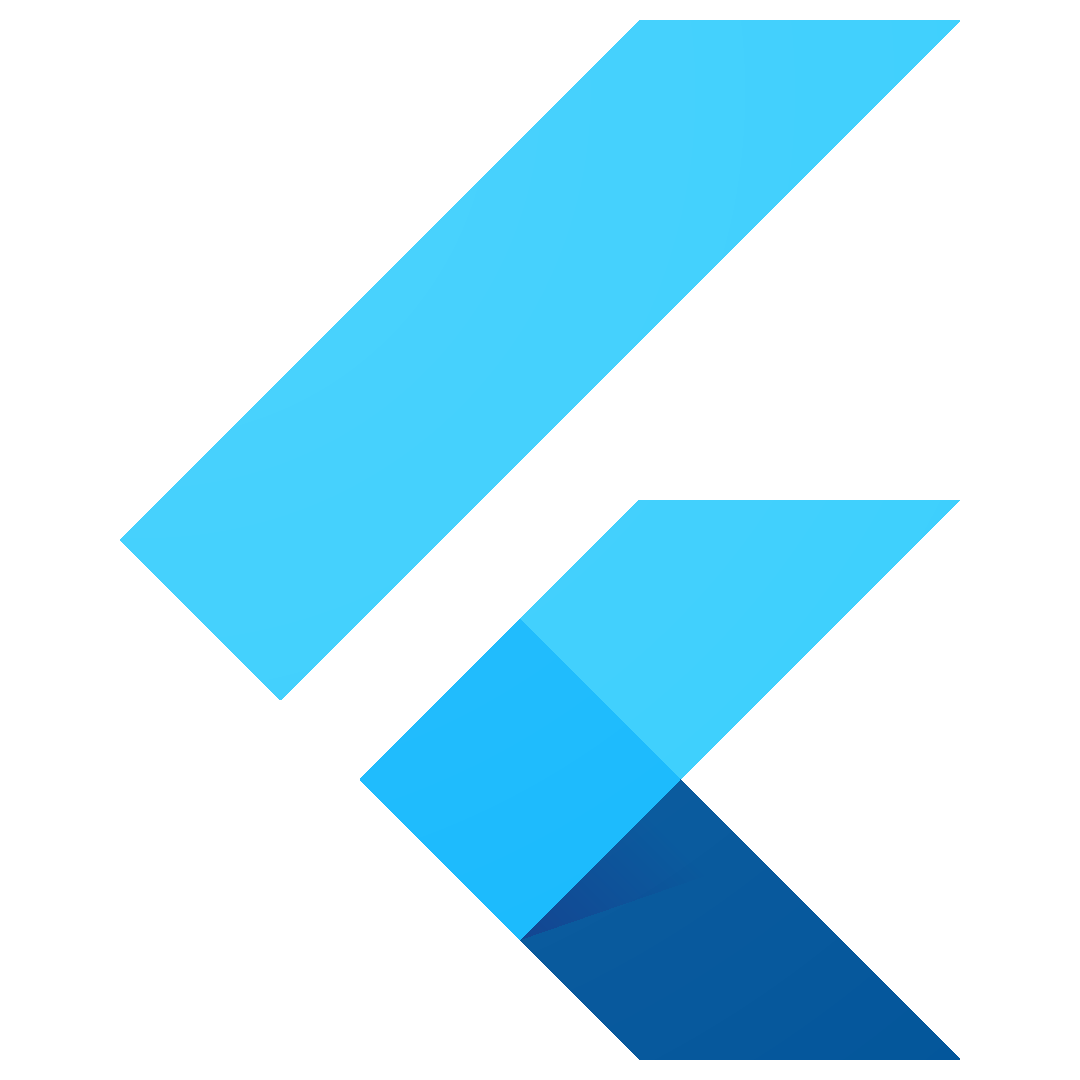
Improve the perception of responsiveness of an application by implementing optimistic state.
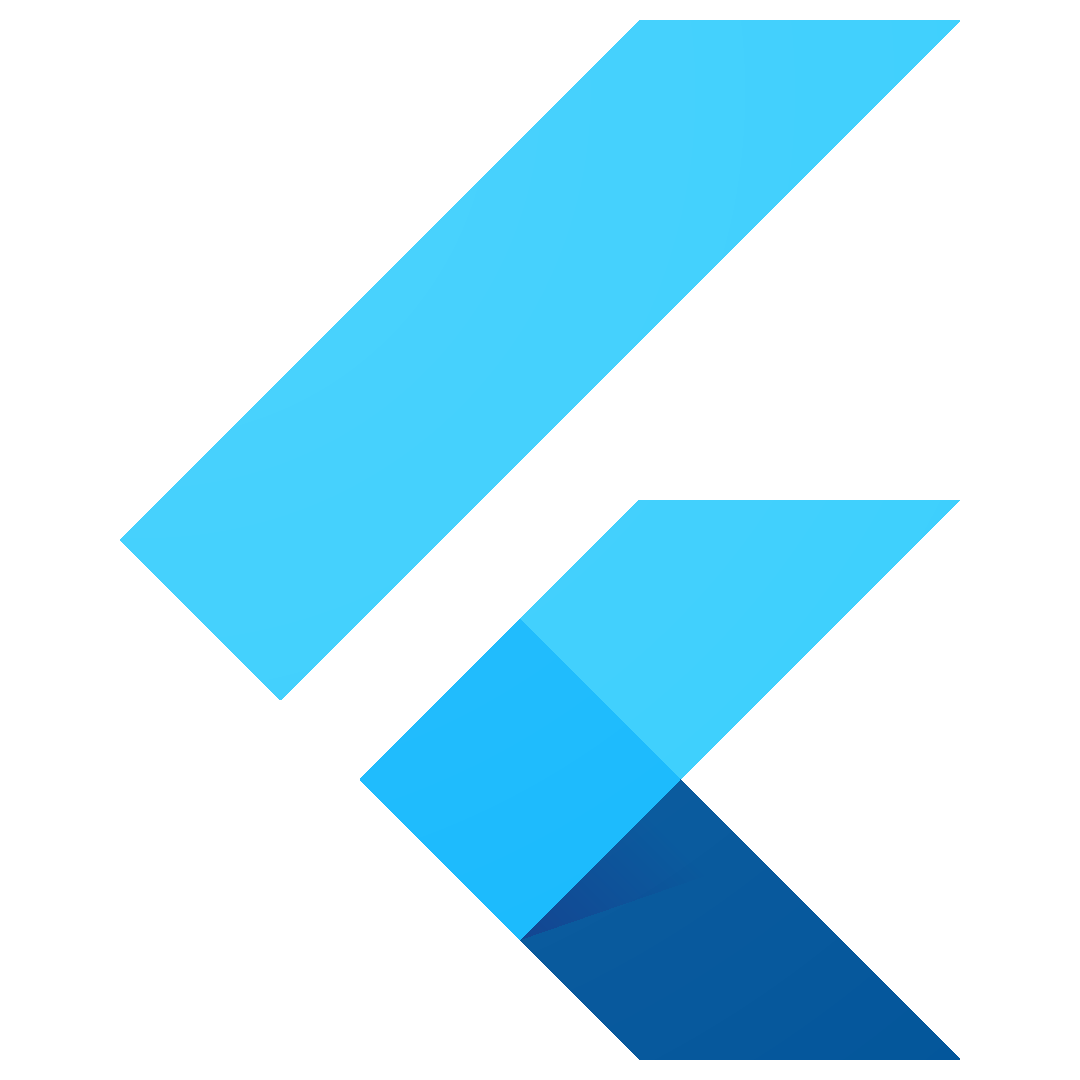
Implement offline-first support for one feature in an application.
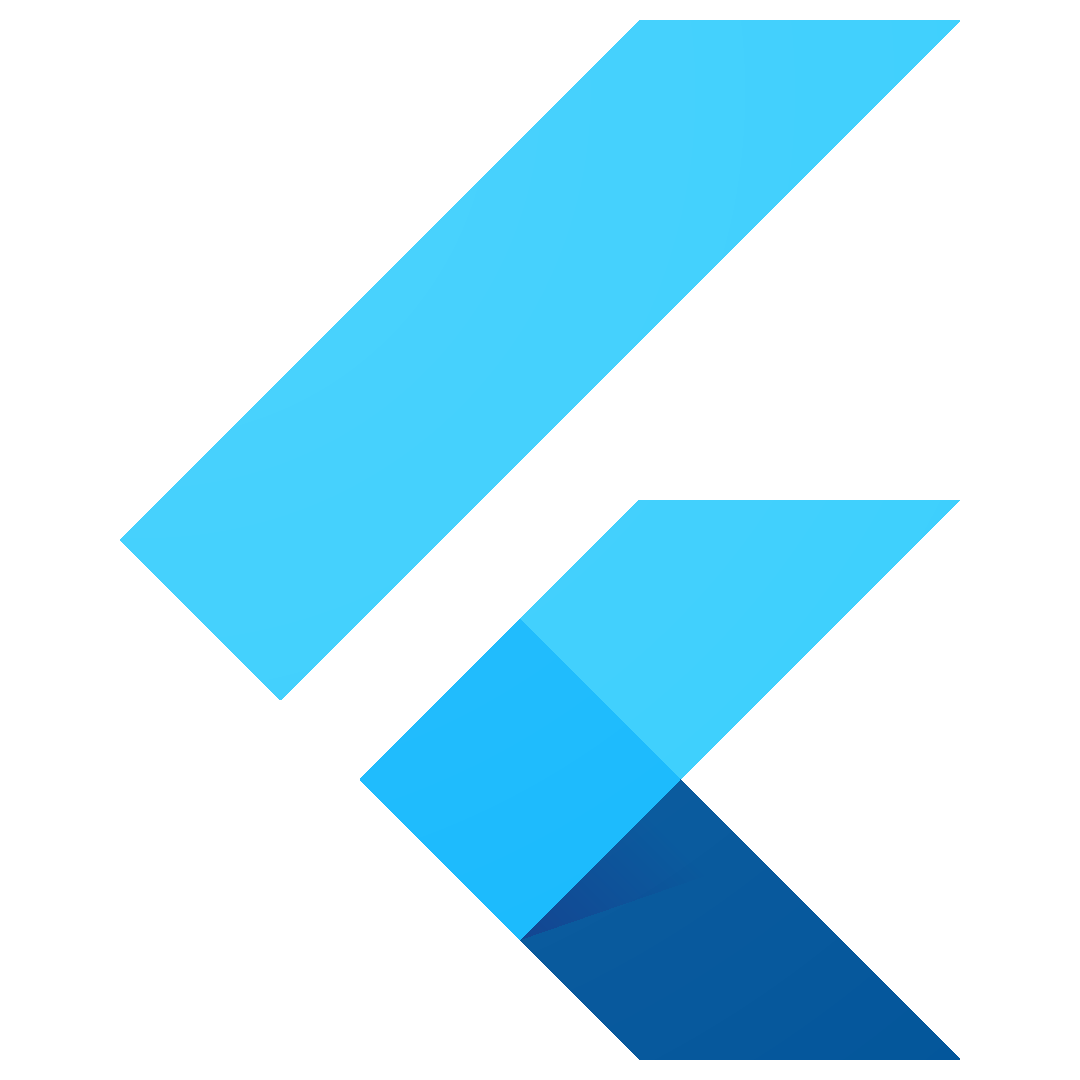
Save application data to a user's on-device key-value store.
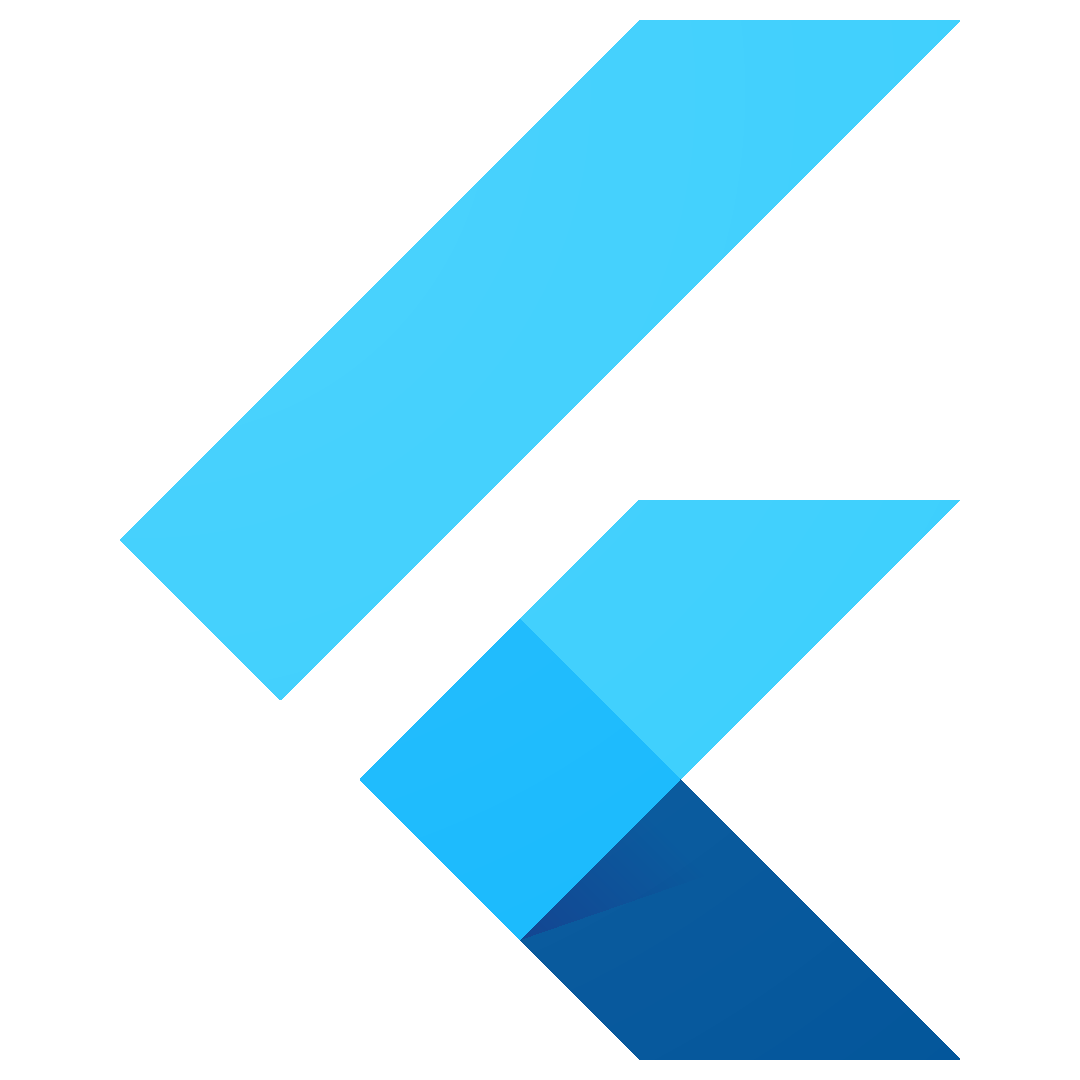
Simplify view model logic by implementing a Command class.
Recommended approaches for adding Flutter to existing apps.
A Flutter sample app that combines a native iOS UIViewController with a full-screen Flutter view.
This sample text editor showcases the use of TextEditingDeltas and a DeltaTextInputClient to expand and contract styled ranges of text.
An iOS sample app that shows which fruits and vegetables are currently in season. It showcases Flutter's Cupertino package.
Demonstrates how to transform images' color scales and formats.
Demonstrates how to use long-lived isolates.
Demonstrates how to share business logic between Flutter client and Dart server using package:shelf
.
This sample shows how to create and customize cross-platform context menus, such as the text selection toolbar on mobile or the right click menu on desktop.
Demonstrates desktop features in both Material and FluentUI design systems.
Demonstrates how to call on-device Flutter APIs based on output from the Gemini API.
A sample demonstrating different types of forms and best practices.
A developer sample written in Flutter demonstrating how to interact with a to-do list in natural language using the Gemini API.
Demonstrates the Google Maps for Flutter plugin.
A Flutter sample app that shows an implementation of the 'infinite list' UX pattern.
A sample application that demonstrate best practices when using isolates.
A sample that shows how to use go_router
API to handle common navigation scenarios.
A sample place tracking app that uses the google_apps_flutter plugin.
This sample project shows a Flutter app that maximizes application code reuse while adhering to different design patterns on Android and iOS.
The starter Flutter application, but using the Provider package to manage state.
A Flutter sample app that shows a state management approach using the Provider package.
A simple Flutter fragment shaders project.
A calculator sample to demonstrate a simple start for a desktop Flutter app.
A sample app that shows different types of testing in Flutter.
Modifies the index.html of a flutter app so it is launched in a custom hostElement. This is the most basic embedding example.
A simple Angular app (and component) that replicates the above example, but in an Angular style.
A sample Flutter app which demonstrates how to use MethodChannel
, EventChannel
, BasicMessageChannel
and MessageCodec
.
A command line app that parses command-line options and fetches from GitHub.
Demonstrates Dart's extensions method syntax.
A series of simple examples demonstrating how to call C libraries from Dart.
Command line applications that demonstrate how to work with Concurrency in Dart using isolates.
A command line application that can be compiled to native code using dart compile exe
.
Examples of running Dart on the server.
Demonstrates best-practices for publishing packages on pub.dev.
Subject
Type
Unless stated otherwise, the documentation on this site reflects the latest stable version of Flutter. Page last updated on 2025-05-30. View source or report an issue.